Inline Web Workers: A Comprehensive Guide
While web workers are typically defined in separate JavaScript files, there is a method to create them inline within the same HTML file. This approach is advantageous when aiming to minimize the number of separate files for distribution, especially when using tools like the closure compiler for code optimization.
Creating Inline Web Workers
Inline web workers utilize Blob() to create a URL handle to the worker code as a string. This allows the worker to be included directly in the HTML file. Here's a complete example:
HTML with Inline Worker Code:
<code class="html"> <script id="worker1" type="javascript/worker"> // This script won't be parsed by JS engines due to its type. self.onmessage = function(e) { self.postMessage('msg from worker'); }; </script></code>
Main JavaScript Code:
<code class="javascript">var blob = new Blob([ document.querySelector('#worker1').textContent ], { type: "text/javascript" }); // Use window.webkitURL.createObjectURL() for Chrome versions below 11. var worker = new Worker(window.URL.createObjectURL(blob)); worker.onmessage = function(e) { console.log("Received: " + e.data); }; worker.postMessage("hello"); // Start the worker.</code>
In this example, the inline worker code is defined within the <script> tag with the type attribute set to "javascript/worker". The main JavaScript code then creates a Blob object from the worker code and uses window.URL.createObjectURL() to create a URL handle for the worker.</script>
Benefits and Use Cases
Inline web workers offer several benefits:
- Combining workers and main code into a single file for optimized distribution and reduced HTTP requests.
- Enhanced security as inline worker code cannot be accessed by external sites.
Some possible use cases for inline web workers include:
- Long-running computations that require offloading from the main thread.
- Handling time-sensitive tasks without interrupting the main thread.
- Isolating specific code for security or maintainability purposes.
The above is the detailed content of Inline Web Workers: When and How to Use Them?. For more information, please follow other related articles on the PHP Chinese website!
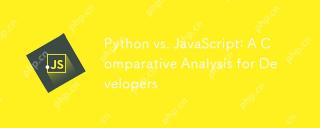
The main difference between Python and JavaScript is the type system and application scenarios. 1. Python uses dynamic types, suitable for scientific computing and data analysis. 2. JavaScript adopts weak types and is widely used in front-end and full-stack development. The two have their own advantages in asynchronous programming and performance optimization, and should be decided according to project requirements when choosing.
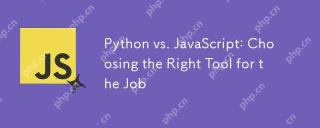
Whether to choose Python or JavaScript depends on the project type: 1) Choose Python for data science and automation tasks; 2) Choose JavaScript for front-end and full-stack development. Python is favored for its powerful library in data processing and automation, while JavaScript is indispensable for its advantages in web interaction and full-stack development.
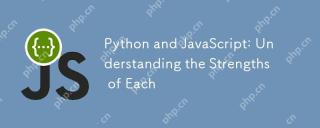
Python and JavaScript each have their own advantages, and the choice depends on project needs and personal preferences. 1. Python is easy to learn, with concise syntax, suitable for data science and back-end development, but has a slow execution speed. 2. JavaScript is everywhere in front-end development and has strong asynchronous programming capabilities. Node.js makes it suitable for full-stack development, but the syntax may be complex and error-prone.
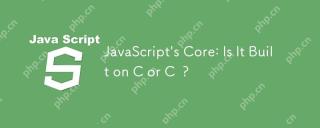
JavaScriptisnotbuiltonCorC ;it'saninterpretedlanguagethatrunsonenginesoftenwritteninC .1)JavaScriptwasdesignedasalightweight,interpretedlanguageforwebbrowsers.2)EnginesevolvedfromsimpleinterpreterstoJITcompilers,typicallyinC ,improvingperformance.
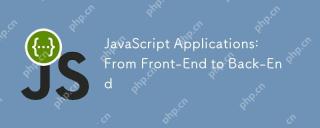
JavaScript can be used for front-end and back-end development. The front-end enhances the user experience through DOM operations, and the back-end handles server tasks through Node.js. 1. Front-end example: Change the content of the web page text. 2. Backend example: Create a Node.js server.
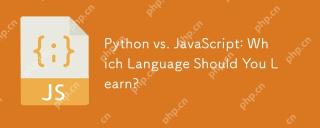
Choosing Python or JavaScript should be based on career development, learning curve and ecosystem: 1) Career development: Python is suitable for data science and back-end development, while JavaScript is suitable for front-end and full-stack development. 2) Learning curve: Python syntax is concise and suitable for beginners; JavaScript syntax is flexible. 3) Ecosystem: Python has rich scientific computing libraries, and JavaScript has a powerful front-end framework.
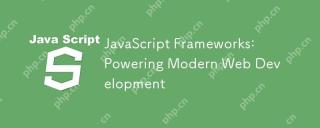
The power of the JavaScript framework lies in simplifying development, improving user experience and application performance. When choosing a framework, consider: 1. Project size and complexity, 2. Team experience, 3. Ecosystem and community support.
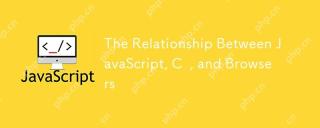
Introduction I know you may find it strange, what exactly does JavaScript, C and browser have to do? They seem to be unrelated, but in fact, they play a very important role in modern web development. Today we will discuss the close connection between these three. Through this article, you will learn how JavaScript runs in the browser, the role of C in the browser engine, and how they work together to drive rendering and interaction of web pages. We all know the relationship between JavaScript and browser. JavaScript is the core language of front-end development. It runs directly in the browser, making web pages vivid and interesting. Have you ever wondered why JavaScr


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

Atom editor mac version download
The most popular open source editor
