


Recovering Array Structures from var_dump Output
Introduction:
Often overlooked, there exists a challenge in PHP where one may encounter the need to reconstruct an array from its var_dump output. This seemingly straightforward task lacks a native solution in PHP and has sparked creativity among developers.
Problem Statement:
Given an array, regardless of its dimensionality, output its var_dump representation:
<code class="php">$data = array('this' => array('is' => 'the'), 'challenge' => array('for' => array('you'))); $var_dump_output = array(2) { ["this"]=> array(1) ["is"]=> string(3) "the" ["challenge"]=> array(1) ["for"]=> array(1) [0]=> string(3) "you" }</code>
The challenge is to devise an optimized method to convert this var_dump representation back into a usable PHP array.
Solution:
While serialization and var_export offer valid solutions, this challenge seeks creative and efficient approaches. One such approach involves converting the var_dump output into a serialized string and then unserializing it.
<code class="php">function unvar_dump($str) { // Add new lines for improved readability if (strpos($str, "\n") === false) { $str = preg_replace(['#(\[.*?\]=>)#', '#(string\(|int\(|float\(|array\(|NULL|object\(|})#'], "\n\1", $str); $str = trim($str); } // Regular expressions for pattern matching $regex = array( '#^\040*NULL\040*$#m', '#^\s*array\((.*?)\)\s*{\s*$#m', '#^\s*string\((.*?)\)\s*(.*?)$#m', '#^\s*int\((.*?)\)\s*$#m', '#^\s*bool\(true\)\s*$#m', '#^\s*bool\(false\)\s*$#m', '#^\s*float\((.*?)\)\s*$#m', '#^\s*\[(\d+)\]\s*=>\s*$#m', '#\s*?\r?\n\s*#m', ); $replace = array( 'N', 'a:\1:{', 's:\1:\2', 'i:\1', 'b:1', 'b:0', 'd:\1', 'i:\1', ';' ); // Convert to serialized string $serialized = preg_replace($regex, $replace, $str); // Handle string keys $func = create_function('$match', 'return "s:".strlen($match[1]).":\"".$match[1]."\"";'); $serialized = preg_replace_callback('#\s*\["(.*?)"\]\s*=>#', $func, $serialized); // Handle objects $func = create_function('$match', 'return "O:".strlen($match[1]).":\"".$match[1]."\":".$match[2].":{";'); $serialized = preg_replace_callback('#object\((.*?)\).*?\((\d+)\)\s*{\s*;#', $func, $serialized); // Finalize serialized string $serialized = preg_replace(['#};#', '#{;#'], ['}', '{'], $serialized); // Unserialize and return the array return unserialize($serialized); }</code>
Example Usage:
<code class="php">$complex_array = array( "foo" => "Foo\"bar\"", 0 => 4, 5 => 43.2, "af" => array( 0 => "123", 1 => (object)[ "bar" => "bart", "foo" => ["re"] ], 2 => NULL ) ); $var_dump_output = var_export($complex_array, true); $reconstructed_array = unvar_dump($var_dump_output); // Verify if the reconstructed array matches the original var_dump($complex_array === $reconstructed_array); // Outputs: bool(true)</code>
This solution provides a practical and optimized method for reconstructing arrays from var_dump output, showcasing alternative approaches to data manipulation in PHP.
The above is the detailed content of How can you effectively reconstruct a PHP array from its var_dump output, given the lack of a native solution in PHP?. For more information, please follow other related articles on the PHP Chinese website!
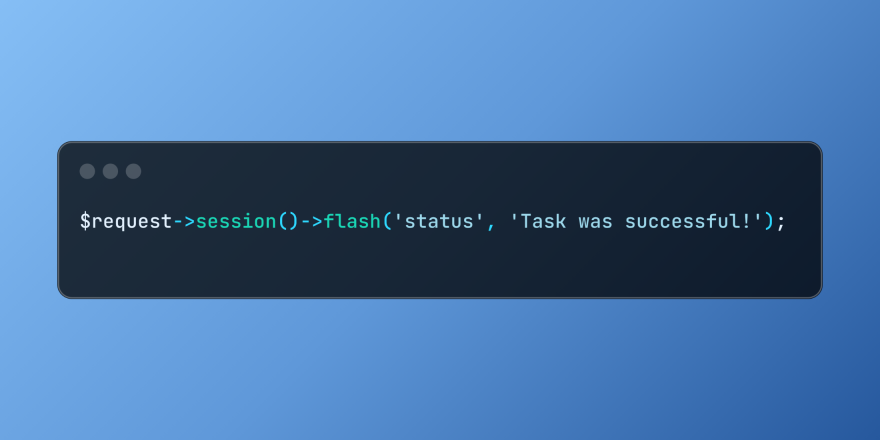
Laravel simplifies handling temporary session data using its intuitive flash methods. This is perfect for displaying brief messages, alerts, or notifications within your application. Data persists only for the subsequent request by default: $request-
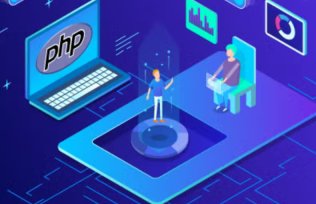
The PHP Client URL (cURL) extension is a powerful tool for developers, enabling seamless interaction with remote servers and REST APIs. By leveraging libcurl, a well-respected multi-protocol file transfer library, PHP cURL facilitates efficient execution of various network protocols, including HTTP, HTTPS, and FTP. This extension offers granular control over HTTP requests, supports multiple concurrent operations, and provides built-in security features.
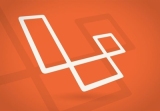
This is the second and final part of the series on building a React application with a Laravel back-end. In the first part of the series, we created a RESTful API using Laravel for a basic product-listing application. In this tutorial, we will be dev
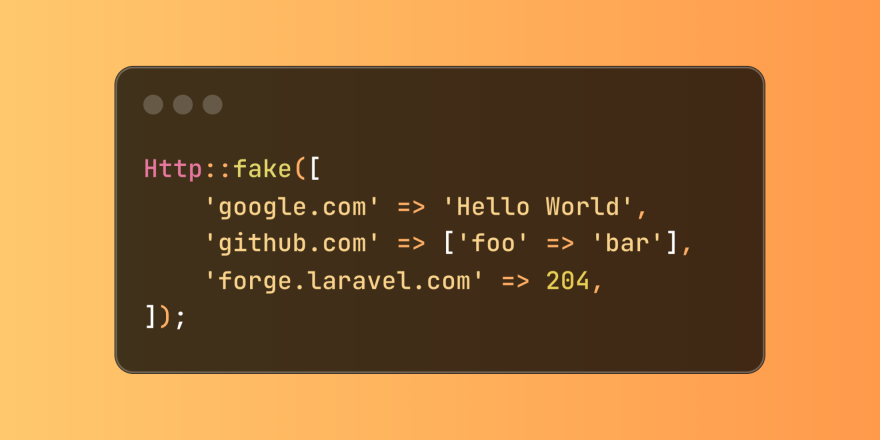
Laravel provides concise HTTP response simulation syntax, simplifying HTTP interaction testing. This approach significantly reduces code redundancy while making your test simulation more intuitive. The basic implementation provides a variety of response type shortcuts: use Illuminate\Support\Facades\Http; Http::fake([ 'google.com' => 'Hello World', 'github.com' => ['foo' => 'bar'], 'forge.laravel.com' =>
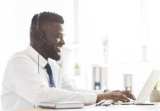
Do you want to provide real-time, instant solutions to your customers' most pressing problems? Live chat lets you have real-time conversations with customers and resolve their problems instantly. It allows you to provide faster service to your custom
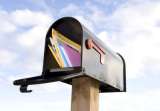
In this article, we're going to explore the notification system in the Laravel web framework. The notification system in Laravel allows you to send notifications to users over different channels. Today, we'll discuss how you can send notifications ov
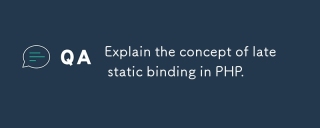
Article discusses late static binding (LSB) in PHP, introduced in PHP 5.3, allowing runtime resolution of static method calls for more flexible inheritance.Main issue: LSB vs. traditional polymorphism; LSB's practical applications and potential perfo
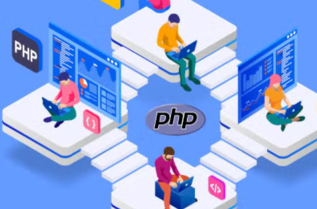
PHP logging is essential for monitoring and debugging web applications, as well as capturing critical events, errors, and runtime behavior. It provides valuable insights into system performance, helps identify issues, and supports faster troubleshoot


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
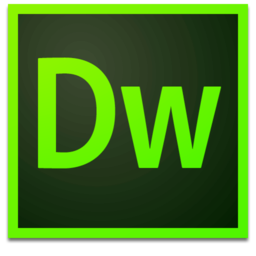
Dreamweaver Mac version
Visual web development tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
