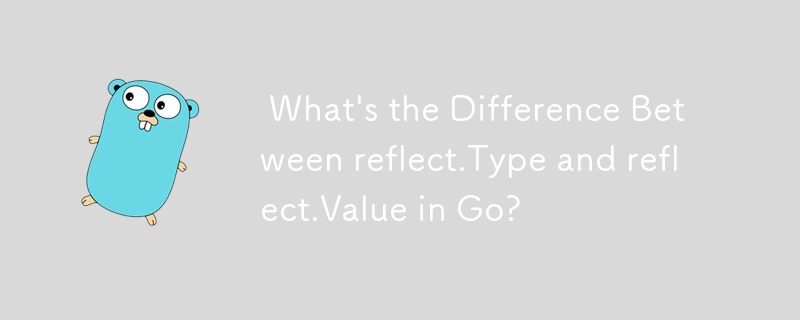
Reflection Type and Value in Go
Question:
In the following Go code snippet:
<code class="go">func show(i interface{}) {
switch t := i.(type) {
case *Person:
t := reflect.TypeOf(i) //what does t contain?
v := reflect.ValueOf(i) //what does v contain?
tag := t.Elem().Field(0).Tag
name := v.Elem().Field(0).String()
}
}</code>
What is the difference between the type and value in reflection?
Answer:
In Go, reflection provides two primary constructs: reflect.Type and reflect.Value. These types allow you to introspect and manipulate data at runtime.
-
reflect.Type: Represents the type information of a value, such as its name, underlying type, and struct fields. It allows you to query information that is tied to all variables with the same type.
-
reflect.Value: Represents an individual value and allows you to interact with its data. You can use it to set and retrieve values, convert between types, and perform other operations on the underlying data.
In the code snippet provided:
- t := reflect.TypeOf(i) returns a reflect.Type that represents the type of the value passed to the show function.
- v := reflect.ValueOf(i) returns a reflect.Value that represents the actual value passed to the function.
To access information about the type and value, you can use the following methods:
- t.Elem(): Returns the reflect.Type of the underlying element if the type is a pointer.
- t.Field(0).Tag: Returns the tag associated with the first field of the type.
- v.Elem(): Returns the reflect.Value of the underlying element if the value is a pointer.
- v.Field(0).String() : Converts the data in the first field of the value to a string.
The above is the detailed content of What\'s the Difference Between reflect.Type and reflect.Value in Go?. For more information, please follow other related articles on the PHP Chinese website!
Statement:The content of this article is voluntarily contributed by netizens, and the copyright belongs to the original author. This site does not assume corresponding legal responsibility. If you find any content suspected of plagiarism or infringement, please contact admin@php.cn