Are All Integer Values Perfectly Represented as Doubles?
The question arises as to whether all integer values are guaranteed to have a perfect representation as double-precision floating-point numbers. To answer this, we first delve into the representation of doubles.
Doubles are represented as mantissa * 2^exponent, where the mantissa includes fractional digits. This allows for the representation of both integer and decimal values.
Representation of 32-Bit Integers
For 32-bit integers, there are up to 53 bits available for the mantissa. This is sufficient to represent all possible 32-bit integers without fractional loss. Thus, yes, all 32-bit integer values are perfectly represented as doubles.
Representation of 64-Bit Integers
However, for 64-bit integers, the situation is different. IEEE 754 double-precision can guarantee perfect representation for up to 53 bits. Beyond that, there may be rounding errors. Therefore, no, not all 64-bit integer values are perfectly represented as doubles.
Empirically Verifying the Behavior
The following code snippet tests the conversion of integers to doubles:
<code class="cpp">#include <iostream> #include <limits> using namespace std; int main() { double test; volatile int test_int; for (int i = 0; i ::max(); i++) { test = i; test_int = test; if (test_int != i) cout <p>Running this code reveals that there are no integer values with fractional conversion errors for 32-bit integers. However, for 64-bit integers, it is possible to find integer values that convert to doubles with fractional differences and round back to the original integer value.</p> <p><strong>Fractional Differences</strong></p> <p>Regarding the possibility of fractional differences during conversion, the answer is still no for integers. This is because the step width between double values represented as mantissa * 2^exponent is always a power of two. Therefore, there is never a difference smaller than 2 between two double values, resolving any rounding issues.</p></limits></iostream></code>
The above is the detailed content of Can All Integer Values be Perfectly Represented as Doubles?. For more information, please follow other related articles on the PHP Chinese website!
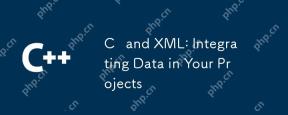
Integrating XML in a C project can be achieved through the following steps: 1) parse and generate XML files using pugixml or TinyXML library, 2) select DOM or SAX methods for parsing, 3) handle nested nodes and multi-level properties, 4) optimize performance using debugging techniques and best practices.
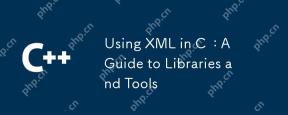
XML is used in C because it provides a convenient way to structure data, especially in configuration files, data storage and network communications. 1) Select the appropriate library, such as TinyXML, pugixml, RapidXML, and decide according to project needs. 2) Understand two ways of XML parsing and generation: DOM is suitable for frequent access and modification, and SAX is suitable for large files or streaming data. 3) When optimizing performance, TinyXML is suitable for small files, pugixml performs well in memory and speed, and RapidXML is excellent in processing large files.
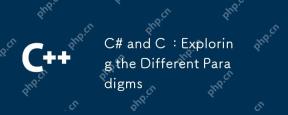
The main differences between C# and C are memory management, polymorphism implementation and performance optimization. 1) C# uses a garbage collector to automatically manage memory, while C needs to be managed manually. 2) C# realizes polymorphism through interfaces and virtual methods, and C uses virtual functions and pure virtual functions. 3) The performance optimization of C# depends on structure and parallel programming, while C is implemented through inline functions and multithreading.
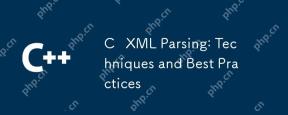
The DOM and SAX methods can be used to parse XML data in C. 1) DOM parsing loads XML into memory, suitable for small files, but may take up a lot of memory. 2) SAX parsing is event-driven and is suitable for large files, but cannot be accessed randomly. Choosing the right method and optimizing the code can improve efficiency.
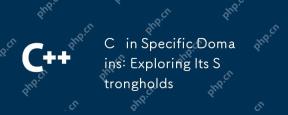
C is widely used in the fields of game development, embedded systems, financial transactions and scientific computing, due to its high performance and flexibility. 1) In game development, C is used for efficient graphics rendering and real-time computing. 2) In embedded systems, C's memory management and hardware control capabilities make it the first choice. 3) In the field of financial transactions, C's high performance meets the needs of real-time computing. 4) In scientific computing, C's efficient algorithm implementation and data processing capabilities are fully reflected.
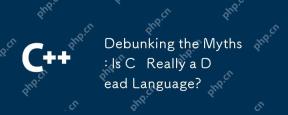
C is not dead, but has flourished in many key areas: 1) game development, 2) system programming, 3) high-performance computing, 4) browsers and network applications, C is still the mainstream choice, showing its strong vitality and application scenarios.
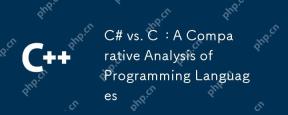
The main differences between C# and C are syntax, memory management and performance: 1) C# syntax is modern, supports lambda and LINQ, and C retains C features and supports templates. 2) C# automatically manages memory, C needs to be managed manually. 3) C performance is better than C#, but C# performance is also being optimized.
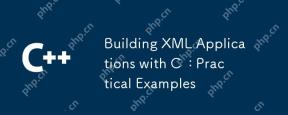
You can use the TinyXML, Pugixml, or libxml2 libraries to process XML data in C. 1) Parse XML files: Use DOM or SAX methods, DOM is suitable for small files, and SAX is suitable for large files. 2) Generate XML file: convert the data structure into XML format and write to the file. Through these steps, XML data can be effectively managed and manipulated.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
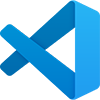
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

Notepad++7.3.1
Easy-to-use and free code editor
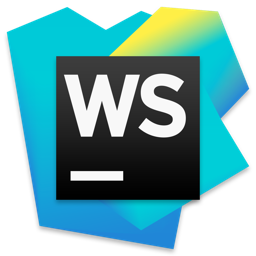
WebStorm Mac version
Useful JavaScript development tools

SublimeText3 Chinese version
Chinese version, very easy to use

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
