


The Value of this Within the Handler Using addEventListener
In JavaScript, this refers to the object on which the method is invoked. However, when handling events using addEventListener, this can refer to the element that raised the event rather than the object containing the event handler function.
Consider the following example:
<code class="javascript">function ticketTable(tickets) { this.tickets = tickets; } ticketTable.prototype.render = function (element) { var tbl = document.createElement("table"); for (var i = 0; i <p>In the handleCellClick function, this refers to the clicked cell, not the ticketTable object. This issue can be resolved using the bind method.</p> <p>bind allows you to specify the value of this for a function. In this case, you can bind the this value to the ticketTable object:</p> <pre class="brush:php;toolbar:false"><code class="javascript">cell1.addEventListener("click", this.handleCellClick.bind(this), false);</code>
The bound function will have the correct this context when the event is raised:
<code class="javascript">ticketTable.prototype.handleCellClick = function () { alert(this.innerHTML); // Still works fine alert(this.tickets.length); // Now works as expected };</code>
Alternatively, you can use the handleEvent method, which is specifically designed to handle events. In this case, this will always refer to the object implementing the method:
<code class="javascript">ticketTable.prototype.handleEvent = function (event) { console.log(this.name); // 'Something Good' switch (event.type) { case 'click': // Some code here... break; case 'dblclick': // Some code here... break; } };</code>
Both bind and handleEvent provide solutions to the problem of this reference in event handlers, allowing you to access the correct object context within your event handler functions.
The above is the detailed content of How Does the \'this\' Keyword Behave In JavaScript\'s `addEventListener` and How Can We Ensure Proper Context?. For more information, please follow other related articles on the PHP Chinese website!
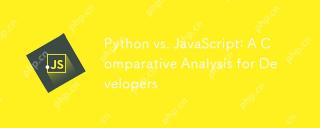
The main difference between Python and JavaScript is the type system and application scenarios. 1. Python uses dynamic types, suitable for scientific computing and data analysis. 2. JavaScript adopts weak types and is widely used in front-end and full-stack development. The two have their own advantages in asynchronous programming and performance optimization, and should be decided according to project requirements when choosing.
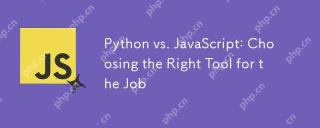
Whether to choose Python or JavaScript depends on the project type: 1) Choose Python for data science and automation tasks; 2) Choose JavaScript for front-end and full-stack development. Python is favored for its powerful library in data processing and automation, while JavaScript is indispensable for its advantages in web interaction and full-stack development.
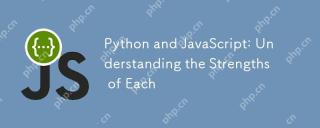
Python and JavaScript each have their own advantages, and the choice depends on project needs and personal preferences. 1. Python is easy to learn, with concise syntax, suitable for data science and back-end development, but has a slow execution speed. 2. JavaScript is everywhere in front-end development and has strong asynchronous programming capabilities. Node.js makes it suitable for full-stack development, but the syntax may be complex and error-prone.
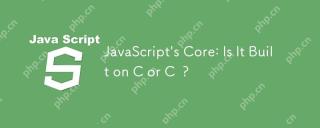
JavaScriptisnotbuiltonCorC ;it'saninterpretedlanguagethatrunsonenginesoftenwritteninC .1)JavaScriptwasdesignedasalightweight,interpretedlanguageforwebbrowsers.2)EnginesevolvedfromsimpleinterpreterstoJITcompilers,typicallyinC ,improvingperformance.
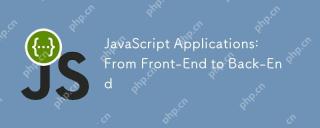
JavaScript can be used for front-end and back-end development. The front-end enhances the user experience through DOM operations, and the back-end handles server tasks through Node.js. 1. Front-end example: Change the content of the web page text. 2. Backend example: Create a Node.js server.
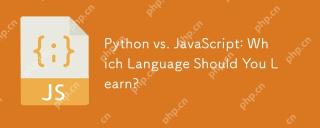
Choosing Python or JavaScript should be based on career development, learning curve and ecosystem: 1) Career development: Python is suitable for data science and back-end development, while JavaScript is suitable for front-end and full-stack development. 2) Learning curve: Python syntax is concise and suitable for beginners; JavaScript syntax is flexible. 3) Ecosystem: Python has rich scientific computing libraries, and JavaScript has a powerful front-end framework.
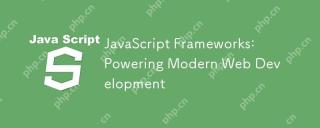
The power of the JavaScript framework lies in simplifying development, improving user experience and application performance. When choosing a framework, consider: 1. Project size and complexity, 2. Team experience, 3. Ecosystem and community support.
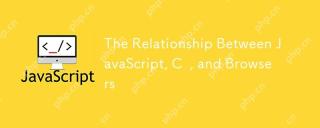
Introduction I know you may find it strange, what exactly does JavaScript, C and browser have to do? They seem to be unrelated, but in fact, they play a very important role in modern web development. Today we will discuss the close connection between these three. Through this article, you will learn how JavaScript runs in the browser, the role of C in the browser engine, and how they work together to drive rendering and interaction of web pages. We all know the relationship between JavaScript and browser. JavaScript is the core language of front-end development. It runs directly in the browser, making web pages vivid and interesting. Have you ever wondered why JavaScr


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
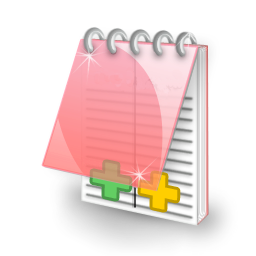
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
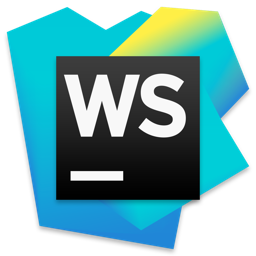
WebStorm Mac version
Useful JavaScript development tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

Zend Studio 13.0.1
Powerful PHP integrated development environment
