


How Can I Use PDO Prepared Statements with Wildcards for Efficient SQL Queries?
Executing SQL Queries with Wildcards Using PDO Prepared Statements
Performing pattern matching queries in database applications is crucial for flexible searches and data retrieval. This article addresses how to effectively use wildcards in conjunction with PDO prepared statements in PHP when executing MySQL queries.
Challenge: Using Wildcards with Prepared Statements
When utilizing prepared statements, which provide improved security and prevent SQL injection vulnerabilities, finding a solution to incorporate wildcards (% and _) for pattern matching can be challenging. This article will explore the successful implementation of wildcards with PDO prepared statements.
Solution: bindValue and bindParam
The key to successfully utilizing wildcards in prepared statements lies in selecting the appropriate binding method. The following two binding options are available:
- bindValue() Method: This method allows direct assignment of values to parameters. For wildcard usage, the wildcard characters must be included as part of the value being bound.
- bindParam() Method: This method binds a variable to a parameter. In this case, the wildcard characters can be assigned to the variable before being passed to the bindParam() method.
Usage Example: bindValue()
The following code snippet demonstrates the successful execution of a query using bindValue():
<code class="php">$stmt = $dbh->prepare("SELECT * FROM `gc_users` WHERE `name` LIKE :name"); $stmt->bindValue(':name', '%' . $name . '%'); $stmt->execute();</code>
Usage Example: bindParam()
Alternatively, the bindParam() method can be employed as shown below:
<code class="php">$name = "%$name%"; $query = $dbh->prepare("SELECT * FROM `gc_users` WHERE `name` like :name"); $query->bindParam(':name', $name); $query->execute();</code>
By employing these techniques, it is possible to effectively perform pattern matching queries using prepared statements while maintaining security and preventing SQL injection vulnerabilities.
The above is the detailed content of How Can I Use PDO Prepared Statements with Wildcards for Efficient SQL Queries?. For more information, please follow other related articles on the PHP Chinese website!
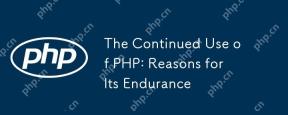
What’s still popular is the ease of use, flexibility and a strong ecosystem. 1) Ease of use and simple syntax make it the first choice for beginners. 2) Closely integrated with web development, excellent interaction with HTTP requests and database. 3) The huge ecosystem provides a wealth of tools and libraries. 4) Active community and open source nature adapts them to new needs and technology trends.
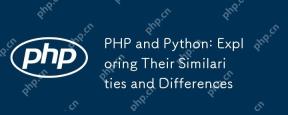
PHP and Python are both high-level programming languages that are widely used in web development, data processing and automation tasks. 1.PHP is often used to build dynamic websites and content management systems, while Python is often used to build web frameworks and data science. 2.PHP uses echo to output content, Python uses print. 3. Both support object-oriented programming, but the syntax and keywords are different. 4. PHP supports weak type conversion, while Python is more stringent. 5. PHP performance optimization includes using OPcache and asynchronous programming, while Python uses cProfile and asynchronous programming.
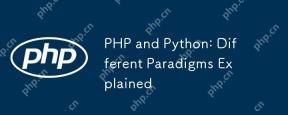
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
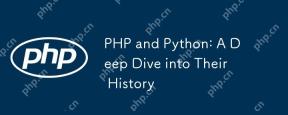
PHP originated in 1994 and was developed by RasmusLerdorf. It was originally used to track website visitors and gradually evolved into a server-side scripting language and was widely used in web development. Python was developed by Guidovan Rossum in the late 1980s and was first released in 1991. It emphasizes code readability and simplicity, and is suitable for scientific computing, data analysis and other fields.
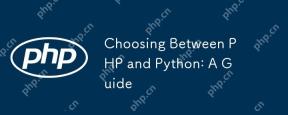
PHP is suitable for web development and rapid prototyping, and Python is suitable for data science and machine learning. 1.PHP is used for dynamic web development, with simple syntax and suitable for rapid development. 2. Python has concise syntax, is suitable for multiple fields, and has a strong library ecosystem.
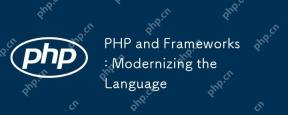
PHP remains important in the modernization process because it supports a large number of websites and applications and adapts to development needs through frameworks. 1.PHP7 improves performance and introduces new features. 2. Modern frameworks such as Laravel, Symfony and CodeIgniter simplify development and improve code quality. 3. Performance optimization and best practices further improve application efficiency.
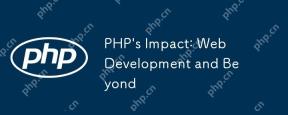
PHPhassignificantlyimpactedwebdevelopmentandextendsbeyondit.1)ItpowersmajorplatformslikeWordPressandexcelsindatabaseinteractions.2)PHP'sadaptabilityallowsittoscaleforlargeapplicationsusingframeworkslikeLaravel.3)Beyondweb,PHPisusedincommand-linescrip
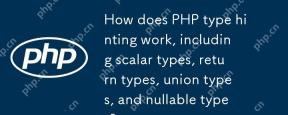
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
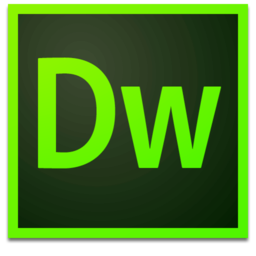
Dreamweaver Mac version
Visual web development tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SublimeText3 Chinese version
Chinese version, very easy to use
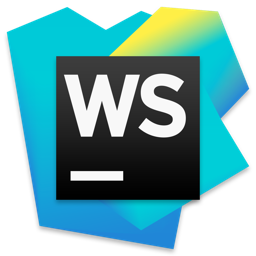
WebStorm Mac version
Useful JavaScript development tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.