


The Enigma of "std::shared_ptr of this"
In the realm of smart pointers, programmers often find themselves grappling with the intricate web of object ownership and lifetime management. One such challenge arises when attempting to pass a std::shared_ptr of the current object to its child.
Consider the following scenario: an object of class A holds a list of children of class B, while each child needs to maintain a reference to its parent. This seemingly straightforward task presents a roadblock when we attempt to create a std::shared_ptr pointing to the parent object.
<code class="cpp">class A { private: std::list<:shared_ptr>> children; }; class B { public: void setParent(std::shared_ptr<a> parent) { ... } };</a></:shared_ptr></code>
How do we pass a std::shared_ptr of the current A object to its children?
The Answer: std::enable_shared_from_this
The solution lies within the std::enable_shared_from_this template class. By inheriting from it, an object gains the ability to create a shared_ptr to itself through the shared_from_this method.
To resolve our dilemma, we modify our A class accordingly:
<code class="cpp">class A : public std::enable_shared_from_this<a> { // ... void addChild(std::shared_ptr<b> child) { children.push_back(child); child->setParent(shared_from_this()); } };</b></a></code>
Now, we can effortlessly pass a shared_ptr of the parent object to its children.
A Caveat: Circular Dependencies and Resource Leaks
However, our solution introduces a potential issue: circular dependencies. By holding references to both the parent and children, we create a cycle where each object relies on the existence of the other. This can lead to resource leaks if the objects are not properly released.
To mitigate this problem, we employ std::weak_ptr for the children's references to their parent. Weak pointers do not prevent the referenced object from being deleted and are automatically nullified upon deletion.
<code class="cpp">class A { private: std::list<:weak_ptr>> children; };</:weak_ptr></code>
With this adjustment, the circular dependency is broken, ensuring proper object destruction and preventing memory leaks.
Limitations: Ownership and Timing
It's important to note that calling shared_from_this() requires the current object to be owned by std::shared_ptr at the time of the call. This implies that the object cannot be created on the stack or called within a constructor or destructor.
The above is the detailed content of How can you create a `std::shared_ptr` of the current object (this) to pass to child objects when using `std::shared_ptr` for ownership?. For more information, please follow other related articles on the PHP Chinese website!
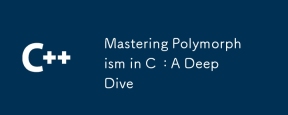
Mastering polymorphisms in C can significantly improve code flexibility and maintainability. 1) Polymorphism allows different types of objects to be treated as objects of the same base type. 2) Implement runtime polymorphism through inheritance and virtual functions. 3) Polymorphism supports code extension without modifying existing classes. 4) Using CRTP to implement compile-time polymorphism can improve performance. 5) Smart pointers help resource management. 6) The base class should have a virtual destructor. 7) Performance optimization requires code analysis first.
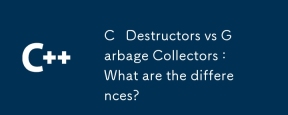
C destructorsprovideprecisecontroloverresourcemanagement,whilegarbagecollectorsautomatememorymanagementbutintroduceunpredictability.C destructors:1)Allowcustomcleanupactionswhenobjectsaredestroyed,2)Releaseresourcesimmediatelywhenobjectsgooutofscop
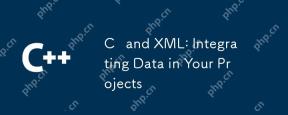
Integrating XML in a C project can be achieved through the following steps: 1) parse and generate XML files using pugixml or TinyXML library, 2) select DOM or SAX methods for parsing, 3) handle nested nodes and multi-level properties, 4) optimize performance using debugging techniques and best practices.
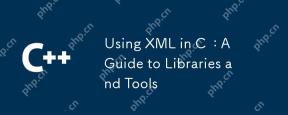
XML is used in C because it provides a convenient way to structure data, especially in configuration files, data storage and network communications. 1) Select the appropriate library, such as TinyXML, pugixml, RapidXML, and decide according to project needs. 2) Understand two ways of XML parsing and generation: DOM is suitable for frequent access and modification, and SAX is suitable for large files or streaming data. 3) When optimizing performance, TinyXML is suitable for small files, pugixml performs well in memory and speed, and RapidXML is excellent in processing large files.
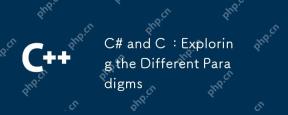
The main differences between C# and C are memory management, polymorphism implementation and performance optimization. 1) C# uses a garbage collector to automatically manage memory, while C needs to be managed manually. 2) C# realizes polymorphism through interfaces and virtual methods, and C uses virtual functions and pure virtual functions. 3) The performance optimization of C# depends on structure and parallel programming, while C is implemented through inline functions and multithreading.
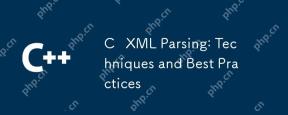
The DOM and SAX methods can be used to parse XML data in C. 1) DOM parsing loads XML into memory, suitable for small files, but may take up a lot of memory. 2) SAX parsing is event-driven and is suitable for large files, but cannot be accessed randomly. Choosing the right method and optimizing the code can improve efficiency.
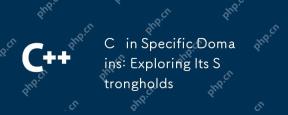
C is widely used in the fields of game development, embedded systems, financial transactions and scientific computing, due to its high performance and flexibility. 1) In game development, C is used for efficient graphics rendering and real-time computing. 2) In embedded systems, C's memory management and hardware control capabilities make it the first choice. 3) In the field of financial transactions, C's high performance meets the needs of real-time computing. 4) In scientific computing, C's efficient algorithm implementation and data processing capabilities are fully reflected.
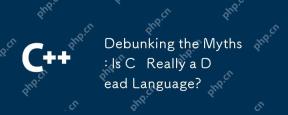
C is not dead, but has flourished in many key areas: 1) game development, 2) system programming, 3) high-performance computing, 4) browsers and network applications, C is still the mainstream choice, showing its strong vitality and application scenarios.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
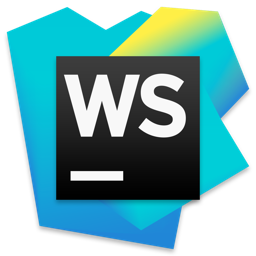
WebStorm Mac version
Useful JavaScript development tools
