Case-Insensitive Sorting Using sort.Strings() in Go
In Go, the sort.Strings() function is used to sort a list of strings. However, it doesn't provide an option for case-insensitive sorting out of the box.
Custom Comparison Function
One way to achieve case-insensitive sorting is to pass a custom comparison function to sort.Strings(). This function should return true if the first string should come before the second string in the sorted order.
The following code demonstrates how to do this:
<code class="go">package main import ( "fmt" "sort" ) func main() { data := []string{"A", "b", "D", "c"} sort.Slice(data, func(i, j int) bool { return strings.ToLower(data[i]) <p>This approach creates a new string for each comparison, which can be inefficient for large lists of strings.</p> <p><strong>Rune-by-Rune Comparison</strong></p> <p>To avoid allocations, a more efficient approach is to compare the strings rune by rune, converting them to lowercase on the fly:</p> <pre class="brush:php;toolbar:false"><code class="go">sort.Slice(data, func(i, j int) bool { for { rb, nb := utf8.DecodeRuneInString(data[j]) if nb == 0 { return false } ra, na := utf8.DecodeRuneInString(data[i]) if na == 0 { return true } rb = unicode.ToLower(rb) ra = unicode.ToLower(ra) if ra != rb { return ra <p><strong>Language-Specific Sorting</strong></p> <p>The collate package in Go provides more advanced functions for language-specific or culture-specific sorting orders.</p></code>
The above is the detailed content of How to Sort Strings Case-Insensitively in Go?. For more information, please follow other related articles on the PHP Chinese website!
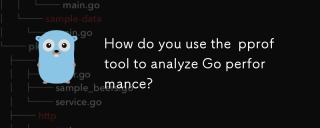
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
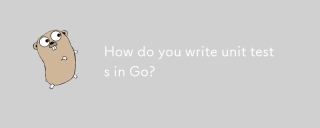
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
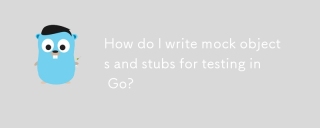
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
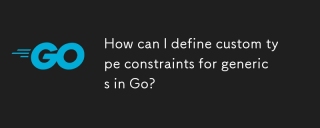
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
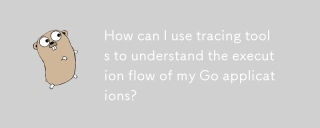
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization
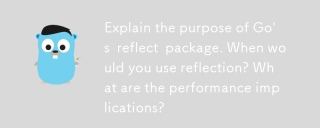
The article discusses Go's reflect package, used for runtime manipulation of code, beneficial for serialization, generic programming, and more. It warns of performance costs like slower execution and higher memory use, advising judicious use and best
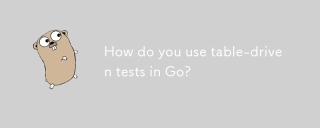
The article discusses using table-driven tests in Go, a method that uses a table of test cases to test functions with multiple inputs and outcomes. It highlights benefits like improved readability, reduced duplication, scalability, consistency, and a
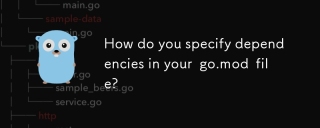
The article discusses managing Go module dependencies via go.mod, covering specification, updates, and conflict resolution. It emphasizes best practices like semantic versioning and regular updates.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

SublimeText3 Chinese version
Chinese version, very easy to use
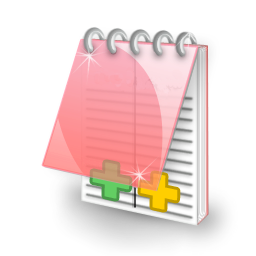
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
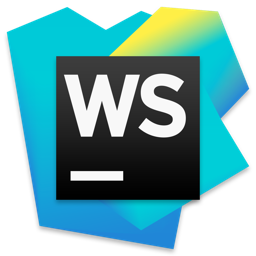
WebStorm Mac version
Useful JavaScript development tools

Notepad++7.3.1
Easy-to-use and free code editor
