


How do I efficiently switch between different panels in a Java GUI using a CardLayout?
Switching JPanels with a CardLayout
When working with component hierarchies in Java, a common task is transitioning between different graphical panels within a single frame. In the specific case of switching between a menu and a game panel, a common misconception is attempting to add and remove components dynamically.
To effectively switch between panels, consider utilizing a CardLayout, which is designed for this purpose. Here's how it works:
- Create a CardLayout: Instantiate a CardLayout object, which will manage the transitions between panels.
- Create a Main Panel: Create a JPanel to serve as the container for your panels and set its layout manager to the CardLayout.
- Add Panels to the Main Panel: Add the MenuPanel and GamePanel to the main panel, assigning them unique names (e.g., "menu" and "game") within the add() method.
- Trigger Transitions: Later in your code, when you need to switch panels, simply call the cardLayout.show(mainPanel, "desiredPanelName") method. This will push the current panel to the back and bring the desired panel to the front.
Implementation Example:
Consider the following example code that uses a CardLayout to switch between a menu panel and a game panel:
<code class="java">import java.awt.*; import java.awt.event.*; import javax.swing.*; public class GameFrame extends JFrame implements ActionListener { private JPanel mainPanel; private CardLayout cardLayout; private MenuPanel menuPanel; private GamePanel gamePanel; public GameFrame() { mainPanel = new JPanel(cardLayout = new CardLayout()); menuPanel = new MenuPanel(); gamePanel = new GamePanel(); mainPanel.add(menuPanel, "menu"); mainPanel.add(gamePanel, "game"); JButton goGameButton = new JButton("Go to Game"); goGameButton.addActionListener(this); add(mainPanel); add(goGameButton, BorderLayout.SOUTH); setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); pack(); setVisible(true); } @Override public void actionPerformed(ActionEvent e) { cardLayout.show(mainPanel, "game"); } public static void main(String[] args) { SwingUtilities.invokeLater(() -> new GameFrame()); } } class MenuPanel extends JPanel { public MenuPanel() { setBackground(Color.GREEN); add(new JLabel("Menu")); } @Override public Dimension getPreferredSize() { return new Dimension(300, 300); } } class GamePanel extends JPanel { public GamePanel() { setBackground(Color.BLUE); add(new JLabel("Game")); } @Override public Dimension getPreferredSize() { return new Dimension(300, 300); } }</code>
By using a CardLayout, you can seamlessly switch between panels without the need to remove and re-add components, which simplifies code and avoids potential performance issues.
The above is the detailed content of How do I efficiently switch between different panels in a Java GUI using a CardLayout?. For more information, please follow other related articles on the PHP Chinese website!
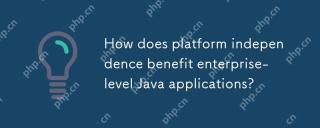
Java is widely used in enterprise-level applications because of its platform independence. 1) Platform independence is implemented through Java virtual machine (JVM), so that the code can run on any platform that supports Java. 2) It simplifies cross-platform deployment and development processes, providing greater flexibility and scalability. 3) However, it is necessary to pay attention to performance differences and third-party library compatibility and adopt best practices such as using pure Java code and cross-platform testing.
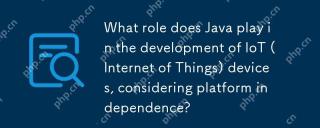
JavaplaysasignificantroleinIoTduetoitsplatformindependence.1)Itallowscodetobewrittenonceandrunonvariousdevices.2)Java'secosystemprovidesusefullibrariesforIoT.3)ItssecurityfeaturesenhanceIoTsystemsafety.However,developersmustaddressmemoryandstartuptim
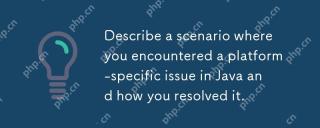
ThesolutiontohandlefilepathsacrossWindowsandLinuxinJavaistousePaths.get()fromthejava.nio.filepackage.1)UsePaths.get()withSystem.getProperty("user.dir")andtherelativepathtoconstructthefilepath.2)ConverttheresultingPathobjecttoaFileobjectifne
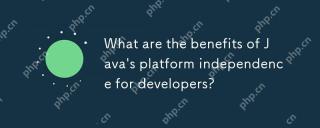
Java'splatformindependenceissignificantbecauseitallowsdeveloperstowritecodeonceandrunitonanyplatformwithaJVM.This"writeonce,runanywhere"(WORA)approachoffers:1)Cross-platformcompatibility,enablingdeploymentacrossdifferentOSwithoutissues;2)Re
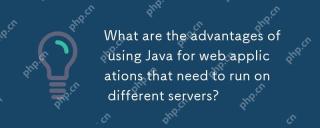
Java is suitable for developing cross-server web applications. 1) Java's "write once, run everywhere" philosophy makes its code run on any platform that supports JVM. 2) Java has a rich ecosystem, including tools such as Spring and Hibernate, to simplify the development process. 3) Java performs excellently in performance and security, providing efficient memory management and strong security guarantees.
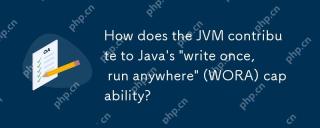
JVM implements the WORA features of Java through bytecode interpretation, platform-independent APIs and dynamic class loading: 1. Bytecode is interpreted as machine code to ensure cross-platform operation; 2. Standard API abstract operating system differences; 3. Classes are loaded dynamically at runtime to ensure consistency.
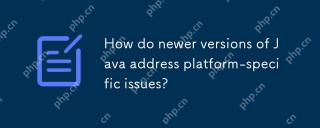
The latest version of Java effectively solves platform-specific problems through JVM optimization, standard library improvements and third-party library support. 1) JVM optimization, such as Java11's ZGC improves garbage collection performance. 2) Standard library improvements, such as Java9's module system reducing platform-related problems. 3) Third-party libraries provide platform-optimized versions, such as OpenCV.
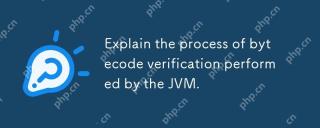
The JVM's bytecode verification process includes four key steps: 1) Check whether the class file format complies with the specifications, 2) Verify the validity and correctness of the bytecode instructions, 3) Perform data flow analysis to ensure type safety, and 4) Balancing the thoroughness and performance of verification. Through these steps, the JVM ensures that only secure, correct bytecode is executed, thereby protecting the integrity and security of the program.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
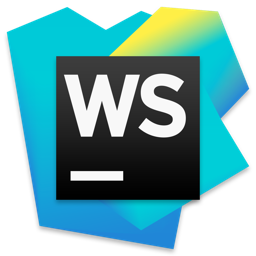
WebStorm Mac version
Useful JavaScript development tools

SublimeText3 English version
Recommended: Win version, supports code prompts!
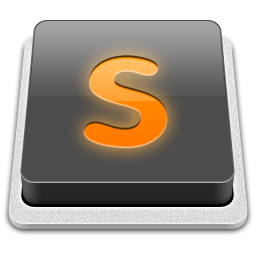
SublimeText3 Mac version
God-level code editing software (SublimeText3)

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
