


Posting to an API with Content-Type: multipart/form-data
When attempting to POST to an API requiring Content-Type: multipart/form-data, you may encounter issues if you are using []byte parameters and string arguments. The provided error message indicates a redirection issue, which is unrelated to the issue at hand.
The solution lies in constructing the multipart/form-data request body using the multipart package. Here's an example:
<code class="go">package main import ( "bytes" "fmt" "io" "io/ioutil" "mime/multipart" "net/http" "github.com/ganshane/typeregistry" ) type FileItem struct { Key string //image_content FileName string //test.jpg Content []byte //[]byte } func NewPostFile(url string, paramTexts map[string]interface{}, paramFile FileItem) ([]byte, error) { // Construct the multipart request body bodyBuf := &bytes.Buffer{} bodyWriter := multipart.NewWriter(bodyBuf) for k, v := range paramTexts { bodyWriter.WriteField(k, v.(string)) } fileWriter, err := bodyWriter.CreateFormFile(paramFile.Key, paramFile.FileName) if err != nil { fmt.Println(err) //fmt.Println("Create form file error: ", error) return nil, err } fileWriter.Write(paramFile.Content) contentType := bodyWriter.FormDataContentType() bodyWriter.Close() fmt.Println(bodyBuf.String()) // Perform the POST request resp, err := http.Post(url, contentType, bodyBuf) if err != nil { return nil, err } defer resp.Body.Close() fmt.Println(resp) // Handle the response if resp.StatusCode = 300 { b, _ := ioutil.ReadAll(resp.Body) return nil, fmt.Errorf("[%d %s]%s", resp.StatusCode, resp.Status, string(b)) } respData, err := ioutil.ReadAll(resp.Body) if err != nil { return nil, err } fmt.Println(string(respData)) return respData, nil } func main() { m := make(map[string]interface{}, 0) m["fileName"] = "good" m["name"] = typeregistry.Base64ToByte("/9j/4AAQSkZJRgABAQEAeAB4AAD/2wBDAAIBAQIBAQICAgICAgICAwUDAwMDAwYEBAMFBwYHBwcGBwcICQsJCAgKCAcHCg0KCgsMDAwMBwkODw0MDgsMDAz/2wBDAQICAgMDAwYDAwYMCAcIDAwMDAwMDAwMDAwMDAwMDAwMDAwMDAwMDAwMDAwMDAwMDAwMDAwMDAwMDAwMDAwMDAz/wAARCAABAAEDASIAAhEBAxEB/8QAHwAAAQUBAQEBAQEAAAAAAAAAAAECAwQFBgcICQoL/8QAtRAAAgEDAwIEAwUFBAQAAAF9AQIDAAQRBRIhMUEGE1FhByJxFDKBkaEII0KxwRVS0fAkM2JyggkKFhcYGRolJicoKSo0NTY3ODk6Q0RFRkdISUpTVFVWV1hZWmNkZWZnaGlqc3R1dnd4eXqDhIWGh4iJipKTlJWWl5iZmqKjpKWmp6ipqrKztLW2t7i5usLDxMXGx8jJytLT1NXW19jZ2uHi4+Tl5ufo6erx8vP09fb3+Pn6/8QAHwEAAwEBAQEBAQEBAQAAAAAAAAECAwQFBgcICQoL/8QAtREAAgECBAQDBAcFBAQAAQJ3AAECAxEEBSExBhJBUQdhcRMiMoEIFEKRobHBCSMzUvAVYnLRChYkNOEl8RcYGRomJygpKjU2Nzg5OkNERUZHSElKU1RVVldYWVpjZGVmZ2hpanN0dXZ3eHl6goOEhYaHiImKkpOUlZaXmJmaoqOkpaanqKmqsrO0tba3uLm6wsPExcbHyMnK0tPU1dbX2Nna4uPk5ebn6Onq8vP09fb3+Pn6/9oADAMBAAIRAxEAPwDHooor+wD+Zz//2Q==") paramFile := FileItem{ Key: "image_content", FileName: "test.jpg", Content: m["name"].([]byte), } respData, err := NewPostFile("API_URL", m, paramFile) if err != nil { // Handle error } fmt.Println(string(respData)) } </code>
In this example, we used the NewPostFile function to construct a POST request with multipart/form-data, including both regular form fields and a file. The function takes the URL, a map of string arguments, and a file item as input.
The response from the API can be retrieved from the respData variable and processed as needed. The error handling and response handling code is left to the developer to implement according to their specific requirements.
This solution should address the issue you were experiencing with POSTing to the API using Content-Type: multipart/form-data with []byte parameters and string arguments.
The above is the detailed content of How to Post to an API with Content-Type: multipart/form-data Using []byte Parameters and String Arguments?. For more information, please follow other related articles on the PHP Chinese website!
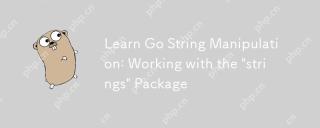
Go's "strings" package provides rich features to make string operation efficient and simple. 1) Use strings.Contains() to check substrings. 2) strings.Split() can be used to parse data, but it should be used with caution to avoid performance problems. 3) strings.Join() is suitable for formatting strings, but for small datasets, looping = is more efficient. 4) For large strings, it is more efficient to build strings using strings.Builder.
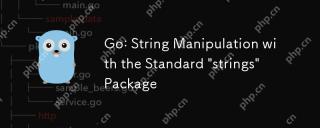
Go uses the "strings" package for string operations. 1) Use strings.Join function to splice strings. 2) Use the strings.Contains function to find substrings. 3) Use the strings.Replace function to replace strings. These functions are efficient and easy to use and are suitable for various string processing tasks.
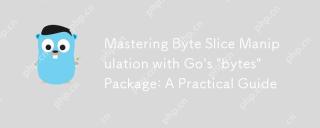
ThebytespackageinGoisessentialforefficientbyteslicemanipulation,offeringfunctionslikeContains,Index,andReplaceforsearchingandmodifyingbinarydata.Itenhancesperformanceandcodereadability,makingitavitaltoolforhandlingbinarydata,networkprotocols,andfileI
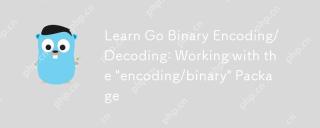
Go uses the "encoding/binary" package for binary encoding and decoding. 1) This package provides binary.Write and binary.Read functions for writing and reading data. 2) Pay attention to choosing the correct endian (such as BigEndian or LittleEndian). 3) Data alignment and error handling are also key to ensure the correctness and performance of the data.
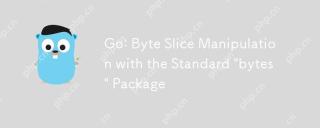
The"bytes"packageinGooffersefficientfunctionsformanipulatingbyteslices.1)Usebytes.Joinforconcatenatingslices,2)bytes.Bufferforincrementalwriting,3)bytes.Indexorbytes.IndexByteforsearching,4)bytes.Readerforreadinginchunks,and5)bytes.SplitNor
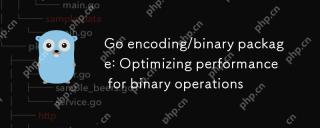
Theencoding/binarypackageinGoiseffectiveforoptimizingbinaryoperationsduetoitssupportforendiannessandefficientdatahandling.Toenhanceperformance:1)Usebinary.NativeEndianfornativeendiannesstoavoidbyteswapping.2)BatchReadandWriteoperationstoreduceI/Oover
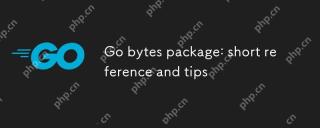
Go's bytes package is mainly used to efficiently process byte slices. 1) Using bytes.Buffer can efficiently perform string splicing to avoid unnecessary memory allocation. 2) The bytes.Equal function is used to quickly compare byte slices. 3) The bytes.Index, bytes.Split and bytes.ReplaceAll functions can be used to search and manipulate byte slices, but performance issues need to be paid attention to.
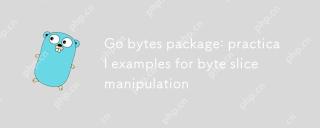
The byte package provides a variety of functions to efficiently process byte slices. 1) Use bytes.Contains to check the byte sequence. 2) Use bytes.Split to split byte slices. 3) Replace the byte sequence bytes.Replace. 4) Use bytes.Join to connect multiple byte slices. 5) Use bytes.Buffer to build data. 6) Combined bytes.Map for error processing and data verification.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

SublimeText3 Linux new version
SublimeText3 Linux latest version

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SublimeText3 English version
Recommended: Win version, supports code prompts!
