How to Utilize COM (Component Object Model) in Golang
Introduction
COM, short for Component Object Model, is a powerful library that enables developers to create reusable software components. In this question, we explore how to harness COM functionality within the Golang programming language.
Problem
The user seeks assistance in using a Windows DLL (XA_Session.dll) in their Golang code, specifically aiming to invoke the ConnectServer COM method. However, they encounter a compilation error.
Solution
To successfully access COM methods from Go, we need to convert them to uintptrs and pass them as arguments to the proc.Call function. The following code snippet demonstrates this approach:
<code class="go">package main import ( "syscall" "unsafe" ) var ( xaSession = syscall.NewLazyDLL("XA_Session.dll") getClassObject = xaSession.NewProc("DllGetClassObject") ) func main() { // Set the CLSID and IID to appropriate values. var rclsid, riid, ppv uintptr // Call DllGetClassObject to obtain the COM object. ret, _, _ := getClassObject.Call(rclsid, riid, ppv) if ret != 0 { // Handle error. } // Cast ppv to *XASession, assuming this type defines the wrapper methods. xasession := (*XASession)(unsafe.Pointer(ppv)) // Invoke the ConnectServer method. result := xasession.ConnectServer(20001) if result != 0 { // Handle error. } else { // Success. } }</code>
In this code, we obtain the COM object through DllGetClassObject and proceed to invoke the ConnectServer method using our wrapper functions.
Implementation Details
COM objects support QueryInterface, AddRef, and Release functions. Wrapper types like XASession can wrap such objects, providing access to additional methods.
The proc.Call function expects uintptr arguments, and we must set the parameters to appropriate values, such as specifying CLSID and IID.
Conclusion
By following the steps outlined in this solution, developers can harness the power of COM in their Golang applications. This allows them to leverage pre-built DLLs and libraries, enhancing their code's functionality and reusability.
The above is the detailed content of How to Call COM Methods in Go Using uintptrs and proc.Call?. For more information, please follow other related articles on the PHP Chinese website!
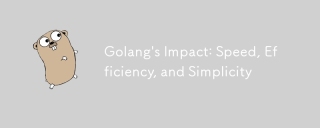
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
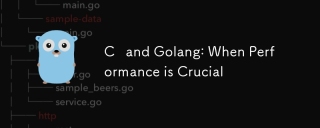
C is more suitable for scenarios where direct control of hardware resources and high performance optimization is required, while Golang is more suitable for scenarios where rapid development and high concurrency processing are required. 1.C's advantage lies in its close to hardware characteristics and high optimization capabilities, which are suitable for high-performance needs such as game development. 2.Golang's advantage lies in its concise syntax and natural concurrency support, which is suitable for high concurrency service development.
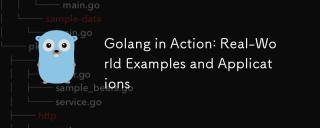
Golang excels in practical applications and is known for its simplicity, efficiency and concurrency. 1) Concurrent programming is implemented through Goroutines and Channels, 2) Flexible code is written using interfaces and polymorphisms, 3) Simplify network programming with net/http packages, 4) Build efficient concurrent crawlers, 5) Debugging and optimizing through tools and best practices.
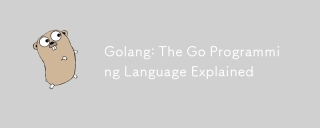
The core features of Go include garbage collection, static linking and concurrency support. 1. The concurrency model of Go language realizes efficient concurrent programming through goroutine and channel. 2. Interfaces and polymorphisms are implemented through interface methods, so that different types can be processed in a unified manner. 3. The basic usage demonstrates the efficiency of function definition and call. 4. In advanced usage, slices provide powerful functions of dynamic resizing. 5. Common errors such as race conditions can be detected and resolved through getest-race. 6. Performance optimization Reuse objects through sync.Pool to reduce garbage collection pressure.
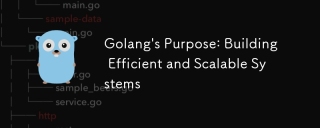
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
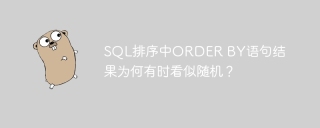
Confused about the sorting of SQL query results. In the process of learning SQL, you often encounter some confusing problems. Recently, the author is reading "MICK-SQL Basics"...
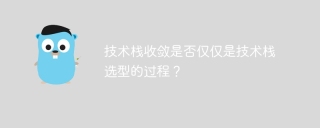
The relationship between technology stack convergence and technology selection In software development, the selection and management of technology stacks are a very critical issue. Recently, some readers have proposed...
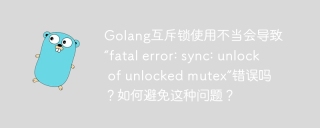
Golang ...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version
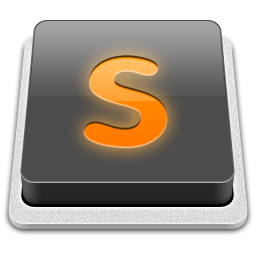
SublimeText3 Mac version
God-level code editing software (SublimeText3)
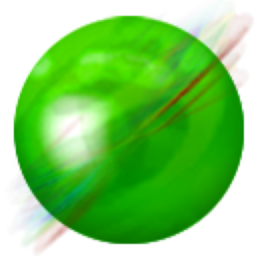
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
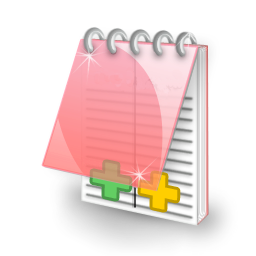
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function