


How do you determine and troubleshoot JavaScript stack size limitations in different browsers?
Debugging JavaScript Stack Size Limitations
Background
Client-side JavaScript stack overflows, particularly in Internet Explorer (IE), can arise due to a limited stack size compared to other browsers. This issue is often encountered when using third-party libraries that make numerous function calls.
Testing Stack Size Limit
To determine the stack size limit for different browsers, the following HTML test was developed:
<code class="html"> <title>Stack Size Limit Test</title> <script type="text/javascript"> function doSomething() { var i = 3200; doSomethingElse(i); } function doSomethingElse(i) { if (i == 0) return -1; doSomethingElse(i-1); } doSomething(); </script> </code>
The test revealed that IE8 raised a stack overflow at around 3200 function calls, while Firefox and Chrome supported significantly deeper recursion.
Identifying the Culprit
To identify the specific JavaScript function causing the stack overflow in IE, it would be useful to:
- Tie the stack-overflow exception to the function that raised it during runtime.
- Obtain a stack trace with the chain of functions in the stack at the time of the error.
JavaScript Stack Trace
JavaScript does not natively provide a stack trace capability. However, browser developer tools (e.g., Chrome DevTools, Firefox Developer Tools) offer ways to display the call stack at the time of an error.
Simpler Stack Size Limit Test
A simplified test using a recursive inc() function can also be used to determine the maximum stack size in a given browser:
<code class="js">var i = 0; function inc() { i++; inc(); } try { inc(); } catch(e) { // The StackOverflow sandbox adds one frame that is not being counted by this code // Incrementing once manually i++; console.log('Maximum stack size is', i, 'in your current browser'); }</code>
This test will print the maximum stack size to the console after the stack overflow occurs.
The above is the detailed content of How do you determine and troubleshoot JavaScript stack size limitations in different browsers?. For more information, please follow other related articles on the PHP Chinese website!
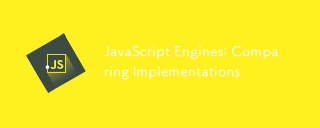
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
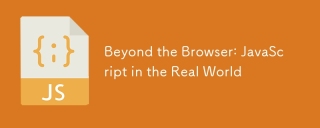
JavaScript's applications in the real world include server-side programming, mobile application development and Internet of Things control: 1. Server-side programming is realized through Node.js, suitable for high concurrent request processing. 2. Mobile application development is carried out through ReactNative and supports cross-platform deployment. 3. Used for IoT device control through Johnny-Five library, suitable for hardware interaction.
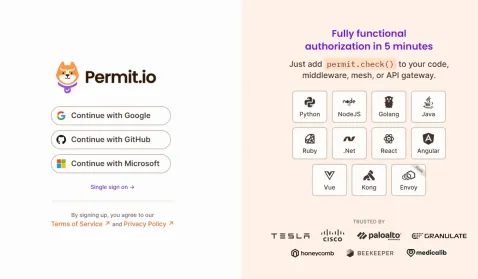
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
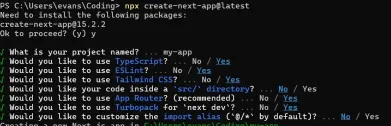
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
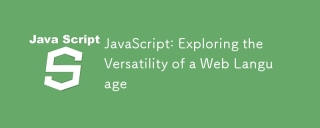
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
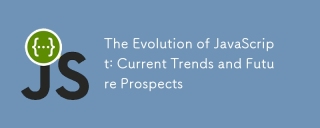
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
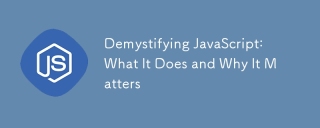
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
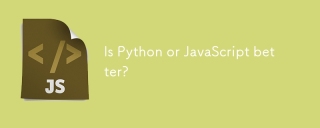
Python is more suitable for data science and machine learning, while JavaScript is more suitable for front-end and full-stack development. 1. Python is known for its concise syntax and rich library ecosystem, and is suitable for data analysis and web development. 2. JavaScript is the core of front-end development. Node.js supports server-side programming and is suitable for full-stack development.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
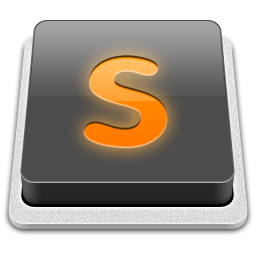
SublimeText3 Mac version
God-level code editing software (SublimeText3)