Prepending a Prefix to Array Keys Efficiently
When manipulating arrays, it's often necessary to add a prefix to all keys. This operation can be performed in several ways, but not all approaches are equally efficient.
Fastest Solution
The fastest solution is to use array_combine() in conjunction with array_map():
<code class="php">$prefix = "prefix"; $array = array_combine( array_map(fn($k) => $prefix . $k, array_keys($array)), $array );</code>
This method iterates over the original array keys, appends the prefix, and creates a new array using array_combine() to reassign the keys and values accordingly.
Other Solutions
Other solutions include:
- Using a foreach loop to iterate over each key and manually append the prefix, followed by unsetting the original key:
<code class="php">foreach ($array as $k => $v) { $array[$prefix . $k] = $v; unset($array[$k]); }</code>
- Utilizing a custom KeyPrefixer class with an __construct() method and mapArray() method for efficiently performing the prefix operation:
<code class="php">$prefix = "prefix"; $array = KeyPrefixer::prefix($array, $prefix);</code>
Historical Perspective
Prior to PHP 5.3, a different approach was necessary:
<code class="php">$prefixer = new KeyPrefixer($prefix); return $prefixer->mapArray($array);</code>
This method utilized a custom class and array_map() with an anonymous function to manipulate the keys and values.
The above is the detailed content of How to Efficiently Prepend a Prefix to Array Keys in PHP?. For more information, please follow other related articles on the PHP Chinese website!
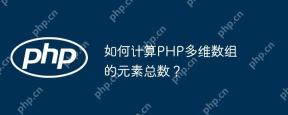
Calculating the total number of elements in a PHP multidimensional array can be done using recursive or iterative methods. 1. The recursive method counts by traversing the array and recursively processing nested arrays. 2. The iterative method uses the stack to simulate recursion to avoid depth problems. 3. The array_walk_recursive function can also be implemented, but it requires manual counting.
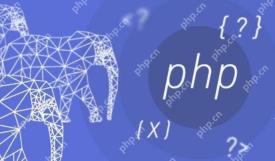
In PHP, the characteristic of a do-while loop is to ensure that the loop body is executed at least once, and then decide whether to continue the loop based on the conditions. 1) It executes the loop body before conditional checking, suitable for scenarios where operations need to be performed at least once, such as user input verification and menu systems. 2) However, the syntax of the do-while loop can cause confusion among newbies and may add unnecessary performance overhead.
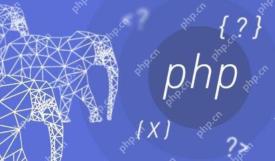
Efficient hashing strings in PHP can use the following methods: 1. Use the md5 function for fast hashing, but is not suitable for password storage. 2. Use the sha256 function to improve security. 3. Use the password_hash function to process passwords to provide the highest security and convenience.
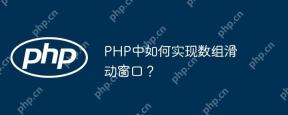
Implementing an array sliding window in PHP can be done by functions slideWindow and slideWindowAverage. 1. Use the slideWindow function to split an array into a fixed-size subarray. 2. Use the slideWindowAverage function to calculate the average value in each window. 3. For real-time data streams, asynchronous processing and outlier detection can be used using ReactPHP.
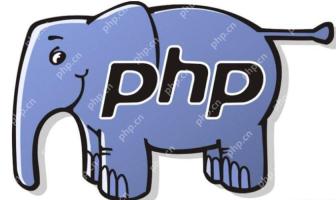
The __clone method in PHP is used to perform custom operations when object cloning. When cloning an object using the clone keyword, if the object has a __clone method, the method will be automatically called, allowing customized processing during the cloning process, such as resetting the reference type attribute to ensure the independence of the cloned object.
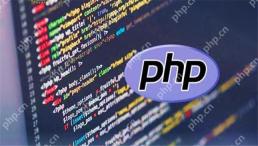
In PHP, goto statements are used to unconditionally jump to specific tags in the program. 1) It can simplify the processing of complex nested loops or conditional statements, but 2) Using goto may make the code difficult to understand and maintain, and 3) It is recommended to give priority to the use of structured control statements. Overall, goto should be used with caution and best practices are followed to ensure the readability and maintainability of the code.
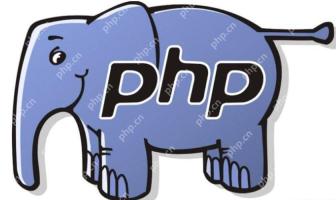
In PHP, data statistics can be achieved by using built-in functions, custom functions, and third-party libraries. 1) Use built-in functions such as array_sum() and count() to perform basic statistics. 2) Write custom functions to calculate complex statistics such as medians. 3) Use the PHP-ML library to perform advanced statistical analysis. Through these methods, data statistics can be performed efficiently.
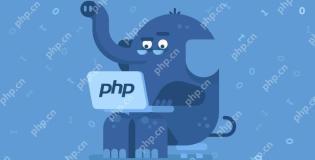
Yes, anonymous functions in PHP refer to functions without names. They can be passed as parameters to other functions and as return values of functions, making the code more flexible and efficient. When using anonymous functions, you need to pay attention to scope and performance issues.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
