


Handling Constrained Types as Function Arguments in Go 1.18 Generics
Go 1.18 introduced generics, allowing developers to create functions and types that operate on arbitrary data types. However, when trying to pass a constrained type as an argument to a function that expects a concrete type, the compiler may raise an error.
To illustrate this issue, consider the following example:
<code class="go">type Pokemon interface { ReceiveDamage(float64) InflictDamage(Pokemon) } type Float interface { float32 | float64 } type Charmander[F Float] struct { Health F AttackPower F }</code>
Here, Charmander implements the Pokemon interface and accepts a generic type parameter F that must be either float32 or float64.
<code class="go">func (c *Charmander[float64]) ReceiveDamage(damage float64) { c.Health -= damage } func (c *Charmander[float64]) InflictDamage(other Pokemon) { other.ReceiveDamage(c.AttackPower) }</code>
However, the compiler raises an error in the InflictDamage method, stating that c.AttackPower cannot be used as a float64 argument for the other.ReceiveDamage function. This is because, despite instantiating the Charmander struct as *Charmander[float64], the compiler still considers AttackPower to be of type F.
The solution to this issue lies in using type conversions. ReceiveDamage expects a float64, but AttackPower is still constrained to F. Therefore, we need to convert AttackPower to float64:
<code class="go">func (c *Charmander[T]) ReceiveDamage(damage float64) { c.Health -= T(damage) } func (c *Charmander[T]) InflictDamage(other Pokemon) { other.ReceiveDamage(float64(c.AttackPower)) }</code>
These conversions compile because float64 is convertible to all types in the type set of F (float32 and float64).
Note that the conversion T(damage) may result in precision loss if T is instantiated with float32. However, this is unlikely to be a concern in this specific use case.
The above is the detailed content of How to Pass Constrained Types as Function Arguments in Go 1.18 Generics?. For more information, please follow other related articles on the PHP Chinese website!
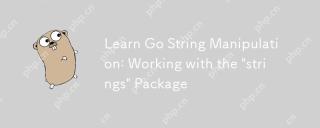
Go's "strings" package provides rich features to make string operation efficient and simple. 1) Use strings.Contains() to check substrings. 2) strings.Split() can be used to parse data, but it should be used with caution to avoid performance problems. 3) strings.Join() is suitable for formatting strings, but for small datasets, looping = is more efficient. 4) For large strings, it is more efficient to build strings using strings.Builder.
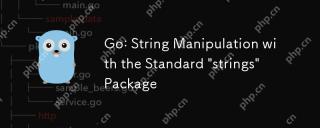
Go uses the "strings" package for string operations. 1) Use strings.Join function to splice strings. 2) Use the strings.Contains function to find substrings. 3) Use the strings.Replace function to replace strings. These functions are efficient and easy to use and are suitable for various string processing tasks.
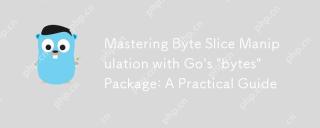
ThebytespackageinGoisessentialforefficientbyteslicemanipulation,offeringfunctionslikeContains,Index,andReplaceforsearchingandmodifyingbinarydata.Itenhancesperformanceandcodereadability,makingitavitaltoolforhandlingbinarydata,networkprotocols,andfileI
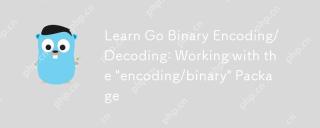
Go uses the "encoding/binary" package for binary encoding and decoding. 1) This package provides binary.Write and binary.Read functions for writing and reading data. 2) Pay attention to choosing the correct endian (such as BigEndian or LittleEndian). 3) Data alignment and error handling are also key to ensure the correctness and performance of the data.
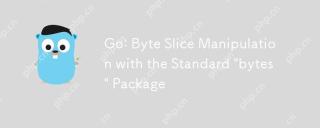
The"bytes"packageinGooffersefficientfunctionsformanipulatingbyteslices.1)Usebytes.Joinforconcatenatingslices,2)bytes.Bufferforincrementalwriting,3)bytes.Indexorbytes.IndexByteforsearching,4)bytes.Readerforreadinginchunks,and5)bytes.SplitNor
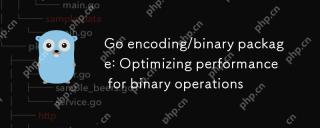
Theencoding/binarypackageinGoiseffectiveforoptimizingbinaryoperationsduetoitssupportforendiannessandefficientdatahandling.Toenhanceperformance:1)Usebinary.NativeEndianfornativeendiannesstoavoidbyteswapping.2)BatchReadandWriteoperationstoreduceI/Oover
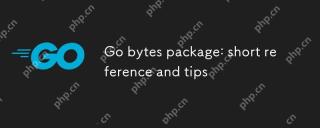
Go's bytes package is mainly used to efficiently process byte slices. 1) Using bytes.Buffer can efficiently perform string splicing to avoid unnecessary memory allocation. 2) The bytes.Equal function is used to quickly compare byte slices. 3) The bytes.Index, bytes.Split and bytes.ReplaceAll functions can be used to search and manipulate byte slices, but performance issues need to be paid attention to.
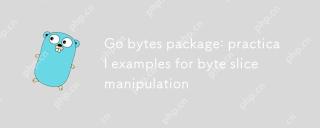
The byte package provides a variety of functions to efficiently process byte slices. 1) Use bytes.Contains to check the byte sequence. 2) Use bytes.Split to split byte slices. 3) Replace the byte sequence bytes.Replace. 4) Use bytes.Join to connect multiple byte slices. 5) Use bytes.Buffer to build data. 6) Combined bytes.Map for error processing and data verification.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!
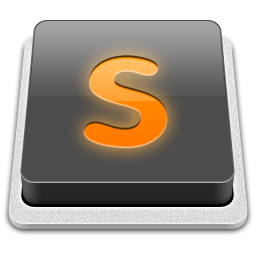
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
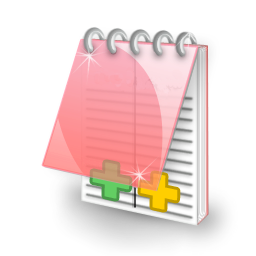
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SublimeText3 Chinese version
Chinese version, very easy to use
