


Logging 404 Errors for Http.FileServer
When serving files with http.FileServer, it can be useful to log instances where a requested file does not exist, resulting in a 404 status code. However, the default http.FileServer handler does not provide this functionality.
Extending the Functionality
To log 404 errors, you can extend the functionality of the http.FileServer handler. This can be achieved by wrapping the handler with a custom http.Handler or http.HandlerFunc.
Wrapping the Handler
The wrapper handler will invoke the original http.FileServer handler and then inspect the HTTP response status code. If it is an error (specifically 404 Not Found), it can log the error appropriately.
Response Status Code Wrapper
As http.ResponseWriter does not support reading the response status code directly, you can create a wrapper that stores the status code when it is set.
<code class="go">type StatusRespWr struct { http.ResponseWriter // Embeds http.ResponseWriter status int } func (w *StatusRespWr) WriteHeader(status int) { w.status = status // Store the status for later use w.ResponseWriter.WriteHeader(status) }</code>
Handler Wrapper
With the response status code wrapper in place, you can create a handler wrapper that logs errors:
<code class="go">func wrapHandler(h http.Handler) http.HandlerFunc { return func(w http.ResponseWriter, r *http.Request) { srw := &StatusRespWr{ResponseWriter: w} h.ServeHTTP(srw, r) if srw.status >= 400 { // 400+ codes indicate errors log.Printf("Error status code: %d when serving path: %s", srw.status, r.RequestURI) } } }</code>
Registering the Wrapped Handler
Finally, you can register the wrapped handler as a route in your HTTP server:
<code class="go">http.HandleFunc("/o/", wrapHandler(http.FileServer(http.Dir("/test"))))</code>
Example Output
When a non-existent file is requested, the wrapped handler will log an error message to the console:
2015/12/01 11:47:40 Error status code: 404 when serving path: /o/sub/b.txt2
The above is the detailed content of How can I log 404 errors when serving files with `http.FileServer` in Go?. For more information, please follow other related articles on the PHP Chinese website!
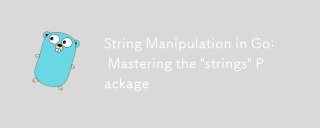
Mastering the strings package in Go language can improve text processing capabilities and development efficiency. 1) Use the Contains function to check substrings, 2) Use the Index function to find the substring position, 3) Join function efficiently splice string slices, 4) Replace function to replace substrings. Be careful to avoid common errors, such as not checking for empty strings and large string operation performance issues.
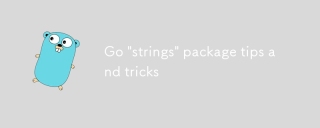
You should care about the strings package in Go because it simplifies string manipulation and makes the code clearer and more efficient. 1) Use strings.Join to efficiently splice strings; 2) Use strings.Fields to divide strings by blank characters; 3) Find substring positions through strings.Index and strings.LastIndex; 4) Use strings.ReplaceAll to replace strings; 5) Use strings.Builder to efficiently splice strings; 6) Always verify input to avoid unexpected results.
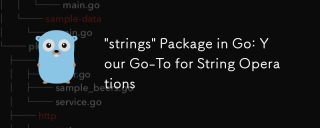
ThestringspackageinGoisessentialforefficientstringmanipulation.1)Itofferssimpleyetpowerfulfunctionsfortaskslikecheckingsubstringsandjoiningstrings.2)IthandlesUnicodewell,withfunctionslikestrings.Fieldsforwhitespace-separatedvalues.3)Forperformance,st
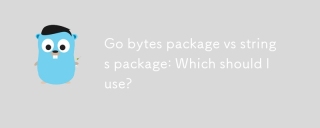
WhendecidingbetweenGo'sbytespackageandstringspackage,usebytes.Bufferforbinarydataandstrings.Builderforstringoperations.1)Usebytes.Bufferforworkingwithbyteslices,binarydata,appendingdifferentdatatypes,andwritingtoio.Writer.2)Usestrings.Builderforstrin
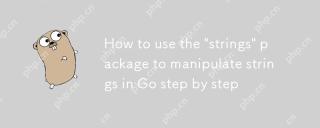
Go's strings package provides a variety of string manipulation functions. 1) Use strings.Contains to check substrings. 2) Use strings.Split to split the string into substring slices. 3) Merge strings through strings.Join. 4) Use strings.TrimSpace or strings.Trim to remove blanks or specified characters at the beginning and end of a string. 5) Replace all specified substrings with strings.ReplaceAll. 6) Use strings.HasPrefix or strings.HasSuffix to check the prefix or suffix of the string.
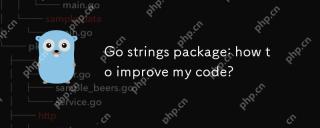
Using the Go language strings package can improve code quality. 1) Use strings.Join() to elegantly connect string arrays to avoid performance overhead. 2) Combine strings.Split() and strings.Contains() to process text and pay attention to case sensitivity issues. 3) Avoid abuse of strings.Replace() and consider using regular expressions for a large number of substitutions. 4) Use strings.Builder to improve the performance of frequently splicing strings.
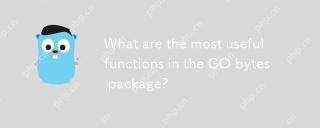
Go's bytes package provides a variety of practical functions to handle byte slicing. 1.bytes.Contains is used to check whether the byte slice contains a specific sequence. 2.bytes.Split is used to split byte slices into smallerpieces. 3.bytes.Join is used to concatenate multiple byte slices into one. 4.bytes.TrimSpace is used to remove the front and back blanks of byte slices. 5.bytes.Equal is used to compare whether two byte slices are equal. 6.bytes.Index is used to find the starting index of sub-slices in largerslices.
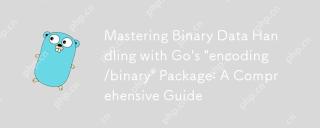
Theencoding/binarypackageinGoisessentialbecauseitprovidesastandardizedwaytoreadandwritebinarydata,ensuringcross-platformcompatibilityandhandlingdifferentendianness.ItoffersfunctionslikeRead,Write,ReadUvarint,andWriteUvarintforprecisecontroloverbinary


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor
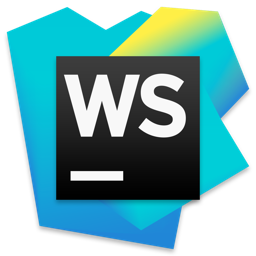
WebStorm Mac version
Useful JavaScript development tools

SublimeText3 English version
Recommended: Win version, supports code prompts!
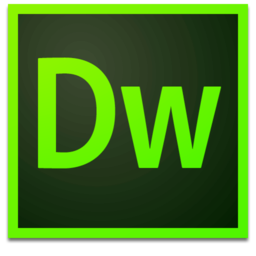
Dreamweaver Mac version
Visual web development tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
