


How to Retrieve the Latest Records for Each Unique Value in a Column using Laravel Eloquent?
How to Get All Rows with Max Created_at in Laravel Eloquent
In Laravel's Eloquent ORM, you may encounter scenarios where you need to retrieve the latest rows for each distinct value in a particular column. This can be achieved using a combination of subqueries and aggregation functions.
Consider a scenario where you have a database table containing the following columns:
id seller_id amount created_at
Suppose you want to obtain the most recent record for each unique seller_id. Here's how you can accomplish this using a raw SQL query:
<code class="sql">select s.* from snapshot s left join snapshot s1 on s.seller_id = s1.seller_id and s.created_at <p>In this query, we utilize a left join to compare each record in the snapshot table (aliased as s) with all other records having the same seller_id. We then use the AND condition to filter out records where s.created_at is less than s1.created_at. Finally, we include a WHERE clause to exclude any records with a matching seller_id in the right table (s1), effectively isolating the latest record for each seller_id.</p> <p>To achieve the same result using the Laravel query builder, you can execute the following code:</p> <pre class="brush:php;toolbar:false"><code class="php">DB::table('snapshot as s') ->select('s.*') ->leftJoin('snapshot as s1', function ($join) { $join->on('s.seller_id', '=', 's1.seller_id') ->whereRaw(DB::raw('s.created_at whereNull('s1.seller_id') ->get();</code>
This query builder approach mirrors the logic of the raw SQL query, encapsulating it within Laravel's fluent interface. It returns a collection of the latest records for each distinct seller_id present in the snapshot table.
The above is the detailed content of How to Retrieve the Latest Records for Each Unique Value in a Column using Laravel Eloquent?. For more information, please follow other related articles on the PHP Chinese website!
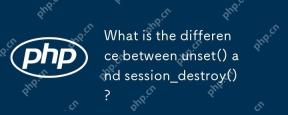
Thedifferencebetweenunset()andsession_destroy()isthatunset()clearsspecificsessionvariableswhilekeepingthesessionactive,whereassession_destroy()terminatestheentiresession.1)Useunset()toremovespecificsessionvariableswithoutaffectingthesession'soveralls
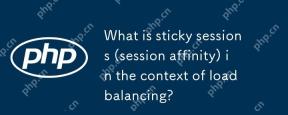
Stickysessionsensureuserrequestsareroutedtothesameserverforsessiondataconsistency.1)SessionIdentificationassignsuserstoserversusingcookiesorURLmodifications.2)ConsistentRoutingdirectssubsequentrequeststothesameserver.3)LoadBalancingdistributesnewuser
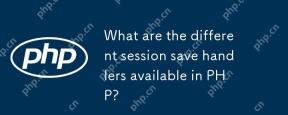
PHPoffersvarioussessionsavehandlers:1)Files:Default,simplebutmaybottleneckonhigh-trafficsites.2)Memcached:High-performance,idealforspeed-criticalapplications.3)Redis:SimilartoMemcached,withaddedpersistence.4)Databases:Offerscontrol,usefulforintegrati
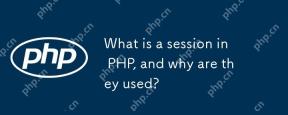
Session in PHP is a mechanism for saving user data on the server side to maintain state between multiple requests. Specifically, 1) the session is started by the session_start() function, and data is stored and read through the $_SESSION super global array; 2) the session data is stored in the server's temporary files by default, but can be optimized through database or memory storage; 3) the session can be used to realize user login status tracking and shopping cart management functions; 4) Pay attention to the secure transmission and performance optimization of the session to ensure the security and efficiency of the application.
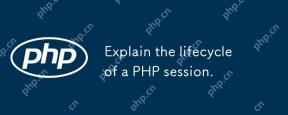
PHPsessionsstartwithsession_start(),whichgeneratesauniqueIDandcreatesaserverfile;theypersistacrossrequestsandcanbemanuallyendedwithsession_destroy().1)Sessionsbeginwhensession_start()iscalled,creatingauniqueIDandserverfile.2)Theycontinueasdataisloade
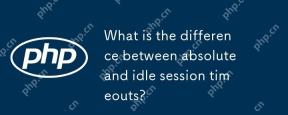
Absolute session timeout starts at the time of session creation, while an idle session timeout starts at the time of user's no operation. Absolute session timeout is suitable for scenarios where strict control of the session life cycle is required, such as financial applications; idle session timeout is suitable for applications that want users to keep their session active for a long time, such as social media.
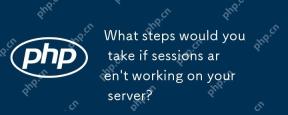
The server session failure can be solved through the following steps: 1. Check the server configuration to ensure that the session is set correctly. 2. Verify client cookies, confirm that the browser supports it and send it correctly. 3. Check session storage services, such as Redis, to ensure that they are running normally. 4. Review the application code to ensure the correct session logic. Through these steps, conversation problems can be effectively diagnosed and repaired and user experience can be improved.
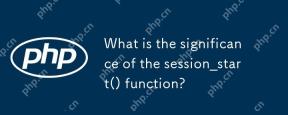
session_start()iscrucialinPHPformanagingusersessions.1)Itinitiatesanewsessionifnoneexists,2)resumesanexistingsession,and3)setsasessioncookieforcontinuityacrossrequests,enablingapplicationslikeuserauthenticationandpersonalizedcontent.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
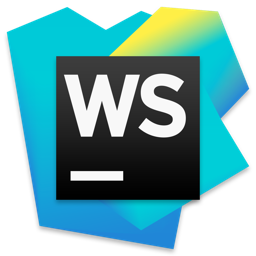
WebStorm Mac version
Useful JavaScript development tools

SublimeText3 Chinese version
Chinese version, very easy to use
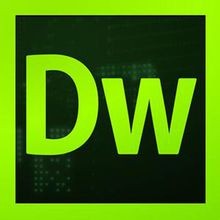
Dreamweaver CS6
Visual web development tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
