Wait 5 Seconds Before Executing Next Line
In JavaScript, the setTimeout() function schedules a function to be executed after a specified number of milliseconds. However, it does not halt the execution of the code, meaning that the next line of code will be executed immediately after the setTimeout() call, regardless of the specified delay.
Your Code
Your code aims to wait 5 seconds before checking if newState is equal to -1. However, since setTimeout() does not block the code, the checking happens immediately, before the 5-second delay occurs.
Solution
To achieve the desired behavior, you need to use a mechanism that can pause the execution of your code for 5 seconds. There are two modern approaches you can consider:
1. Async/Await Syntax (Browser Only)
ES6 introduced the async/await syntax, which allows you to write asynchronous code in a more synchronous style. By marking your function as async, you can use the await keyword to pause execution until a promise is resolved.
<code class="javascript">const delay = ms => new Promise(resolve => setTimeout(resolve, ms)); const stateChange = async newState => { await delay(5000); if (newState === -1) { alert('VIDEO HAS STOPPED'); } };</code>
2. Timers/Promises Module (Node.js Only)
In Node.js 16, the timers/promises module provides a promise-based version of setTimeout. This allows you to pause execution and wait for the delay to complete before continuing.
<code class="javascript">import { setTimeout } from "timers/promises"; const stateChange = async newState => { await setTimeout(5000); if (newState === -1) { console.log('VIDEO HAS STOPPED'); } };</code>
Both of these approaches will ensure that the code execution is halted for 5 seconds before the newState check is performed.
The above is the detailed content of How to Wait 5 Seconds Before Executing the Next Line in JavaScript?. For more information, please follow other related articles on the PHP Chinese website!
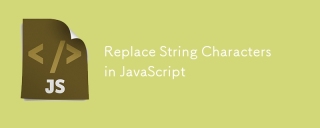
Detailed explanation of JavaScript string replacement method and FAQ This article will explore two ways to replace string characters in JavaScript: internal JavaScript code and internal HTML for web pages. Replace string inside JavaScript code The most direct way is to use the replace() method: str = str.replace("find","replace"); This method replaces only the first match. To replace all matches, use a regular expression and add the global flag g: str = str.replace(/fi
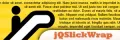
Leverage jQuery for Effortless Web Page Layouts: 8 Essential Plugins jQuery simplifies web page layout significantly. This article highlights eight powerful jQuery plugins that streamline the process, particularly useful for manual website creation
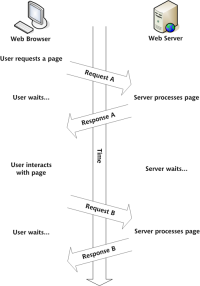
So here you are, ready to learn all about this thing called AJAX. But, what exactly is it? The term AJAX refers to a loose grouping of technologies that are used to create dynamic, interactive web content. The term AJAX, originally coined by Jesse J
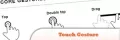
This post compiles helpful cheat sheets, reference guides, quick recipes, and code snippets for Android, Blackberry, and iPhone app development. No developer should be without them! Touch Gesture Reference Guide (PDF) A valuable resource for desig

jQuery is a great JavaScript framework. However, as with any library, sometimes it’s necessary to get under the hood to discover what’s going on. Perhaps it’s because you’re tracing a bug or are just curious about how jQuery achieves a particular UI
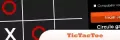
10 fun jQuery game plugins to make your website more attractive and enhance user stickiness! While Flash is still the best software for developing casual web games, jQuery can also create surprising effects, and while not comparable to pure action Flash games, in some cases you can also have unexpected fun in your browser. jQuery tic toe game The "Hello world" of game programming now has a jQuery version. Source code jQuery Crazy Word Composition Game This is a fill-in-the-blank game, and it can produce some weird results due to not knowing the context of the word. Source code jQuery mine sweeping game
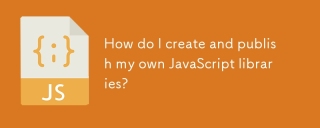
Article discusses creating, publishing, and maintaining JavaScript libraries, focusing on planning, development, testing, documentation, and promotion strategies.
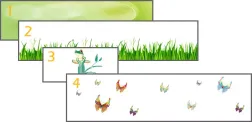
This tutorial demonstrates how to create a captivating parallax background effect using jQuery. We'll build a header banner with layered images that create a stunning visual depth. The updated plugin works with jQuery 1.6.4 and later. Download the


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
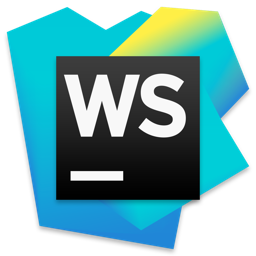
WebStorm Mac version
Useful JavaScript development tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Zend Studio 13.0.1
Powerful PHP integrated development environment
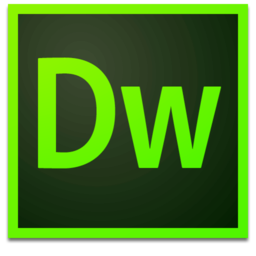
Dreamweaver Mac version
Visual web development tools

Notepad++7.3.1
Easy-to-use and free code editor
