


Understanding the Issue
In your code, you handle population counts within a two-level loop and try to optimize the inner loop with assembly. The loop iterates through a byte slice and uses the __mm_add_epi32_inplace_purego function to add positional popcounts to an array.
Optimization via Assembly
To optimize the inner loop, you can implement __mm_add_epi32_inplace_purego in assembly. Below is the suggested optimized version of the function:
<code class="assembly">.text .globl __mm_add_epi32_inplace_purego __mm_add_epi32_inplace_purego: movq rdi, [rsi] movq rsi, [rdi+8] addq rsi, rdi movups (%rsi, %rax, 8), %xmm0 addq , %rsi movups (%rsi, %rax, 8), %xmm1 paddusbd %xmm0, %xmm0 paddusbd %xmm1, %xmm1 vextracti128 <pre class="brush:php;toolbar:false"><code class="assembly">.text .globl __optimized_population_count_loop __optimized_population_count_loop: movq rdi, [rsi] leaq (0, %rdi, 4), %rdx # multiple rdi by 4, rdx = counts movq rsp, r11 and rsp, -16 subq r15, r11 movq r15, r9 mov rdi, (%rsi) movq r15, rsi mov %rsi, rsi pxor %eax, %eax dec %rsi .loop: inc %rsi addq , rsi cmp rsi, rdi cmovge %rsi, rsi movsw (%rdi, %rax, 2), %ax movsw (%rsi, %rax, 2), %dx movw %ax, (%rdx) movw %dx, 2(%rdx) .end_loop:</code>, %xmm0, %eax vextracti128 , %xmm1, %edx addl %eax, (%rdi) addl %edx, 4(%rdi) addl %r8d, (%rdi) ret
Explanation:
This assembly code optimizes the function using packed SSE instructions. It:
- Computes popcounts in 16-bit blocks using paddusbd.
- Extracts the low 128-bit portion using vextracti128.
- Adds the results to the [8]int32 array at the address given by %rdi.
Enhanced Whole Loop with Assembly
Explanation:
The complete loop is now optimized in assembly. It uses:
- A loop to process 2-byte chunks.
- Streaming loads via consecutive addq $32, rsi to avoid cache misses.
- A fast and compact way to save the results using movw.
Conclusion
This optimized version should significantly improve the performance of your algorithm for computing positional population counts.
The above is the detailed content of How can SSE instructions and assembly optimization improve the performance of a population count algorithm with a two-level loop?. For more information, please follow other related articles on the PHP Chinese website!
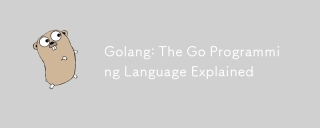
The core features of Go include garbage collection, static linking and concurrency support. 1. The concurrency model of Go language realizes efficient concurrent programming through goroutine and channel. 2. Interfaces and polymorphisms are implemented through interface methods, so that different types can be processed in a unified manner. 3. The basic usage demonstrates the efficiency of function definition and call. 4. In advanced usage, slices provide powerful functions of dynamic resizing. 5. Common errors such as race conditions can be detected and resolved through getest-race. 6. Performance optimization Reuse objects through sync.Pool to reduce garbage collection pressure.
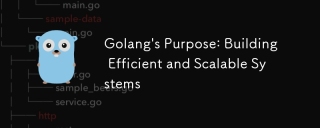
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
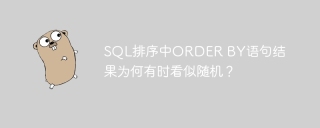
Confused about the sorting of SQL query results. In the process of learning SQL, you often encounter some confusing problems. Recently, the author is reading "MICK-SQL Basics"...
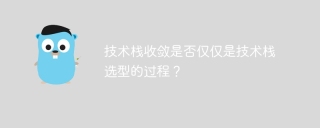
The relationship between technology stack convergence and technology selection In software development, the selection and management of technology stacks are a very critical issue. Recently, some readers have proposed...
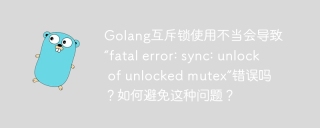
Golang ...
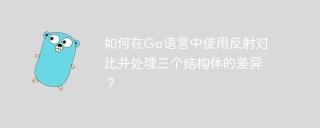
How to compare and handle three structures in Go language. In Go programming, it is sometimes necessary to compare the differences between two structures and apply these differences to the...
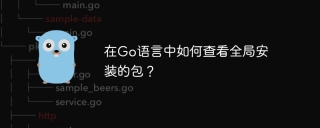
How to view globally installed packages in Go? In the process of developing with Go language, go often uses...
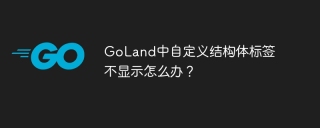
What should I do if the custom structure labels in GoLand are not displayed? When using GoLand for Go language development, many developers will encounter custom structure tags...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
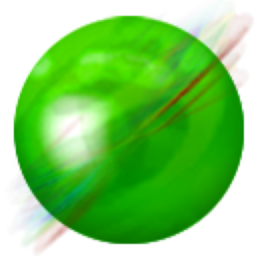
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SublimeText3 Chinese version
Chinese version, very easy to use

Atom editor mac version download
The most popular open source editor