951. Flip Equivalent Binary Trees
Difficulty: Medium
Topics: Tree, Depth-First Search, Binary Tree
For a binary tree T, we can define a flip operation as follows: choose any node, and swap the left and right child subtrees.
A binary tree X is flip equivalent to a binary tree Y if and only if we can make X equal to Y after some number of flip operations.
Given the roots of two binary trees root1 and root2, return true if the two trees are flip equivalent or false otherwise.
Example 1:
- Input: root1 = [1,2,3,4,5,6,null,null,null,7,8], root2 = [1,3,2,null,6,4,5,null,null,null,null,8,7]
- Output: true
- Explanation: We flipped at nodes with values 1, 3, and 5.
Example 2:
- Input: root1 = [], root2 = []
- Output: true
Example 3:
- Input: root1 = [], root2 = [1]
- Output: false
Constraints:
- The number of nodes in each tree is in the range [0, 100].
- Each tree will have unique node values in the range [0, 99].
Solution:
We can use a recursive depth-first search (DFS). The idea is that two trees are flip equivalent if their root values are the same, and the subtrees are either the same (without any flips) or they become the same after flipping the left and right children at some nodes.
Plan:
-
Base Cases:
- If both root1 and root2 are null, they are trivially flip equivalent.
- If only one of them is null, they can't be equivalent.
- If the root values of root1 and root2 are different, they can't be equivalent.
-
Recursive Case:
- Recursively check two possibilities:
- The left subtree of root1 is flip equivalent to the left subtree of root2, and the right subtree of root1 is flip equivalent to the right subtree of root2 (i.e., no flip).
- The left subtree of root1 is flip equivalent to the right subtree of root2, and the right subtree of root1 is flip equivalent to the left subtree of root2 (i.e., flip the children).
- Recursively check two possibilities:
Let's implement this solution in PHP: 951. Flip Equivalent Binary Trees
<?php // Definition for a binary tree node. class TreeNode { public $val; public $left; public $right; function __construct($val = 0, $left = null, $right = null) { $this->val = $val; $this->left = $left; $this->right = $right; } } /** * @param TreeNode $root1 * @param TreeNode $root2 * @return Boolean */ function flipEquiv($root1, $root2) { ... ... ... /** * go to ./solution.php */ } // Example usage: $root1 = new TreeNode(1, new TreeNode(2, new TreeNode(4), new TreeNode(5, new TreeNode(7), new TreeNode(8))), new TreeNode(3, new TreeNode(6), null) ); $root2 = new TreeNode(1, new TreeNode(3, null, new TreeNode(6)), new TreeNode(2, new TreeNode(4), new TreeNode(5, new TreeNode(8), new TreeNode(7))) ); var_dump(flipEquiv($root1, $root2)); // Output: bool(true) ?>
Explanation:
TreeNode Class: The TreeNode class represents a node in the binary tree, with a constructor to initialize the node's value, left child, and right child.
-
flipEquiv Function:
- The base cases handle when both nodes are null, when one is null, or when the values do not match.
- The recursive case checks both possibilities (no flip vs. flip), ensuring the subtrees are flip equivalent under either condition.
Time Complexity:
- The function checks every node in both trees, and each recursive call processes two subtrees. Therefore, the time complexity is O(N), where N is the number of nodes in the tree.
Space Complexity:
- The space complexity is O(H), where H is the height of the tree, because of the recursive stack. In the worst case (for a skewed tree), this could be O(N).
Contact Links
If you found this series helpful, please consider giving the repository a star on GitHub or sharing the post on your favorite social networks ?. Your support would mean a lot to me!
If you want more helpful content like this, feel free to follow me:
- GitHub
The above is the detailed content of . Flip Equivalent Binary Trees. For more information, please follow other related articles on the PHP Chinese website!
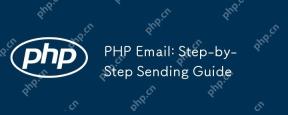
PHPisusedforsendingemailsduetoitsintegrationwithservermailservicesandexternalSMTPproviders,automatingnotificationsandmarketingcampaigns.1)SetupyourPHPenvironmentwithawebserverandPHP,ensuringthemailfunctionisenabled.2)UseabasicscriptwithPHP'smailfunct
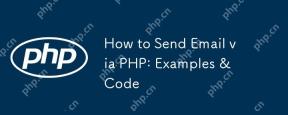
The best way to send emails is to use the PHPMailer library. 1) Using the mail() function is simple but unreliable, which may cause emails to enter spam or cannot be delivered. 2) PHPMailer provides better control and reliability, and supports HTML mail, attachments and SMTP authentication. 3) Make sure SMTP settings are configured correctly and encryption (such as STARTTLS or SSL/TLS) is used to enhance security. 4) For large amounts of emails, consider using a mail queue system to optimize performance.
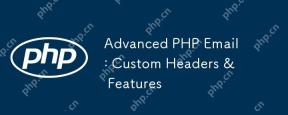
CustomheadersandadvancedfeaturesinPHPemailenhancefunctionalityandreliability.1)Customheadersaddmetadatafortrackingandcategorization.2)HTMLemailsallowformattingandinteractivity.3)AttachmentscanbesentusinglibrarieslikePHPMailer.4)SMTPauthenticationimpr
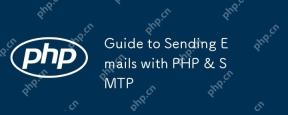
Sending mail using PHP and SMTP can be achieved through the PHPMailer library. 1) Install and configure PHPMailer, 2) Set SMTP server details, 3) Define the email content, 4) Send emails and handle errors. Use this method to ensure the reliability and security of emails.
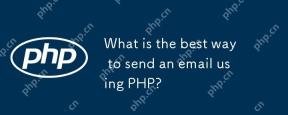
ThebestapproachforsendingemailsinPHPisusingthePHPMailerlibraryduetoitsreliability,featurerichness,andeaseofuse.PHPMailersupportsSMTP,providesdetailederrorhandling,allowssendingHTMLandplaintextemails,supportsattachments,andenhancessecurity.Foroptimalu
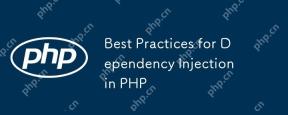
The reason for using Dependency Injection (DI) is that it promotes loose coupling, testability, and maintainability of the code. 1) Use constructor to inject dependencies, 2) Avoid using service locators, 3) Use dependency injection containers to manage dependencies, 4) Improve testability through injecting dependencies, 5) Avoid over-injection dependencies, 6) Consider the impact of DI on performance.
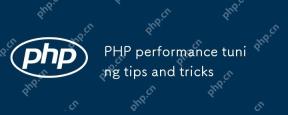
PHPperformancetuningiscrucialbecauseitenhancesspeedandefficiency,whicharevitalforwebapplications.1)CachingwithAPCureducesdatabaseloadandimprovesresponsetimes.2)Optimizingdatabasequeriesbyselectingnecessarycolumnsandusingindexingspeedsupdataretrieval.
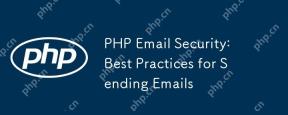
ThebestpracticesforsendingemailssecurelyinPHPinclude:1)UsingsecureconfigurationswithSMTPandSTARTTLSencryption,2)Validatingandsanitizinginputstopreventinjectionattacks,3)EncryptingsensitivedatawithinemailsusingOpenSSL,4)Properlyhandlingemailheaderstoa


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
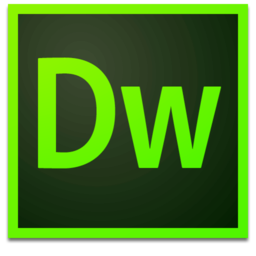
Dreamweaver Mac version
Visual web development tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SublimeText3 Chinese version
Chinese version, very easy to use

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
