Cancelling Boost Asio Deadline Timer Safely
This article addresses the proper cancellation of boost::asio::basic_waitable_timer<:chrono::steady_clock> timers.
Problem:
Initially, the proposed solution to cancel a timer via timer.get_io_service().post([&]{timer.cancel();}) might appear to fail. The timer does not abort even after calling cancel().
Reason:
The issue lies in the timer's behavior post-cancellation. It does not terminate outstanding asynchronous operations and simply starts a new asynchronous wait when the completion handler is invoked.
Solution:
To cancel a timer safely, it's essential to check if any asynchronous operations are currently in progress. This check can be performed by comparing the timer's expiry time with the current time:
<code class="cpp">timer.get_io_service().post([](){ if (timer.expires_from_now() >= std::chrono::steady_clock::duration(0)) { timer.cancel(); } });</code>
Enhanced Cancellation:
In addition to canceling the current operation, it can be beneficial to communicate a shutdown signal to the completion handler. This can be achieved by setting the timer's expiry time to a special value like Timer::clock_type::time_point::min().
<code class="cpp">timer.expires_at(Timer::clock_type::time_point::min());</code>
This allows the completion handler to identify the shutdown state:
<code class="cpp">void handle_timeout(const boost::system::error_code& ec) { if (timer.expires_at() != Timer::time_point::min()) { // timer is not in shutdown state // ... } else { // timer is in shutdown state std::cerr <p>By implementing these techniques, the cancellation of boost::asio::basic_waitable_timer<:chrono::steady_clock> timers becomes both safe and robust.</:chrono::steady_clock></p></code>
The above is the detailed content of How to Safely Cancel a boost::asio::basic_waitable_timer?. For more information, please follow other related articles on the PHP Chinese website!
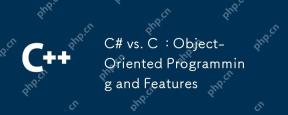
There are significant differences in how C# and C implement and features in object-oriented programming (OOP). 1) The class definition and syntax of C# are more concise and support advanced features such as LINQ. 2) C provides finer granular control, suitable for system programming and high performance needs. Both have their own advantages, and the choice should be based on the specific application scenario.
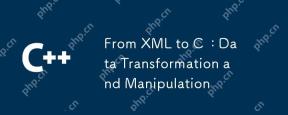
Converting from XML to C and performing data operations can be achieved through the following steps: 1) parsing XML files using tinyxml2 library, 2) mapping data into C's data structure, 3) using C standard library such as std::vector for data operations. Through these steps, data converted from XML can be processed and manipulated efficiently.
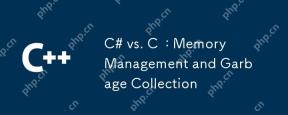
C# uses automatic garbage collection mechanism, while C uses manual memory management. 1. C#'s garbage collector automatically manages memory to reduce the risk of memory leakage, but may lead to performance degradation. 2.C provides flexible memory control, suitable for applications that require fine management, but should be handled with caution to avoid memory leakage.

C still has important relevance in modern programming. 1) High performance and direct hardware operation capabilities make it the first choice in the fields of game development, embedded systems and high-performance computing. 2) Rich programming paradigms and modern features such as smart pointers and template programming enhance its flexibility and efficiency. Although the learning curve is steep, its powerful capabilities make it still important in today's programming ecosystem.
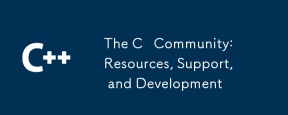
C Learners and developers can get resources and support from StackOverflow, Reddit's r/cpp community, Coursera and edX courses, open source projects on GitHub, professional consulting services, and CppCon. 1. StackOverflow provides answers to technical questions; 2. Reddit's r/cpp community shares the latest news; 3. Coursera and edX provide formal C courses; 4. Open source projects on GitHub such as LLVM and Boost improve skills; 5. Professional consulting services such as JetBrains and Perforce provide technical support; 6. CppCon and other conferences help careers
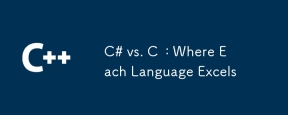
C# is suitable for projects that require high development efficiency and cross-platform support, while C is suitable for applications that require high performance and underlying control. 1) C# simplifies development, provides garbage collection and rich class libraries, suitable for enterprise-level applications. 2)C allows direct memory operation, suitable for game development and high-performance computing.
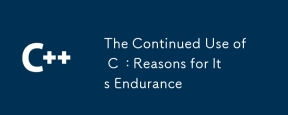
C Reasons for continuous use include its high performance, wide application and evolving characteristics. 1) High-efficiency performance: C performs excellently in system programming and high-performance computing by directly manipulating memory and hardware. 2) Widely used: shine in the fields of game development, embedded systems, etc. 3) Continuous evolution: Since its release in 1983, C has continued to add new features to maintain its competitiveness.
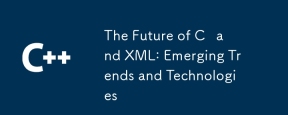
The future development trends of C and XML are: 1) C will introduce new features such as modules, concepts and coroutines through the C 20 and C 23 standards to improve programming efficiency and security; 2) XML will continue to occupy an important position in data exchange and configuration files, but will face the challenges of JSON and YAML, and will develop in a more concise and easy-to-parse direction, such as the improvements of XMLSchema1.1 and XPath3.1.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
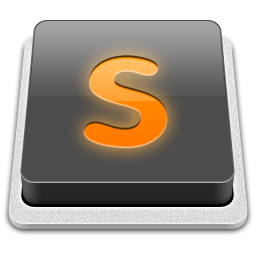
SublimeText3 Mac version
God-level code editing software (SublimeText3)

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SublimeText3 Linux new version
SublimeText3 Linux latest version

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.