


CSV Double Quote Escaping in Go's encoding/csv
When working with CSV files in Go using the encoding/csv package, it's crucial to understand how double quote characters ("") are handled. Double quotes are used to enclose strings containing special characters like commas, which can otherwise break the CSV format.
In Go, when writing to a CSV file, it's important to escape double quote characters within strings. The encoding/csv package does this automatically, adding extra double quotes around any double quotes in the string. This is part of the CSV standard, which requires double quotes to be escaped for parsing accuracy.
Example:
<code class="go">import ( "encoding/csv" "fmt" "os" ) func main() { f, err := os.Create("./test.csv") if err != nil { log.Fatal("Error: %s", err) } defer f.Close() w := csv.NewWriter(f) s := "Cr@zy text with , and \ and \" etc" record := []string{ "Unquoted string", s, } fmt.Println(record) w.Write(record) // Quote the string to escape double quotes record = []string{ "Quoted string", fmt.Sprintf("%q", s), } fmt.Println(record) w.Write(record) w.Flush() }</code>
When the script runs, the output will show the strings surrounded by double quotes:
[Unquoted string Cr@zy text with , and \ and " etc] [Quoted string "Cr@zy text with , and \ and \" etc"]
However, when reading from the CSV file, the encoding/csv package automatically unescapes any escaped double quotes. This means that the strings will be parsed correctly, without any additional double quotes.
Example Read Function:
<code class="go">func readCSV() { file, err := os.Open("./test.csv") defer file.Close() cr := csv.NewReader(file) records, err := cr.ReadAll() if err != nil { log.Fatal("Error: %s", err) } for _, record := range records { fmt.Println(record) } }</code>
When the read function is called, you will see the output:
[Unquoted string Cr@zy text with , and \ and " etc] [Quoted string Cr@zy text with , and \ and " etc]
This shows how the double quotes are handled during write and read operations, ensuring that the data is both stored and retrieved correctly.
The above is the detailed content of How does Go\'s `encoding/csv` package handle double quote escaping when writing and reading CSV files?. For more information, please follow other related articles on the PHP Chinese website!
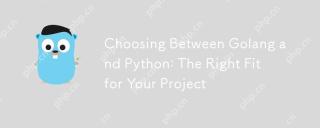
Golangisidealforperformance-criticalapplicationsandconcurrentprogramming,whilePythonexcelsindatascience,rapidprototyping,andversatility.1)Forhigh-performanceneeds,chooseGolangduetoitsefficiencyandconcurrencyfeatures.2)Fordata-drivenprojects,Pythonisp
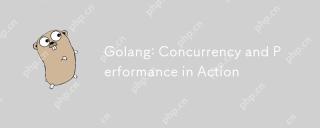
Golang achieves efficient concurrency through goroutine and channel: 1.goroutine is a lightweight thread, started with the go keyword; 2.channel is used for secure communication between goroutines to avoid race conditions; 3. The usage example shows basic and advanced usage; 4. Common errors include deadlocks and data competition, which can be detected by gorun-race; 5. Performance optimization suggests reducing the use of channel, reasonably setting the number of goroutines, and using sync.Pool to manage memory.
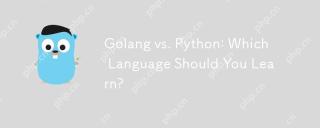
Golang is more suitable for system programming and high concurrency applications, while Python is more suitable for data science and rapid development. 1) Golang is developed by Google, statically typing, emphasizing simplicity and efficiency, and is suitable for high concurrency scenarios. 2) Python is created by Guidovan Rossum, dynamically typed, concise syntax, wide application, suitable for beginners and data processing.
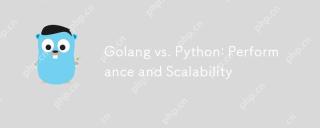
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
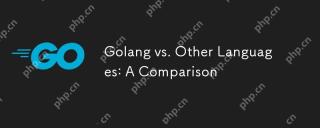
Go language has unique advantages in concurrent programming, performance, learning curve, etc.: 1. Concurrent programming is realized through goroutine and channel, which is lightweight and efficient. 2. The compilation speed is fast and the operation performance is close to that of C language. 3. The grammar is concise, the learning curve is smooth, and the ecosystem is rich.
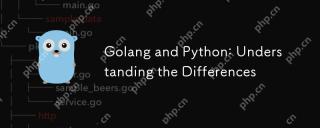
The main differences between Golang and Python are concurrency models, type systems, performance and execution speed. 1. Golang uses the CSP model, which is suitable for high concurrent tasks; Python relies on multi-threading and GIL, which is suitable for I/O-intensive tasks. 2. Golang is a static type, and Python is a dynamic type. 3. Golang compiled language execution speed is fast, and Python interpreted language development is fast.
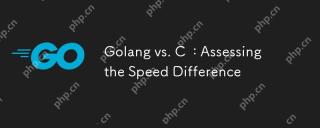
Golang is usually slower than C, but Golang has more advantages in concurrent programming and development efficiency: 1) Golang's garbage collection and concurrency model makes it perform well in high concurrency scenarios; 2) C obtains higher performance through manual memory management and hardware optimization, but has higher development complexity.
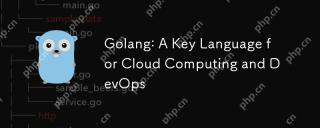
Golang is widely used in cloud computing and DevOps, and its advantages lie in simplicity, efficiency and concurrent programming capabilities. 1) In cloud computing, Golang efficiently handles concurrent requests through goroutine and channel mechanisms. 2) In DevOps, Golang's fast compilation and cross-platform features make it the first choice for automation tools.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

SublimeText3 Linux new version
SublimeText3 Linux latest version
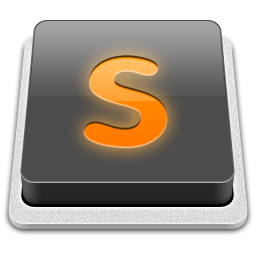
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SublimeText3 English version
Recommended: Win version, supports code prompts!

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.