


Sharing Custom Data Types Between a Go Plugin and an Application
In Go plugins, it's possible to share custom data types between the plugin and the application, but not through direct type assertion.
Shared Data Types in Separate Packages
To define shared types, create them in a separate package and import it into both the plugin and the main application. For example:
Shared Type Package:
<code class="go">package shared type Person struct { Name string }</code>
Plugin Code:
<code class="go">package main import ( "shared" ) var P = shared.Person{Name: "Emma"}</code>
Main Application Code:
<code class="go">package main import ( "fmt" "plugin" "shared" "os" ) func main() { plug, err := plugin.Open("./plugin.so") if err != nil { fmt.Println(err) os.Exit(1) } // Lookup shared type symbol sym, err := plug.Lookup("P") if err != nil { fmt.Println(err) os.Exit(1) } // Type-assert symbol into shared type var p shared.Person p, ok := sym.(shared.Person) if !ok { fmt.Println("Wrong symbol type") os.Exit(1) } // Use shared type as expected fmt.Println(p.Name) }</code>
Non-Pointer Types and Pointer Symbols
When looking up variable symbols from a plugin, the result is a pointer to the variable, even if it's a non-pointer type. This allows modification of the variable's value from the plugin.
Conclusion
By using shared types defined in a separate package, it's possible to pass custom data types between a Go plugin and an application, enabling efficient data exchange and extending the plugin's capabilities.
The above is the detailed content of How Can I Share Custom Data Types Between a Go Plugin and Its Application?. For more information, please follow other related articles on the PHP Chinese website!
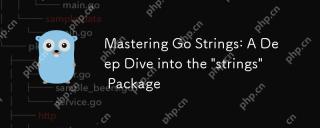
You should care about the "strings" package in Go because it provides tools for handling text data, splicing from basic strings to advanced regular expression matching. 1) The "strings" package provides efficient string operations, such as Join functions used to splice strings to avoid performance problems. 2) It contains advanced functions, such as the ContainsAny function, to check whether a string contains a specific character set. 3) The Replace function is used to replace substrings in a string, and attention should be paid to the replacement order and case sensitivity. 4) The Split function can split strings according to the separator and is often used for regular expression processing. 5) Performance needs to be considered when using, such as
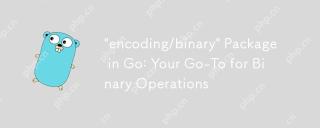
The"encoding/binary"packageinGoisessentialforhandlingbinarydata,offeringtoolsforreadingandwritingbinarydataefficiently.1)Itsupportsbothlittle-endianandbig-endianbyteorders,crucialforcross-systemcompatibility.2)Thepackageallowsworkingwithcus
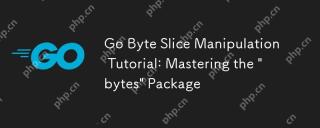
Mastering the bytes package in Go can help improve the efficiency and elegance of your code. 1) The bytes package is crucial for parsing binary data, processing network protocols, and memory management. 2) Use bytes.Buffer to gradually build byte slices. 3) The bytes package provides the functions of searching, replacing and segmenting byte slices. 4) The bytes.Reader type is suitable for reading data from byte slices, especially in I/O operations. 5) The bytes package works in collaboration with Go's garbage collector, improving the efficiency of big data processing.
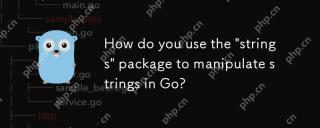
You can use the "strings" package in Go to manipulate strings. 1) Use strings.TrimSpace to remove whitespace characters at both ends of the string. 2) Use strings.Split to split the string into slices according to the specified delimiter. 3) Merge string slices into one string through strings.Join. 4) Use strings.Contains to check whether the string contains a specific substring. 5) Use strings.ReplaceAll to perform global replacement. Pay attention to performance and potential pitfalls when using it.
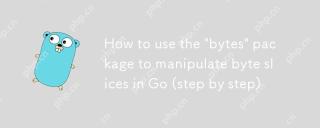
ThebytespackageinGoishighlyeffectiveforbyteslicemanipulation,offeringfunctionsforsearching,splitting,joining,andbuffering.1)Usebytes.Containstosearchforbytesequences.2)bytes.Splithelpsbreakdownbyteslicesusingdelimiters.3)bytes.Joinreconstructsbytesli
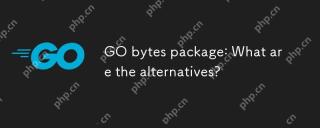
ThealternativestoGo'sbytespackageincludethestringspackage,bufiopackage,andcustomstructs.1)Thestringspackagecanbeusedforbytemanipulationbyconvertingbytestostringsandback.2)Thebufiopackageisidealforhandlinglargestreamsofbytedataefficiently.3)Customstru
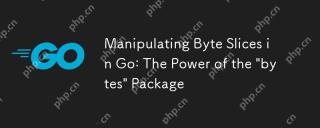
The"bytes"packageinGoisessentialforefficientlymanipulatingbyteslices,crucialforbinarydata,networkprotocols,andfileI/O.ItoffersfunctionslikeIndexforsearching,Bufferforhandlinglargedatasets,Readerforsimulatingstreamreading,andJoinforefficient
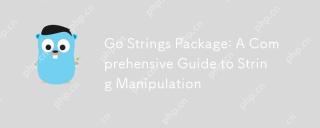
Go'sstringspackageiscrucialforefficientstringmanipulation,offeringtoolslikestrings.Split(),strings.Join(),strings.ReplaceAll(),andstrings.Contains().1)strings.Split()dividesastringintosubstrings;2)strings.Join()combinesslicesintoastring;3)strings.Rep


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

SublimeText3 Linux new version
SublimeText3 Linux latest version
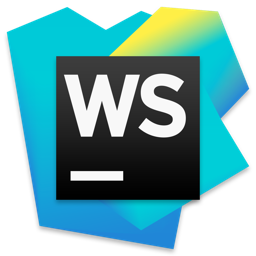
WebStorm Mac version
Useful JavaScript development tools
