Making Immutable Objects in Python
Creating immutable objects in Python can pose challenges. Simply overriding setattr is insufficient, as attributes cannot be set during initialization. A commonly employed solution is to subclass a tuple, as demonstrated below:
<code class="python">class Immutable(tuple): def __new__(cls, a, b): return tuple.__new__(cls, (a, b)) @property def a(self): return self[0] @property def b(self): return self[1] def __str__(self): return "<immutable>".format(self.a, self.b) def __setattr__(self, *ignored): raise NotImplementedError def __delattr__(self, *ignored): raise NotImplementedError</immutable></code>
However, this approach grants access to the a and b attributes through self[0] and self[1], which can be inconvenient.
To achieve pure Python immutability, another alternative exists:
<code class="python">Immutable = collections.namedtuple("Immutable", ["a", "b"])</code>
This method generates a type with the desired behavior, utilizing slots and inheriting from tuple. It offers the benefits of compatibility with pickle and copy, but still allows for accessing attributes via [0] and [1].
The above is the detailed content of How Can We Achieve True Immutability in Python?. For more information, please follow other related articles on the PHP Chinese website!
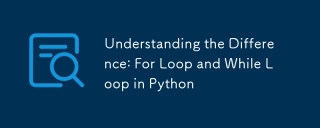
ThedifferencebetweenaforloopandawhileloopinPythonisthataforloopisusedwhenthenumberofiterationsisknowninadvance,whileawhileloopisusedwhenaconditionneedstobecheckedrepeatedlywithoutknowingthenumberofiterations.1)Forloopsareidealforiteratingoversequence
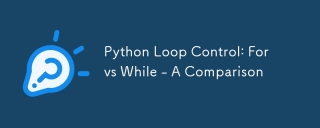
In Python, for loops are suitable for cases where the number of iterations is known, while loops are suitable for cases where the number of iterations is unknown and more control is required. 1) For loops are suitable for traversing sequences, such as lists, strings, etc., with concise and Pythonic code. 2) While loops are more appropriate when you need to control the loop according to conditions or wait for user input, but you need to pay attention to avoid infinite loops. 3) In terms of performance, the for loop is slightly faster, but the difference is usually not large. Choosing the right loop type can improve the efficiency and readability of your code.
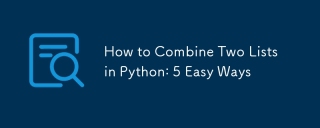
In Python, lists can be merged through five methods: 1) Use operators, which are simple and intuitive, suitable for small lists; 2) Use extend() method to directly modify the original list, suitable for lists that need to be updated frequently; 3) Use list analytical formulas, concise and operational on elements; 4) Use itertools.chain() function to efficient memory and suitable for large data sets; 5) Use * operators and zip() function to be suitable for scenes where elements need to be paired. Each method has its specific uses and advantages and disadvantages, and the project requirements and performance should be taken into account when choosing.
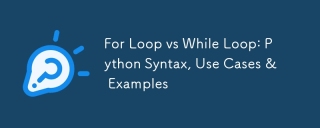
Forloopsareusedwhenthenumberofiterationsisknown,whilewhileloopsareuseduntilaconditionismet.1)Forloopsareidealforsequenceslikelists,usingsyntaxlike'forfruitinfruits:print(fruit)'.2)Whileloopsaresuitableforunknowniterationcounts,e.g.,'whilecountdown>
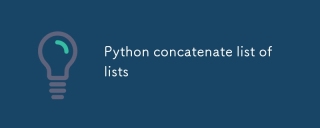
ToconcatenatealistoflistsinPython,useextend,listcomprehensions,itertools.chain,orrecursivefunctions.1)Extendmethodisstraightforwardbutverbose.2)Listcomprehensionsareconciseandefficientforlargerdatasets.3)Itertools.chainismemory-efficientforlargedatas
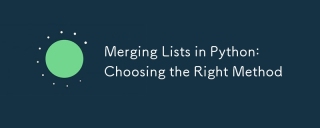
TomergelistsinPython,youcanusethe operator,extendmethod,listcomprehension,oritertools.chain,eachwithspecificadvantages:1)The operatorissimplebutlessefficientforlargelists;2)extendismemory-efficientbutmodifiestheoriginallist;3)listcomprehensionoffersf
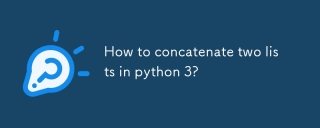
In Python 3, two lists can be connected through a variety of methods: 1) Use operator, which is suitable for small lists, but is inefficient for large lists; 2) Use extend method, which is suitable for large lists, with high memory efficiency, but will modify the original list; 3) Use * operator, which is suitable for merging multiple lists, without modifying the original list; 4) Use itertools.chain, which is suitable for large data sets, with high memory efficiency.
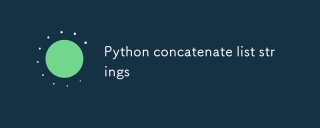
Using the join() method is the most efficient way to connect strings from lists in Python. 1) Use the join() method to be efficient and easy to read. 2) The cycle uses operators inefficiently for large lists. 3) The combination of list comprehension and join() is suitable for scenarios that require conversion. 4) The reduce() method is suitable for other types of reductions, but is inefficient for string concatenation. The complete sentence ends.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor
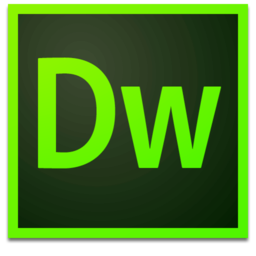
Dreamweaver Mac version
Visual web development tools

SublimeText3 Chinese version
Chinese version, very easy to use

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SublimeText3 English version
Recommended: Win version, supports code prompts!
