


Using Reflection to Modify Struct Fields: CanSet() and Structs
When using reflection to modify struct fields, it's important to understand the principles behind field accessibility and modification.
CanSet() for Structs
In your example, you encountered CanSet() returning false for struct fields. This is because by default, Go does not allow modifying non-exported (private) fields of a struct using reflection. This is a security measure to prevent accidental or malicious modification of internal struct state.
Addressing the Issues
To set the values of struct fields using reflection, consider the following steps:
- Modify a Value: When calling your SetField() function, pass the pointer to the struct, not the struct value itself. This allows you to modify the actual struct, not a copy.
- Use Value.Elem() for Pointers: If you pass a pointer to the struct, you need to use reflect.ValueOf(source).Elem() to obtain the reflect.Value of the pointed struct. This navigates to the actual struct value.
- Use FieldByName for Field Access: Instead of looping through all fields in the struct, use v.FieldByName(fieldName) to access the specific field you want to modify. This ensures you're accessing the correct field and is more efficient.
Modified Code
Here's the modified code that addresses the issues:
<code class="go">func SetField(source interface{}, fieldName string, fieldValue string) { v := reflect.ValueOf(source).Elem() fmt.Println(v.FieldByName(fieldName).CanSet()) if v.FieldByName(fieldName).CanSet() { v.FieldByName(fieldName).SetString(fieldValue) } } func main() { source := ProductionInfo{} source.StructA = append(source.StructA, Entry{Field1: "A", Field2: 2}) fmt.Println("Before: ", source.StructA[0]) SetField(&source.StructA[0], "Field1", "NEW_VALUE") fmt.Println("After: ", source.StructA[0]) }</code>
This code will now successfully modify the Field1 value of the Entry struct.
The above is the detailed content of How to Use Reflection to Modify Struct Fields with CanSet() and Structs?. For more information, please follow other related articles on the PHP Chinese website!
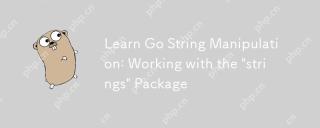
Go's "strings" package provides rich features to make string operation efficient and simple. 1) Use strings.Contains() to check substrings. 2) strings.Split() can be used to parse data, but it should be used with caution to avoid performance problems. 3) strings.Join() is suitable for formatting strings, but for small datasets, looping = is more efficient. 4) For large strings, it is more efficient to build strings using strings.Builder.
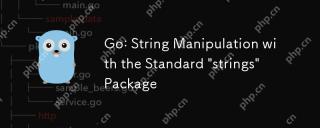
Go uses the "strings" package for string operations. 1) Use strings.Join function to splice strings. 2) Use the strings.Contains function to find substrings. 3) Use the strings.Replace function to replace strings. These functions are efficient and easy to use and are suitable for various string processing tasks.
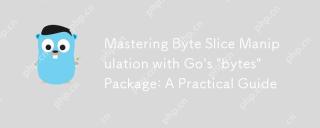
ThebytespackageinGoisessentialforefficientbyteslicemanipulation,offeringfunctionslikeContains,Index,andReplaceforsearchingandmodifyingbinarydata.Itenhancesperformanceandcodereadability,makingitavitaltoolforhandlingbinarydata,networkprotocols,andfileI
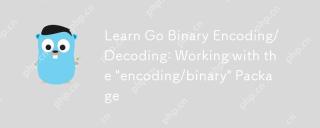
Go uses the "encoding/binary" package for binary encoding and decoding. 1) This package provides binary.Write and binary.Read functions for writing and reading data. 2) Pay attention to choosing the correct endian (such as BigEndian or LittleEndian). 3) Data alignment and error handling are also key to ensure the correctness and performance of the data.
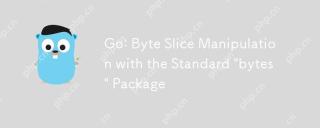
The"bytes"packageinGooffersefficientfunctionsformanipulatingbyteslices.1)Usebytes.Joinforconcatenatingslices,2)bytes.Bufferforincrementalwriting,3)bytes.Indexorbytes.IndexByteforsearching,4)bytes.Readerforreadinginchunks,and5)bytes.SplitNor
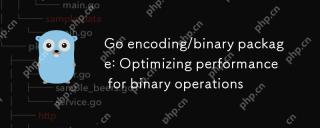
Theencoding/binarypackageinGoiseffectiveforoptimizingbinaryoperationsduetoitssupportforendiannessandefficientdatahandling.Toenhanceperformance:1)Usebinary.NativeEndianfornativeendiannesstoavoidbyteswapping.2)BatchReadandWriteoperationstoreduceI/Oover
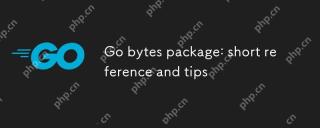
Go's bytes package is mainly used to efficiently process byte slices. 1) Using bytes.Buffer can efficiently perform string splicing to avoid unnecessary memory allocation. 2) The bytes.Equal function is used to quickly compare byte slices. 3) The bytes.Index, bytes.Split and bytes.ReplaceAll functions can be used to search and manipulate byte slices, but performance issues need to be paid attention to.
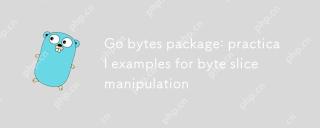
The byte package provides a variety of functions to efficiently process byte slices. 1) Use bytes.Contains to check the byte sequence. 2) Use bytes.Split to split byte slices. 3) Replace the byte sequence bytes.Replace. 4) Use bytes.Join to connect multiple byte slices. 5) Use bytes.Buffer to build data. 6) Combined bytes.Map for error processing and data verification.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
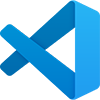
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Atom editor mac version download
The most popular open source editor

SublimeText3 English version
Recommended: Win version, supports code prompts!

Notepad++7.3.1
Easy-to-use and free code editor
