


How to Override JLabel Mouse Events to Prevent Event Consumption During Drag and Drop?
JLabel Mouse Events for Drag and Drop
Drag and drop functionality can be implemented over a JLabel by overriding its mouse events. However, an issue arises when defining drag and drop in the mousePressed event, as it prevents the mouseReleased event from triggering on the same JLabel.
Implementation:
<code class="java">Thumbnails[I_Loop].setText("1"); Thumbnails[I_Loop].setTransferHandler(new TransferHandler("text")); Thumbnails[I_Loop].addMouseListener(new MouseAdapter() { public void mouseReleased(MouseEvent me) { System.out.println("here mouse released"); } public void mousePressed(MouseEvent me) { System.out.println("here mouse pressed"); JComponent comp = (JComponent) me.getSource(); TransferHandler handler = comp.getTransferHandler(); handler.exportAsDrag(comp, me, TransferHandler.COPY); } });</code>
In this example, the mousePressed event is used to initiate the drag and drop process, but it consumes the mouse event and prevents the mouseReleased event from being triggered. As a result, the "here mouse released" message is not printed.
Alternative Approaches:
1. Using TransferHandler:
As @Thomas mentioned, TransferHandler can be used for drag and drop operations. It provides methods such as exportAsDrag() to initiate the dragging process.
2. Using MouseMotionListener:
This approach listens for mouse drag events to move the component rather than relying on mouse pressed and released events.
3. Using JLayeredPane:
Components can be dragged over a JLayeredPane using mouse events. Refer to the linked Stack Overflow examples for more details.
Improved Code:
<code class="java">Thumbnails[I_Loop].setTransferHandler(new TransferHandler("text")); Thumbnails[I_Loop].addMouseListener(new MouseAdapter() { public void mouseReleased(MouseEvent me) { System.out.println("here mouse released"); } }); Thumbnails[I_Loop].addMouseMotionListener(new MouseMotionAdapter() { @Override public void mouseDragged(MouseEvent e) { int dx = e.getX() - mousePt.x; int dy = e.getY() - mousePt.y; Thumbnails[I_Loop].setBounds(Thumbnails[I_Loop].getX() + dx, Thumbnails[I_Loop].getY() + dy, Thumbnails[I_Loop].getWidth(), Thumbnails[I_Loop].getHeight()); } });</code>
This implementation uses MouseMotionListener to handle mouse drag events and update the JLabel's position accordingly. The mouseReleased event is now triggered and the "here mouse released" message is printed.
The above is the detailed content of How to Override JLabel Mouse Events to Prevent Event Consumption During Drag and Drop?. For more information, please follow other related articles on the PHP Chinese website!
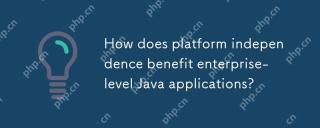
Java is widely used in enterprise-level applications because of its platform independence. 1) Platform independence is implemented through Java virtual machine (JVM), so that the code can run on any platform that supports Java. 2) It simplifies cross-platform deployment and development processes, providing greater flexibility and scalability. 3) However, it is necessary to pay attention to performance differences and third-party library compatibility and adopt best practices such as using pure Java code and cross-platform testing.
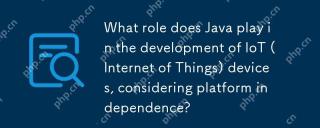
JavaplaysasignificantroleinIoTduetoitsplatformindependence.1)Itallowscodetobewrittenonceandrunonvariousdevices.2)Java'secosystemprovidesusefullibrariesforIoT.3)ItssecurityfeaturesenhanceIoTsystemsafety.However,developersmustaddressmemoryandstartuptim
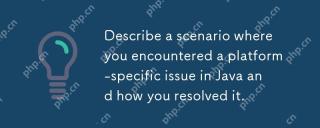
ThesolutiontohandlefilepathsacrossWindowsandLinuxinJavaistousePaths.get()fromthejava.nio.filepackage.1)UsePaths.get()withSystem.getProperty("user.dir")andtherelativepathtoconstructthefilepath.2)ConverttheresultingPathobjecttoaFileobjectifne
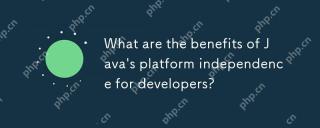
Java'splatformindependenceissignificantbecauseitallowsdeveloperstowritecodeonceandrunitonanyplatformwithaJVM.This"writeonce,runanywhere"(WORA)approachoffers:1)Cross-platformcompatibility,enablingdeploymentacrossdifferentOSwithoutissues;2)Re
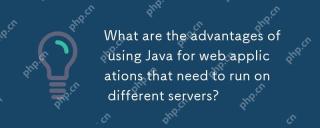
Java is suitable for developing cross-server web applications. 1) Java's "write once, run everywhere" philosophy makes its code run on any platform that supports JVM. 2) Java has a rich ecosystem, including tools such as Spring and Hibernate, to simplify the development process. 3) Java performs excellently in performance and security, providing efficient memory management and strong security guarantees.
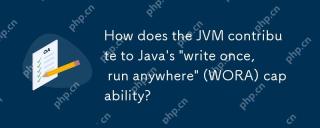
JVM implements the WORA features of Java through bytecode interpretation, platform-independent APIs and dynamic class loading: 1. Bytecode is interpreted as machine code to ensure cross-platform operation; 2. Standard API abstract operating system differences; 3. Classes are loaded dynamically at runtime to ensure consistency.
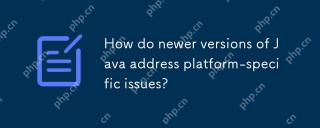
The latest version of Java effectively solves platform-specific problems through JVM optimization, standard library improvements and third-party library support. 1) JVM optimization, such as Java11's ZGC improves garbage collection performance. 2) Standard library improvements, such as Java9's module system reducing platform-related problems. 3) Third-party libraries provide platform-optimized versions, such as OpenCV.
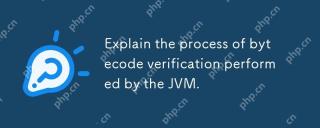
The JVM's bytecode verification process includes four key steps: 1) Check whether the class file format complies with the specifications, 2) Verify the validity and correctness of the bytecode instructions, 3) Perform data flow analysis to ensure type safety, and 4) Balancing the thoroughness and performance of verification. Through these steps, the JVM ensures that only secure, correct bytecode is executed, thereby protecting the integrity and security of the program.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
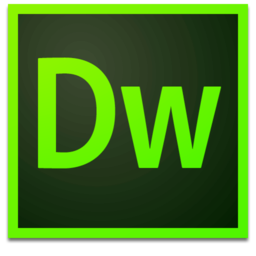
Dreamweaver Mac version
Visual web development tools

Atom editor mac version download
The most popular open source editor
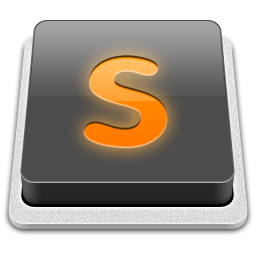
SublimeText3 Mac version
God-level code editing software (SublimeText3)
