Bypassing Swagger UI for JSON Data Input in FastAPI
When working with FastAPI, it's possible to post JSON data without the intermediary of the Swagger UI. Here's how to achieve this:
JavaScript Interface for JSON Data Submission
Employ a JavaScript-based interface like the Fetch API to send data in JSON format. Here's an example:
<code class="javascript">var data = { name: "foo", roll: 1 } fetch('/', { method: 'POST', headers: { 'Accept': 'application/json', 'Content-Type': 'application/json' }, body: JSON.stringify(data) }).then(resp => { return resp.text(); }).then(data => { // Handle the response });</code>
HTML Form and Jinja2 Templates
Alternatively, you can utilize Jinja2 Templates and an HTML form to submit your data. Here's how:
app.py
<code class="python">from fastapi import FastAPI, Request from fastapi.templating import Jinja2Templates from pydantic import BaseModel app = FastAPI() templates = Jinja2Templates(directory="templates") class Item(BaseModel): name: str roll: int @app.post("/") async def create_item(item: Item): return item @app.get("/") async def index(request: Request): return templates.TemplateResponse("index.html", {"request": request})</code>
index.html
<code class="html"><form method="post"> <input type="text" name="name" value="foo"> <input type="number" name="roll" value="1"> <input type="submit" value="Submit"> </form> <div id="responseArea"></div> <script> document.querySelector('form').addEventListener('submit', (event) => { event.preventDefault(); var data = new FormData(event.target); fetch('/', { method: 'POST', headers: { 'Accept': 'application/json', 'Content-Type': 'application/json' }, body: JSON.stringify(Object.fromEntries(data)) }).then(resp => { return resp.text(); }).then(data => { document.getElementById("responseArea").innerHTML = data; }).catch(error => { console.error(error); }); }); </script></code>
By using these techniques, you can conveniently post JSON data to your FastAPI backend without relying on the Swagger UI interface.
The above is the detailed content of How to Submit JSON Data to FastAPI without Swagger UI?. For more information, please follow other related articles on the PHP Chinese website!
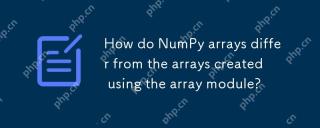
NumPyarraysarebetterfornumericaloperationsandmulti-dimensionaldata,whilethearraymoduleissuitableforbasic,memory-efficientarrays.1)NumPyexcelsinperformanceandfunctionalityforlargedatasetsandcomplexoperations.2)Thearraymoduleismorememory-efficientandfa
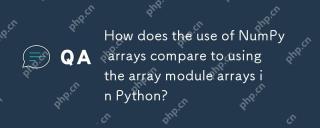
NumPyarraysarebetterforheavynumericalcomputing,whilethearraymoduleismoresuitableformemory-constrainedprojectswithsimpledatatypes.1)NumPyarraysofferversatilityandperformanceforlargedatasetsandcomplexoperations.2)Thearraymoduleislightweightandmemory-ef
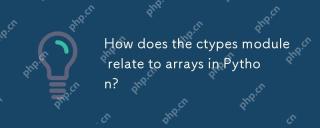
ctypesallowscreatingandmanipulatingC-stylearraysinPython.1)UsectypestointerfacewithClibrariesforperformance.2)CreateC-stylearraysfornumericalcomputations.3)PassarraystoCfunctionsforefficientoperations.However,becautiousofmemorymanagement,performanceo
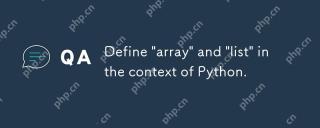
InPython,a"list"isaversatile,mutablesequencethatcanholdmixeddatatypes,whilean"array"isamorememory-efficient,homogeneoussequencerequiringelementsofthesametype.1)Listsareidealfordiversedatastorageandmanipulationduetotheirflexibility
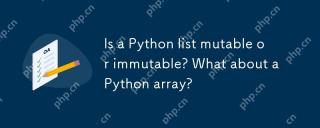
Pythonlistsandarraysarebothmutable.1)Listsareflexibleandsupportheterogeneousdatabutarelessmemory-efficient.2)Arraysaremorememory-efficientforhomogeneousdatabutlessversatile,requiringcorrecttypecodeusagetoavoiderrors.
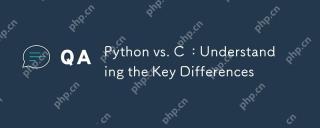
Python and C each have their own advantages, and the choice should be based on project requirements. 1) Python is suitable for rapid development and data processing due to its concise syntax and dynamic typing. 2)C is suitable for high performance and system programming due to its static typing and manual memory management.
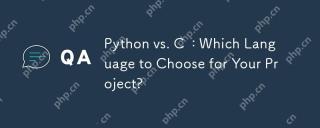
Choosing Python or C depends on project requirements: 1) If you need rapid development, data processing and prototype design, choose Python; 2) If you need high performance, low latency and close hardware control, choose C.
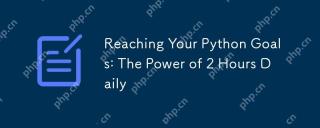
By investing 2 hours of Python learning every day, you can effectively improve your programming skills. 1. Learn new knowledge: read documents or watch tutorials. 2. Practice: Write code and complete exercises. 3. Review: Consolidate the content you have learned. 4. Project practice: Apply what you have learned in actual projects. Such a structured learning plan can help you systematically master Python and achieve career goals.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

Atom editor mac version download
The most popular open source editor
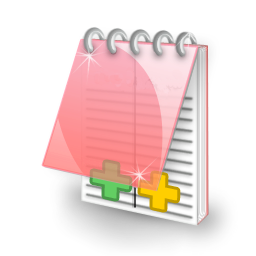
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
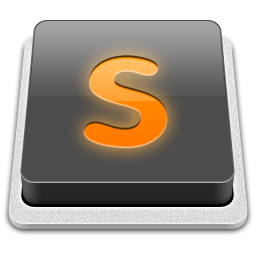
SublimeText3 Mac version
God-level code editing software (SublimeText3)
