Sleep Functionality in C
In C , developers often encounter the need to pause program execution for a specific duration, similar to the Sleep() function in other programming languages. This article aims to address that requirement and provide comprehensive solutions.
std::this_thread::sleep_for
C 11 introduced the
<code class="cpp">#include <chrono> #include <thread> std::chrono::milliseconds timespan(111605); // or whatever std::this_thread::sleep_for(timespan);</thread></chrono></code>
std::this_thread::sleep_until
An alternative to std::this_thread::sleep_for is std::this_thread::sleep_until, which allows pausing until a specific point in time is reached.
Pre-C 11 Solutions
Before C 11, C lacked thread capabilities and sleep functionality. As such, platform-dependent solutions were necessary. Here's an example that works for both Windows and Unix:
<code class="cpp">#ifdef _WIN32 #include <windows.h> void sleep(unsigned milliseconds) { Sleep(milliseconds); } #else #include <unistd.h> void sleep(unsigned milliseconds) { usleep(milliseconds * 1000); // takes microseconds } #endif</unistd.h></windows.h></code>
Alternatively, boost::this_thread::sleep can be used as a simpler pre-C 11 approach.
The above is the detailed content of How to Implement Sleep Functionality in C ?. For more information, please follow other related articles on the PHP Chinese website!
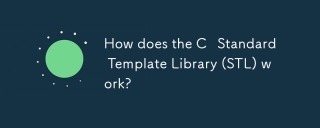
This article explains the C Standard Template Library (STL), focusing on its core components: containers, iterators, algorithms, and functors. It details how these interact to enable generic programming, improving code efficiency and readability t
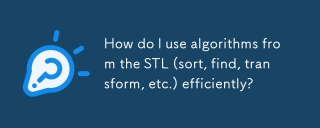
This article details efficient STL algorithm usage in C . It emphasizes data structure choice (vectors vs. lists), algorithm complexity analysis (e.g., std::sort vs. std::partial_sort), iterator usage, and parallel execution. Common pitfalls like
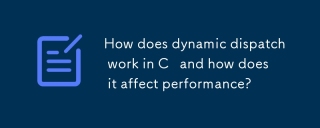
The article discusses dynamic dispatch in C , its performance costs, and optimization strategies. It highlights scenarios where dynamic dispatch impacts performance and compares it with static dispatch, emphasizing trade-offs between performance and
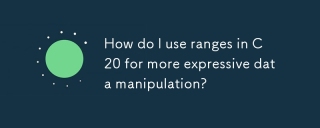
C 20 ranges enhance data manipulation with expressiveness, composability, and efficiency. They simplify complex transformations and integrate into existing codebases for better performance and maintainability.
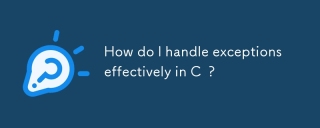
This article details effective exception handling in C , covering try, catch, and throw mechanics. It emphasizes best practices like RAII, avoiding unnecessary catch blocks, and logging exceptions for robust code. The article also addresses perf
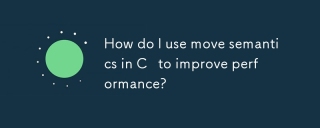
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
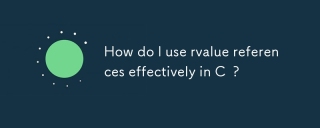
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)
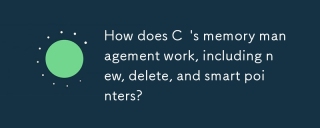
C memory management uses new, delete, and smart pointers. The article discusses manual vs. automated management and how smart pointers prevent memory leaks.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version
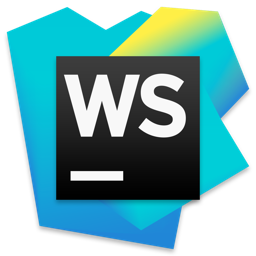
WebStorm Mac version
Useful JavaScript development tools
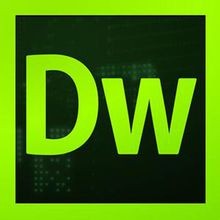
Dreamweaver CS6
Visual web development tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SublimeText3 Chinese version
Chinese version, very easy to use
