Using Swing Timer and SwingWorker to Update JLabel Continuously
In order to regularly update a JLabel with the results of a time-consuming activity, it is recommended to employ both a Swing Timer and a SwingWorker. The Swing Timer handles recurring tasks, while the SwingWorker manages time-consuming undertakings. Here's a sample code that illustrates how this strategy functions:
<code class="java">import java.awt.event.*; import javax.swing.*; import java.net.Socket; public class LabelUpdateUsingTimer { static String hostnameOrIP = "stackoverflow.com"; int delay = 5000; JLabel label = new JLabel("0000"); LabelUpdateUsingTimer() { label.setFont(label.getFont().deriveFont(120f)); ActionListener timerListener = new ActionListener() { @Override public void actionPerformed(ActionEvent e) { new PingWorker().execute(); } }; Timer timer = new Timer(delay, timerListener); timer.start(); JOptionPane.showMessageDialog( null, label, hostnameOrIP, JOptionPane.INFORMATION_MESSAGE); timer.stop(); } class PingWorker extends SwingWorker { int time; @Override protected Object doInBackground() throws Exception { time = pingTime(); return new Integer(time); } @Override protected void done() { label.setText("" + time); } }; public static int pingTime() { Socket socket = null; long start = System.currentTimeMillis(); try { socket = new Socket(hostnameOrIP, 80); } catch (Exception weTried) { } finally { if (socket != null) { try { socket.close(); } catch (Exception weTried) {} } } long end = System.currentTimeMillis(); return (int) (end - start); } public static void main(String[] args) { Runnable r = new Runnable() { @Override public void run() { new LabelUpdateUsingTimer(); } }; SwingUtilities.invokeLater(r); } }</code>
For more details regarding the Event Dispatch Thread and executing repetitive or extended tasks in a GUI, please consult the Concurrency in Swing document.
How the Code Works:
- Initialization: The LabelUpdateUsingTimer constructor initializes a JLabel named label and sets its font.
- ActionListener and Timer: An ActionListener called timerListener is created, and it will trigger the execution of the PingWorker every delay milliseconds. A Timer object is then initiated using the delay and timerListener as arguments, and it is subsequently started.
- JOptionPane: The JLabel is displayed within an JOptionPane dialog box with the specified hostnameOrIP.
- PingWorker: The PingWorker class, a SwingWorker subclass, performs the time-consuming operation in a background thread.
- Ping Time: The pingTime method establishes a TCP connection to the specified hostnameOrIP and calculates the latency.
By employing this technique, you can continuously update a JLabel with the latest results of a time-consuming task, enabling real-time monitoring and user interaction.
The above is the detailed content of How to Continuously Update a JLabel Using Swing Timer and SwingWorker?. For more information, please follow other related articles on the PHP Chinese website!
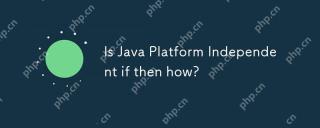
Java is platform-independent because of its "write once, run everywhere" design philosophy, which relies on Java virtual machines (JVMs) and bytecode. 1) Java code is compiled into bytecode, interpreted by the JVM or compiled on the fly locally. 2) Pay attention to library dependencies, performance differences and environment configuration. 3) Using standard libraries, cross-platform testing and version management is the best practice to ensure platform independence.
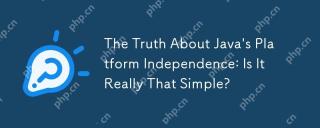
Java'splatformindependenceisnotsimple;itinvolvescomplexities.1)JVMcompatibilitymustbeensuredacrossplatforms.2)Nativelibrariesandsystemcallsneedcarefulhandling.3)Dependenciesandlibrariesrequirecross-platformcompatibility.4)Performanceoptimizationacros
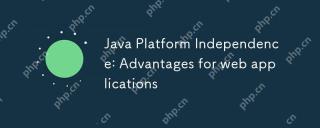
Java'splatformindependencebenefitswebapplicationsbyallowingcodetorunonanysystemwithaJVM,simplifyingdeploymentandscaling.Itenables:1)easydeploymentacrossdifferentservers,2)seamlessscalingacrosscloudplatforms,and3)consistentdevelopmenttodeploymentproce
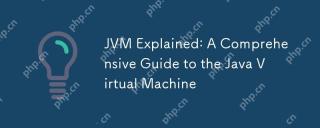
TheJVMistheruntimeenvironmentforexecutingJavabytecode,crucialforJava's"writeonce,runanywhere"capability.Itmanagesmemory,executesthreads,andensuressecurity,makingitessentialforJavadeveloperstounderstandforefficientandrobustapplicationdevelop
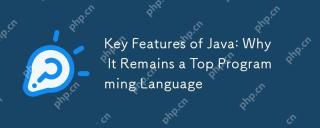
Javaremainsatopchoicefordevelopersduetoitsplatformindependence,object-orienteddesign,strongtyping,automaticmemorymanagement,andcomprehensivestandardlibrary.ThesefeaturesmakeJavaversatileandpowerful,suitableforawiderangeofapplications,despitesomechall
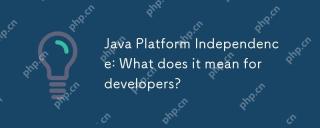
Java'splatformindependencemeansdeveloperscanwritecodeonceandrunitonanydevicewithoutrecompiling.ThisisachievedthroughtheJavaVirtualMachine(JVM),whichtranslatesbytecodeintomachine-specificinstructions,allowinguniversalcompatibilityacrossplatforms.Howev
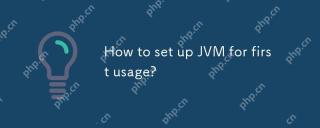
To set up the JVM, you need to follow the following steps: 1) Download and install the JDK, 2) Set environment variables, 3) Verify the installation, 4) Set the IDE, 5) Test the runner program. Setting up a JVM is not just about making it work, it also involves optimizing memory allocation, garbage collection, performance tuning, and error handling to ensure optimal operation.
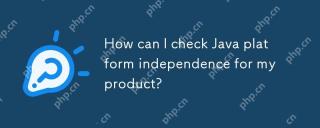
ToensureJavaplatformindependence,followthesesteps:1)CompileandrunyourapplicationonmultipleplatformsusingdifferentOSandJVMversions.2)UtilizeCI/CDpipelineslikeJenkinsorGitHubActionsforautomatedcross-platformtesting.3)Usecross-platformtestingframeworkss


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!
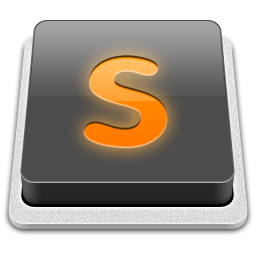
SublimeText3 Mac version
God-level code editing software (SublimeText3)

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
