CSS Property Extraction with JavaScript
Accessing CSS properties of HTML elements through JavaScript is an essential skill for dynamic web development. Consider the scenario where an external stylesheet, such as "style.css," is linked to a webpage. To manipulate specific CSS properties, it is necessary to retrieve their values using JavaScript.
Options for Reading CSS Properties
There are two primary methods to achieve this:
- Direct Style Object Access: In modern browsers, accessing the document.styleSheets object provides a comprehensive list of all style rules applied to the document. By parsing this object, it is possible to locate and extract individual properties. However, this method is not recommended due to its complexity and limitations.
- GetComputedStyle Method: A more versatile approach involves creating an element with the desired style configuration, using the createElement() method. By applying the getComputedStyle() or currentStyle (IE) method to this element, the specific CSS property value can be obtained.
Example: Extracting the Color Property
Consider the following code snippet:
<code class="javascript">function getStyleProp(elem, prop) { if (window.getComputedStyle) { return window.getComputedStyle(elem, null).getPropertyValue(prop); } else if (elem.currentStyle) { return elem.currentStyle[prop]; // IE } } window.onload = function () { var d = document.createElement("div"); // Create div d.className = "layout"; // Set class = "layout" alert(getStyleProp(d, "color")); // Get property value };</code>
This function takes two parameters: the targeted element and the desired CSS property (e.g., "color"). By creating a temporary div element with the same styling as the target element, the code extracts and returns the specified property value.
Advanced Techniques
To retrieve style properties that exclude inline styles, the getNonInlineStyle() function can be employed:
<code class="javascript">function getNonInlineStyle(elem, prop) { var style = elem.cssText; // Cache the inline style elem.cssText = ""; // Remove all inline styles var inheritedPropValue = getStyle(elem, prop); // Get inherited value elem.cssText = style; // Add the inline style back return inheritedPropValue; }</code>
By temporarily erasing inline styles, this function reveals the inherited property values from the stylesheet.
The above is the detailed content of How Can JavaScript Access CSS Properties of HTML Elements?. For more information, please follow other related articles on the PHP Chinese website!
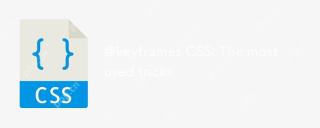
@keyframesispopularduetoitsversatilityandpowerincreatingsmoothCSSanimations.Keytricksinclude:1)Definingsmoothtransitionsbetweenstates,2)Animatingmultiplepropertiessimultaneously,3)Usingvendorprefixesforbrowsercompatibility,4)CombiningwithJavaScriptfo
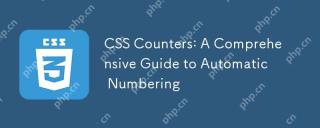
CSSCountersareusedtomanageautomaticnumberinginwebdesigns.1)Theycanbeusedfortablesofcontents,listitems,andcustomnumbering.2)Advancedusesincludenestednumberingsystems.3)Challengesincludebrowsercompatibilityandperformanceissues.4)Creativeusesinvolvecust
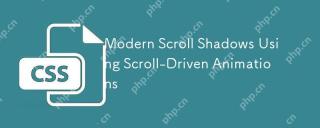
Using scroll shadows, especially for mobile devices, is a subtle bit of UX that Chris has covered before. Geoff covered a newer approach that uses the animation-timeline property. Here’s yet another way.
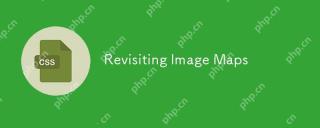
Let’s run through a quick refresher. Image maps date all the way back to HTML 3.2, where, first, server-side maps and then client-side maps defined clickable regions over an image using map and area elements.
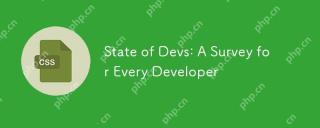
The State of Devs survey is now open to participation, and unlike previous surveys it covers everything except code: career, workplace, but also health, hobbies, and more.
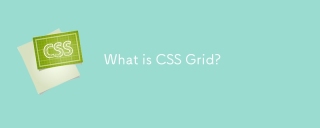
CSS Grid is a powerful tool for creating complex, responsive web layouts. It simplifies design, improves accessibility, and offers more control than older methods.
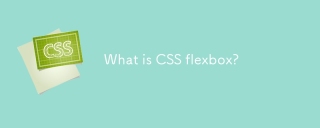
Article discusses CSS Flexbox, a layout method for efficient alignment and distribution of space in responsive designs. It explains Flexbox usage, compares it with CSS Grid, and details browser support.
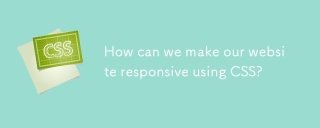
The article discusses techniques for creating responsive websites using CSS, including viewport meta tags, flexible grids, fluid media, media queries, and relative units. It also covers using CSS Grid and Flexbox together and recommends CSS framework


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
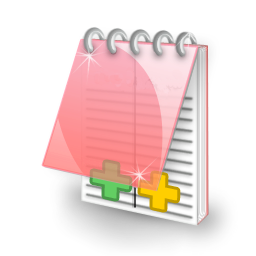
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

SublimeText3 English version
Recommended: Win version, supports code prompts!

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
