The fundamentals of operators in programming are essential for performing mathematical operations, logical comparisons, data manipulation and flow control within a program. Let's learn them using JavaScript?
Main types of operators in JavaScript:
1. Arithmetic Operators
They are used to perform mathematical operations between numbers. These operators include:
- Addition ( ): Adds two values.
- Subtraction (-): Subtracts the second value from the first.
- Multiplication (*): Multiplies two values.
- Division (/): Divides the first value by the second.
- Module (%): Returns the remainder of the division between two values.
- Exponentiation (``)**: Raises the first value to the power of the second.
Example:
let a = 10; let b = 3; console.log(a + b); // Adição: 13 console.log(a - b); // Subtração: 7 console.log(a * b); // Multiplicação: 30 console.log(a / b); // Divisão: 3.333 console.log(a % b); // Módulo: 1 (resto da divisão de 10 por 3) console.log(a ** b); // Exponenciação: 1000 (10 elevado a 3)
2. Assignment Operators
Assignment operators are used to assign values to variables. The most common operator is "=", but there are combinations with arithmetic operators that make the code easier.
- Assignment (=): Assigns a value to the variable.
- Assignment with addition ( =): Adds and assigns the result to the variable.
- Assignment with subtraction (-=): Subtracts and assigns the result to the variable.
- Assignment with multiplication (*=): Multiplies and assigns the result to the variable.
- Assignment with division (/=): Divides and assigns the result to the variable.
Example:
let x = 5; x += 3; // x = x + 3 -> 8 x -= 2; // x = x - 2 -> 6 x *= 4; // x = x * 4 -> 24 x /= 2; // x = x / 2 -> 12 console.log(x); // Resultado final: 12
3. Comparison Operators
These operators compare two values and return a boolean value (true or false). They are widely used in control structures, such as if, and while.
- Equality (==): Checks if the values are equal, without checking the type.
- Identity (===): Checks if the values and types are exactly the same.
- Different (!=): Checks if the values are different.
- Strict difference (!==): Checks if values and types are different.
- Greater than (>): Checks if the value on the left is greater.
- Less than (: Checks if the value on the left is smaller.
- Greater or equal (>=): Checks if the value on the left is greater or equal.
- Less than or equal (: Checks if the value on the left is less than or equal.
Example:
let a = 10; let b = 3; console.log(a + b); // Adição: 13 console.log(a - b); // Subtração: 7 console.log(a * b); // Multiplicação: 30 console.log(a / b); // Divisão: 3.333 console.log(a % b); // Módulo: 1 (resto da divisão de 10 por 3) console.log(a ** b); // Exponenciação: 1000 (10 elevado a 3)
4. Logical Operators
Logical operators are used to combine Boolean expressions (true or false) and are essential for flow control.
- AND ( && ): Returns true if both expressions are true.
- OR ( || ): Returns true if at least one of the expressions is true.
- NOT ( ! ): Inverts the Boolean value of an expression.
Example:
let x = 5; x += 3; // x = x + 3 -> 8 x -= 2; // x = x - 2 -> 6 x *= 4; // x = x * 4 -> 24 x /= 2; // x = x / 2 -> 12 console.log(x); // Resultado final: 12
5. Unary Operators
These operators work with just one operand and can modify or return the value of a variable.
- Increment ( ): Adds 1 to the variable value.
- Decrement (--): Subtract 1 from the variable value.
Example:
let num1 = 10; let num2 = '10'; console.log(num1 == num2); // true (só compara o valor) console.log(num1 === num2); // false (compara valor e tipo) console.log(num1 != num2); // false (valores são iguais) console.log(num1 !== num2); // true (tipos são diferentes) console.log(num1 > 5); // true console.log(num1 <p>It is important to remember that the order of these operators influences the behavior of the variable. There are two ways to use them:</p>
- Prefixed x or --x: Use the current value of the variable in the expression and then increment/decrement it.
- Posfixed x or x--: Increments/decrements the value before using it in the expression (as exemplified previously).
Find out more by clicking here
6. Ternary Operators
The ternary operator is a simplified form of an if to assign values based on a condition. Is your structure a condition? value_if_true : value_if_false.
Example:
let a = true; let b = false; console.log(a && b); // false (AND: ambos devem ser verdadeiros) console.log(a || b); // true (OR: ao menos um deve ser verdadeiro) console.log(!a); // false (NOT: inverte o valor de 'a')
Learn more about Ternary Operators here
7. String Concatenate Operator ( )
The addition operator ( ) can also be used to concatenate strings (join texts).
Example:
let a = 10; let b = 3; console.log(a + b); // Adição: 13 console.log(a - b); // Subtração: 7 console.log(a * b); // Multiplicação: 30 console.log(a / b); // Divisão: 3.333 console.log(a % b); // Módulo: 1 (resto da divisão de 10 por 3) console.log(a ** b); // Exponenciação: 1000 (10 elevado a 3)
8. Bitwise Operators (Bitwise)
These operators perform bit-level operations (0s and 1s), generally used in low-level programming, such as hardware operations. It is not common to use these types of operators.
- AND Bit by Bit (&)
- OR Bit by Bit (|)
- XOR Bit by Bit (^)
- NOT Bit by Bit (~)
- Left shift (
- Right shift (>>)
Example:
let x = 5; x += 3; // x = x + 3 -> 8 x -= 2; // x = x - 2 -> 6 x *= 4; // x = x * 4 -> 24 x /= 2; // x = x / 2 -> 12 console.log(x); // Resultado final: 12
Understanding how Operators work is fundamental to building programs that perform calculations, comparisons and control the flow of code efficiently.
The above is the detailed content of Operator Fundamentals. For more information, please follow other related articles on the PHP Chinese website!
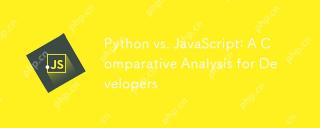
The main difference between Python and JavaScript is the type system and application scenarios. 1. Python uses dynamic types, suitable for scientific computing and data analysis. 2. JavaScript adopts weak types and is widely used in front-end and full-stack development. The two have their own advantages in asynchronous programming and performance optimization, and should be decided according to project requirements when choosing.
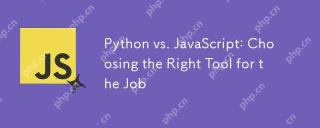
Whether to choose Python or JavaScript depends on the project type: 1) Choose Python for data science and automation tasks; 2) Choose JavaScript for front-end and full-stack development. Python is favored for its powerful library in data processing and automation, while JavaScript is indispensable for its advantages in web interaction and full-stack development.
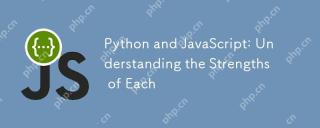
Python and JavaScript each have their own advantages, and the choice depends on project needs and personal preferences. 1. Python is easy to learn, with concise syntax, suitable for data science and back-end development, but has a slow execution speed. 2. JavaScript is everywhere in front-end development and has strong asynchronous programming capabilities. Node.js makes it suitable for full-stack development, but the syntax may be complex and error-prone.
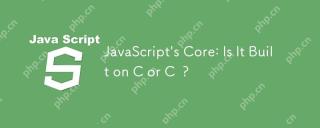
JavaScriptisnotbuiltonCorC ;it'saninterpretedlanguagethatrunsonenginesoftenwritteninC .1)JavaScriptwasdesignedasalightweight,interpretedlanguageforwebbrowsers.2)EnginesevolvedfromsimpleinterpreterstoJITcompilers,typicallyinC ,improvingperformance.
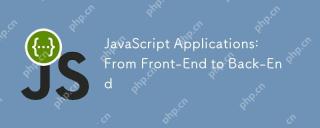
JavaScript can be used for front-end and back-end development. The front-end enhances the user experience through DOM operations, and the back-end handles server tasks through Node.js. 1. Front-end example: Change the content of the web page text. 2. Backend example: Create a Node.js server.
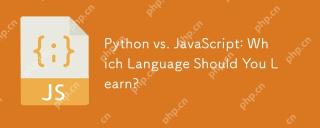
Choosing Python or JavaScript should be based on career development, learning curve and ecosystem: 1) Career development: Python is suitable for data science and back-end development, while JavaScript is suitable for front-end and full-stack development. 2) Learning curve: Python syntax is concise and suitable for beginners; JavaScript syntax is flexible. 3) Ecosystem: Python has rich scientific computing libraries, and JavaScript has a powerful front-end framework.
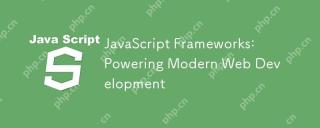
The power of the JavaScript framework lies in simplifying development, improving user experience and application performance. When choosing a framework, consider: 1. Project size and complexity, 2. Team experience, 3. Ecosystem and community support.
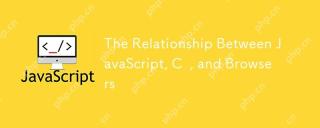
Introduction I know you may find it strange, what exactly does JavaScript, C and browser have to do? They seem to be unrelated, but in fact, they play a very important role in modern web development. Today we will discuss the close connection between these three. Through this article, you will learn how JavaScript runs in the browser, the role of C in the browser engine, and how they work together to drive rendering and interaction of web pages. We all know the relationship between JavaScript and browser. JavaScript is the core language of front-end development. It runs directly in the browser, making web pages vivid and interesting. Have you ever wondered why JavaScr


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

Atom editor mac version download
The most popular open source editor
