React, like any other JavaScript framework, handles a lot of things behind the scenes that we often don’t even think about.
And that’s okay—our job as developers is to solve problems, and the simpler the implementation, the better. You don’t always need to understand every detail of what a framework does for you.
JavaScript is an interesting language; it’s the king of the browser, and browsers are still used heavily, so I don’t see it disappearing anytime soon.
In fact, many native apps (iOS, Android, Smart TVs) run hybrid solutions using both native and web technologies.
In this post, I want to test a simple counter in React versus its Vanilla JavaScript version.
Measuring Performance with Chrome DevTools
First, let's talk about a useful tab that Chrome offers called Performance. This tab includes a recording feature that measures a web application's performance.
In this post, I'm going to focus on three key metrics: JS Heap, Nodes, and Listeners.
JS Heap: The heap is a region of memory in JavaScript where objects, arrays, and functions are stored. Unlike the stack, which holds primitive values (numbers, strings, booleans) and function calls, the heap manages dynamic memory allocation.
DOM Nodes: A DOM node is an individual element, attribute, or text within a web page's HTML, represented in the Document Object Model (DOM).
Event Listeners: In JavaScript, event listeners are functions that wait for specific events (e.g., clicks, key presses, mouse movements) on HTML elements. When the event occurs, the listener triggers, executing code in response.
Demo: Building a Basic React Counter
Alright, the UI is a simple counter. The UI is just a button with a click handler. Every time the button is clicked, the counter increments.
- React Demo
The React code looks like this:
"use client"; import { useState } from "react"; export default function Page() { const [counter, setCounter] = useState(0); const incrementClickHandler = (event: { preventDefault: () => void }) => { event.preventDefault(); setCounter((prevCounter) => prevCounter + 1); }; return ( <div style="{{" maxwidth: margin: auto> <a href="#" style="{{" display: padding: fontsize: border: solid black width: textalign: margintop: onclick="{incrementClickHandler}"> Increment {counter} </a> </div> ); }
The code is pretty self-explanatory. One thing to note is that the demo runs on top of Next.js, which is why we need "use client". Other than that, it's just a basic React component.
React Counter UI
20 Seconds and Only One Click
Now, I'm going to open the Performance tab in Chrome, click the record icon, and let it run for 20 seconds while clicking the button only once. At the end of the 20 seconds, the performance results look like this:
See how just one click bumps the numbers to:
React | |
---|---|
JS Heap | 3.4MB |
Nodes | 47 |
Listeners | 287 |
?
20 Seconds with a Click per Second
Now, I’m going to let it run for another 20 seconds, but this time I’ll click the button once per second. Let’s take a look at the results:
React | |
---|---|
JS Heap | 4MB |
Nodes | 46 |
Listeners | 331 |
Two things to note about React:
a) When a state variable is updated, the component is re-rendered, meaning that in this case, the component was rendered 20 times.
b) Thanks to the virtual DOM, React only updates the nodes that need to be updated.
Now, let’s go back to the chart and see how the blue line (JS Heap) and the yellow line (Listeners) increment, while the green line (Nodes) remains constant.
It's also worth mentioning that the numbers didn't change much compared to the one-click run.
Demo: Building a Vanilla JavaScript Counter
Now, we have the same UI, but this time it’s built with vanilla HTML and JavaScript—no frameworks involved.
- Vanilla Demo .
"use client"; import { useState } from "react"; export default function Page() { const [counter, setCounter] = useState(0); const incrementClickHandler = (event: { preventDefault: () => void }) => { event.preventDefault(); setCounter((prevCounter) => prevCounter + 1); }; return ( <div style="{{" maxwidth: margin: auto> <a href="#" style="{{" display: padding: fontsize: border: solid black width: textalign: margintop: onclick="{incrementClickHandler}"> Increment {counter} </a> </div> ); }
One thing to mention is the necessity of the following element:
<script> let increment = 0; window.onload = function () { document.querySelector("#counter").innerText = increment; document.querySelector("a").addEventListener("click", function (event) { event.preventDefault(); increment++; document.querySelector("#counter").innerText = increment; }); }; </script> <a href="#" style=" display: inline-block; padding: 20px 40px; font-size: 28px; border: 1px solid black; width: 100%; text-align: center; text-decoration: none; color: black; margin-top: 40; box-sizing: border-box; ">Increment <span id="counter"></span> </a>
that is manipulated with JavaScript to update its content:
<span id="counter"></span>
Vanilla Counter UI
20 Seconds and Only One Click
Again, I’m going to click the record icon and let it run for 20 seconds, clicking the button only once.
Take a look at the results:
Vanilla | |
---|---|
JS Heap | 1.7MB |
Nodes | 16 |
Listeners | 20 |
20 Seconds with a Click per Second
Again, I’m going to click the record icon and let it run for another 20 seconds, but this time, I’ll click the button once per second. Check out the results:
Vanilla | |
---|---|
JS Heap | 2.3MB |
Nodes | 42 |
Listeners | 77 |
Just like in the React example, the blue line (JS Heap) and the yellow line (Listeners) increased over time. However, the green line (Nodes) is not constant; it increases as the button is clicked.
A Few Words on Garbage Collection
Garbage Collection: The main concept that garbage collection algorithms rely on is the concept of reference.
JavaScript automatically handles garbage collection for us; we don’t need to trigger it manually. In the previous examples, we saw how resources are consumed, but at some point, JavaScript takes care of releasing some of those resources through its garbage collector.
Conclusion
One click or twenty clicks isn’t that different in terms of resource consumption. As soon as a click happens, JavaScript allocates resources, and subsequent clicks continue to consume resources. However, the jump isn't as significant as the transition from zero to one click.
Let’s take a look at the end values for 20 clicks in both versions:
Vanilla | React | |
---|---|---|
JS Heap | 2.3MB | 4.0MB |
Nodes | 42 | 46 |
Listeners | 77 | 331 |
It makes sense that React consumes more resources; that’s the cost of using a framework.
One key difference is that React attaches all the nodes from the beginning, while the vanilla version adds nodes as the clicks happen. However, in the end, both versions ended up with pretty much the same number of nodes.
The demo is quite simple, and at this level, there’s no significant difference in terms of performance. As mentioned earlier, there’s a price to pay for using the framework, but it’s worth it considering all the features and conveniences it provides.
The above is the detailed content of How Many Resources Does a Click Consume? React vs. Vanilla. For more information, please follow other related articles on the PHP Chinese website!
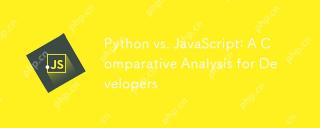
The main difference between Python and JavaScript is the type system and application scenarios. 1. Python uses dynamic types, suitable for scientific computing and data analysis. 2. JavaScript adopts weak types and is widely used in front-end and full-stack development. The two have their own advantages in asynchronous programming and performance optimization, and should be decided according to project requirements when choosing.
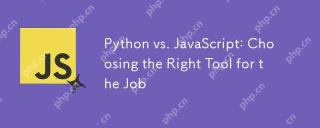
Whether to choose Python or JavaScript depends on the project type: 1) Choose Python for data science and automation tasks; 2) Choose JavaScript for front-end and full-stack development. Python is favored for its powerful library in data processing and automation, while JavaScript is indispensable for its advantages in web interaction and full-stack development.
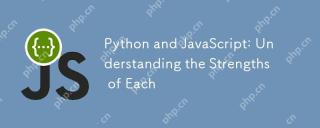
Python and JavaScript each have their own advantages, and the choice depends on project needs and personal preferences. 1. Python is easy to learn, with concise syntax, suitable for data science and back-end development, but has a slow execution speed. 2. JavaScript is everywhere in front-end development and has strong asynchronous programming capabilities. Node.js makes it suitable for full-stack development, but the syntax may be complex and error-prone.
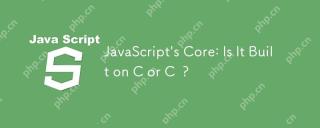
JavaScriptisnotbuiltonCorC ;it'saninterpretedlanguagethatrunsonenginesoftenwritteninC .1)JavaScriptwasdesignedasalightweight,interpretedlanguageforwebbrowsers.2)EnginesevolvedfromsimpleinterpreterstoJITcompilers,typicallyinC ,improvingperformance.
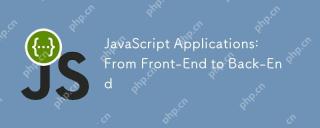
JavaScript can be used for front-end and back-end development. The front-end enhances the user experience through DOM operations, and the back-end handles server tasks through Node.js. 1. Front-end example: Change the content of the web page text. 2. Backend example: Create a Node.js server.
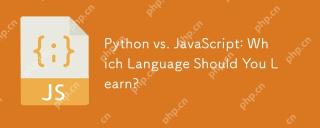
Choosing Python or JavaScript should be based on career development, learning curve and ecosystem: 1) Career development: Python is suitable for data science and back-end development, while JavaScript is suitable for front-end and full-stack development. 2) Learning curve: Python syntax is concise and suitable for beginners; JavaScript syntax is flexible. 3) Ecosystem: Python has rich scientific computing libraries, and JavaScript has a powerful front-end framework.
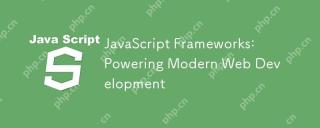
The power of the JavaScript framework lies in simplifying development, improving user experience and application performance. When choosing a framework, consider: 1. Project size and complexity, 2. Team experience, 3. Ecosystem and community support.
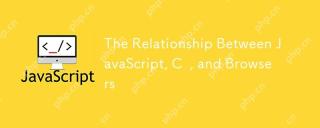
Introduction I know you may find it strange, what exactly does JavaScript, C and browser have to do? They seem to be unrelated, but in fact, they play a very important role in modern web development. Today we will discuss the close connection between these three. Through this article, you will learn how JavaScript runs in the browser, the role of C in the browser engine, and how they work together to drive rendering and interaction of web pages. We all know the relationship between JavaScript and browser. JavaScript is the core language of front-end development. It runs directly in the browser, making web pages vivid and interesting. Have you ever wondered why JavaScr


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
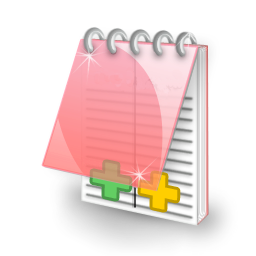
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
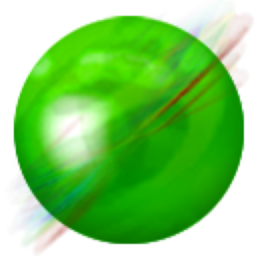
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
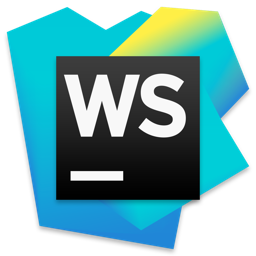
WebStorm Mac version
Useful JavaScript development tools
