If you’ve been developing with Laravel for a while, you’ve probably heard the phrase "clean code" tossed around. But what does it actually mean in the context of Laravel development? More importantly, why should you care?
"Clean Code" refers to code that is easy to understand, maintain, and extend. Clean code architecture takes this one step further by providing a structure that makes it easier to keep your codebase clean as your application grows. In this blog, we’ll explore how you can implement clean code architecture in Laravel to make your projects more scalable, maintainable, and enjoyable to work on.
Table of Contents
- What is Clean Code Architecture?
- Why You Should Care About Clean Code
- Key Principles of Clean Code Architecture
-
Implementing Clean Code Architecture in Laravel
- Entities and Use Cases
- Repositories and Interfaces
- Controllers and Dependency Injection
- Services and Business Logic
- Real-World Example: Building a Blog Platform
- Best Practices for Clean Code in Laravel
- Final Thoughts
What is Clean Code Architecture?
Clean code architecture is a way of organizing your application to make it easier to maintain and scale. It’s not tied to any particular framework or language, and it separates your application into layers. Each layer has a specific responsibility and is loosely coupled to the others.
This separation helps you avoid the infamous "spaghetti code" where everything is tangled together. With clean code architecture, your business logic is kept separate from your user interface and data access, allowing you to make changes to one part of the application without breaking the entire system.
Why You Should Care About Clean Code
Writing clean code isn’t just about making your code look nice; it’s about making your life easier in the long run. When your code is clean:
- It’s easier to debug: Clear, well-structured code helps you find and fix bugs faster.
- It’s easier to scale: When your app grows, clean code makes it easier to add new features without breaking existing ones.
- It’s easier to work with others: Whether you’re working with a team or sharing your project with the open-source community, clean code is easier for others to understand.
In a framework like Laravel, which encourages rapid development, it can be tempting to focus on building quickly rather than cleanly. But by following clean code principles, you’ll end up saving time and effort in the long run.
Key Principles of Clean Code Architecture
Before we dive into how to implement clean code architecture in Laravel, let’s go over a few key principles:
- Separation of Concerns: Each layer in your architecture should have a specific responsibility, and you should avoid mixing concerns (e.g., don’t put database logic in your controllers).
- Dependency Inversion: Higher-level modules should not depend on lower-level modules. Instead, both should depend on abstractions (like interfaces).
- Single Responsibility Principle: Every class or function should do one thing and do it well. This makes it easier to test and maintain your code.
Implementing Clean Code Architecture in Laravel
Now, let’s see how you can implement clean code architecture in a Laravel application.
Entities and Use Cases
At the core of clean code architecture are entities and use cases. Entities are the core objects in your system (like a Post or User), and use cases define what you can do with those entities (like creating a post or deleting a user).
In Laravel, entities can be represented as Eloquent models, while use cases are typically services that perform specific actions on these models.
For example, let’s create a simple use case for creating a blog post:
// app/Domain/Post/Post.php class Post { private $title; private $content; public function __construct($title, $content) { $this->title = $title; $this->content = $content; } // Getter methods and other domain logic }
And here’s a use case for creating a new post:
// app/Services/Post/CreatePostService.php class CreatePostService { private $postRepository; public function __construct(PostRepositoryInterface $postRepository) { $this->postRepository = $postRepository; } public function execute($data) { $post = new Post($data['title'], $data['content']); $this->postRepository->save($post); } }
Repositories and Interfaces
In clean code architecture, repositories handle data access, and interfaces define the methods that repositories should implement. This way, your business logic doesn’t depend on the database or ORM you’re using—it depends on the abstraction.
Let’s create an interface for the PostRepository:
// app/Repositories/PostRepositoryInterface.php interface PostRepositoryInterface { public function save(Post $post): void; public function findById($id): ?Post; }
And an Eloquent implementation of this repository:
// app/Repositories/EloquentPostRepository.php class EloquentPostRepository implements PostRepositoryInterface { public function save(Post $post): void { // Save post using Eloquent } public function findById($id): ?Post { // Fetch post using Eloquent } }
Controllers and Dependency Injection
Your controllers should be thin, meaning they should only handle HTTP requests and delegate the heavy lifting to services. By using dependency injection, you can inject the necessary services into your controllers.
// app/Http/Controllers/PostController.php class PostController { private $createPostService; public function __construct(CreatePostService $createPostService) { $this->createPostService = $createPostService; } public function store(Request $request) { $this->createPostService->execute($request->all()); return response()->json(['message' => 'Post created!']); } }
Services and Business Logic
All your business logic should live in services. This keeps your controllers clean and ensures that your logic can be reused across different parts of your application.
// app/Services/Post/CreatePostService.php class CreatePostService { private $postRepository; public function __construct(PostRepositoryInterface $postRepository) { $this->postRepository = $postRepository; } public function execute($data) { $post = new Post($data['title'], $data['content']); $this->postRepository->save($post); } }
Real-World Example: Building a Blog Platform
Let’s walk through a real-world example of building a simple blog platform. Here’s how you would structure it using clean code architecture:
- Entities: Your Post and User models.
- Repositories: Interfaces like PostRepositoryInterface and UserRepositoryInterface, and implementations like EloquentPostRepository.
- Services: CreatePostService, DeletePostService, etc.
- Controllers: Thin controllers that delegate work to services.
This separation ensures that your code remains modular and easy to test. For example, if you decide to switch from Eloquent to another ORM, you’d only need to update the repository implementation, not your entire application.
Best Practices for Clean Code in Laravel
- Keep Controllers Thin: Let your services handle the business logic.
- Use Dependency Injection: This makes your code easier to test and maintain.
- Write Unit Tests: Clean code architecture makes it easier to write tests, so make sure you take advantage of that.
- Separate Concerns: Keep business logic, data access, and presentation code separate.
Final Thoughts
Clean code architecture isn’t just for large enterprise applications—it’s a mindset that helps you keep your codebase clean and organized, even for small to medium-sized projects. By separating concerns, using dependency injection, and following the single responsibility principle, you’ll find your Laravel applications easier to maintain, test, and scale.
Start small. Try refactoring one part of your Laravel app to follow clean code architecture, and you’ll quickly see the benefits.
Happy coding!
The above is the detailed content of Clean Code Architecture in Laravel: A Practical Guide. For more information, please follow other related articles on the PHP Chinese website!
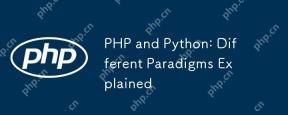
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
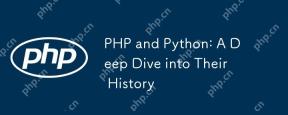
PHP originated in 1994 and was developed by RasmusLerdorf. It was originally used to track website visitors and gradually evolved into a server-side scripting language and was widely used in web development. Python was developed by Guidovan Rossum in the late 1980s and was first released in 1991. It emphasizes code readability and simplicity, and is suitable for scientific computing, data analysis and other fields.
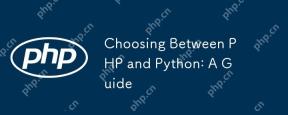
PHP is suitable for web development and rapid prototyping, and Python is suitable for data science and machine learning. 1.PHP is used for dynamic web development, with simple syntax and suitable for rapid development. 2. Python has concise syntax, is suitable for multiple fields, and has a strong library ecosystem.
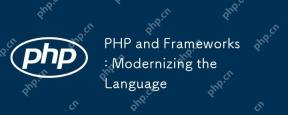
PHP remains important in the modernization process because it supports a large number of websites and applications and adapts to development needs through frameworks. 1.PHP7 improves performance and introduces new features. 2. Modern frameworks such as Laravel, Symfony and CodeIgniter simplify development and improve code quality. 3. Performance optimization and best practices further improve application efficiency.
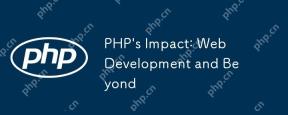
PHPhassignificantlyimpactedwebdevelopmentandextendsbeyondit.1)ItpowersmajorplatformslikeWordPressandexcelsindatabaseinteractions.2)PHP'sadaptabilityallowsittoscaleforlargeapplicationsusingframeworkslikeLaravel.3)Beyondweb,PHPisusedincommand-linescrip
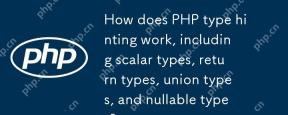
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.
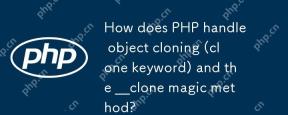
In PHP, use the clone keyword to create a copy of the object and customize the cloning behavior through the \_\_clone magic method. 1. Use the clone keyword to make a shallow copy, cloning the object's properties but not the object's properties. 2. The \_\_clone method can deeply copy nested objects to avoid shallow copying problems. 3. Pay attention to avoid circular references and performance problems in cloning, and optimize cloning operations to improve efficiency.
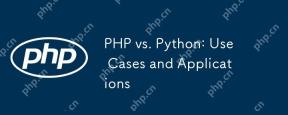
PHP is suitable for web development and content management systems, and Python is suitable for data science, machine learning and automation scripts. 1.PHP performs well in building fast and scalable websites and applications and is commonly used in CMS such as WordPress. 2. Python has performed outstandingly in the fields of data science and machine learning, with rich libraries such as NumPy and TensorFlow.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
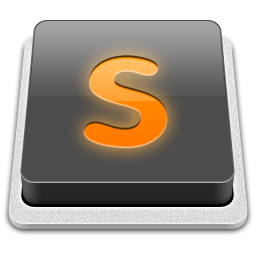
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use