


Upload Video Files with PHP and Save Them in Appropriate Folders and Create a Database Entry
This PHP script allows users to upload video files to a specified folder and create corresponding database entries:
<code class="php"><?php // Upload settings define('DESTINATION_FOLDER', '/uploads/'); // Folder to save uploaded videos define('MAX_FILE_SIZE', 10485760); // Maximum file size allowed in bytes (10MB) define('ALLOWED_EXTENSIONS', ['avi', 'mov', 'mp4']); // Allowed file extensions // Database settings define('DB_HOST', 'localhost'); define('DB_USER', 'root'); define('DB_PASSWORD', ''); define('DB_NAME', 'my_database'); // Initialize variables $errors = []; $message = ''; $uploadSuccess = false; // Process form submission if (isset($_POST['submit'])) { // Fetch form data $filename = $_FILES['filename']['name']; $tmp_filename = $_FILES['filename']['tmp_name']; $file_ext = strtolower(pathinfo($filename, PATHINFO_EXTENSION)); $filesize = $_FILES['filename']['size']; // Validate file if (!$tmp_filename) { $errors[] = 'Error: No file selected.'; } elseif (!in_array($file_ext, ALLOWED_EXTENSIONS)) { $errors[] = 'Error: File type not allowed.'; } elseif ($filesize > MAX_FILE_SIZE) { $errors[] = 'Error: File size exceeds limit.'; } // Upload file if no errors if (empty($errors)) { $newFilename = uniqid() . '.' . $file_ext; $uploadPath = DESTINATION_FOLDER . $newFilename; // Move uploaded file to the specified folder if (move_uploaded_file($tmp_filename, $uploadPath)) { $uploadSuccess = true; $message = 'File uploaded successfully.'; } else { $errors[] = 'Error: Unable to move uploaded file.'; } } // Create database entry if upload was successful if ($uploadSuccess) { try { // Establish database connection $conn = new PDO('mysql:host=' . DB_HOST . ';dbname=' . DB_NAME, DB_USER, DB_PASSWORD); $conn->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION); // Prepare SQL query $stmt = $conn->prepare("INSERT INTO uploads (`name`, `path`) VALUES (?, ?)"); $stmt->execute([$filename, $uploadPath]); } catch (PDOException $e) { $errors[] = 'Error: Database connection failed: ' . $e->getMessage(); } } } ?> <title>Upload Video Files</title> <h1 id="Upload-Video-Files">Upload Video Files</h1> <form method="post" enctype="multipart/form-data"> <label for="filename">Filename:</label> <input type="file" name="filename"> <input type="submit" name="submit" value="Upload"> </form> <?php if (!empty($message)): ?> <div><?php echo $message; ?></div> <?php endif; ?> <?php if (!empty($errors)): ?> <ul> <?php foreach ($errors as $error): ?> <li><?php echo $error; ?></li> <?php endforeach; ?> </ul> <?php endif; ?> </code>
Upon submission, this script performs the following tasks:
- Validates the uploaded file type, size, and extension.
- Moves the uploaded file to the specified folder.
- Creates a database connection and inserts an entry with the video's filename and path in the uploads table.
The above is the detailed content of How to Upload Video Files to a Folder, Create a Database Entry, and Perform Validation with PHP?. For more information, please follow other related articles on the PHP Chinese website!
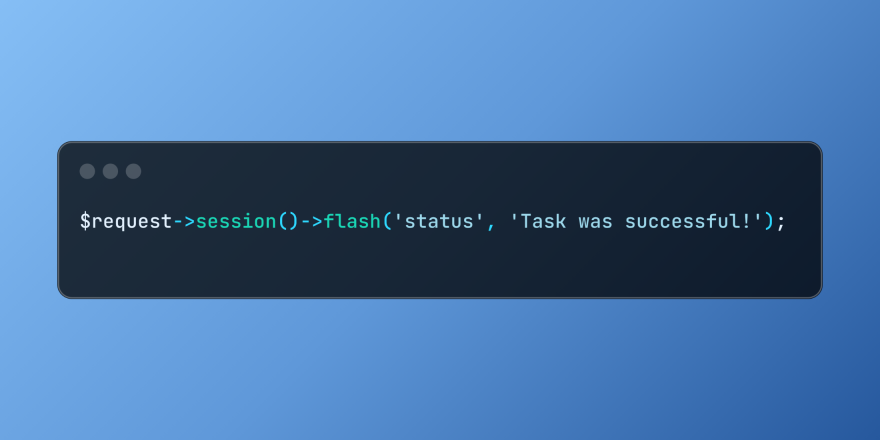
Laravel simplifies handling temporary session data using its intuitive flash methods. This is perfect for displaying brief messages, alerts, or notifications within your application. Data persists only for the subsequent request by default: $request-
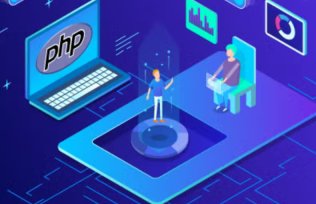
The PHP Client URL (cURL) extension is a powerful tool for developers, enabling seamless interaction with remote servers and REST APIs. By leveraging libcurl, a well-respected multi-protocol file transfer library, PHP cURL facilitates efficient execution of various network protocols, including HTTP, HTTPS, and FTP. This extension offers granular control over HTTP requests, supports multiple concurrent operations, and provides built-in security features.
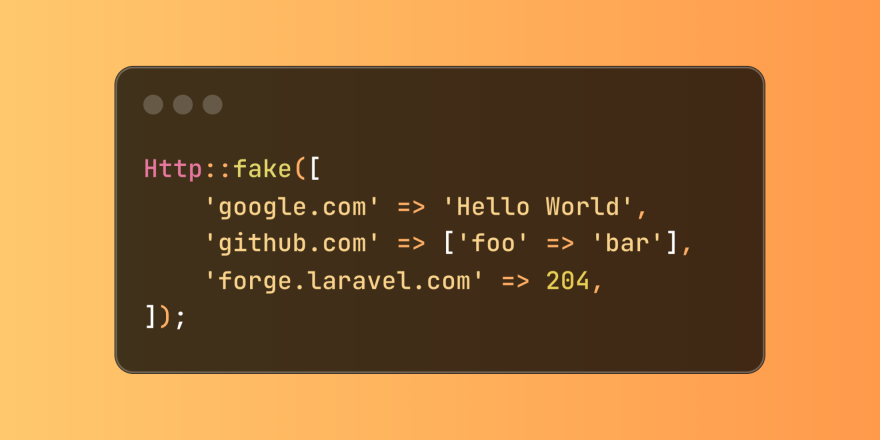
Laravel provides concise HTTP response simulation syntax, simplifying HTTP interaction testing. This approach significantly reduces code redundancy while making your test simulation more intuitive. The basic implementation provides a variety of response type shortcuts: use Illuminate\Support\Facades\Http; Http::fake([ 'google.com' => 'Hello World', 'github.com' => ['foo' => 'bar'], 'forge.laravel.com' =>
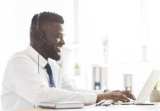
Do you want to provide real-time, instant solutions to your customers' most pressing problems? Live chat lets you have real-time conversations with customers and resolve their problems instantly. It allows you to provide faster service to your custom
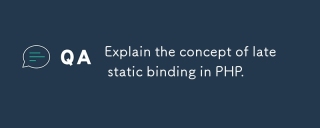
Article discusses late static binding (LSB) in PHP, introduced in PHP 5.3, allowing runtime resolution of static method calls for more flexible inheritance.Main issue: LSB vs. traditional polymorphism; LSB's practical applications and potential perfo
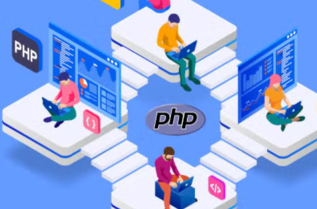
PHP logging is essential for monitoring and debugging web applications, as well as capturing critical events, errors, and runtime behavior. It provides valuable insights into system performance, helps identify issues, and supports faster troubleshoot
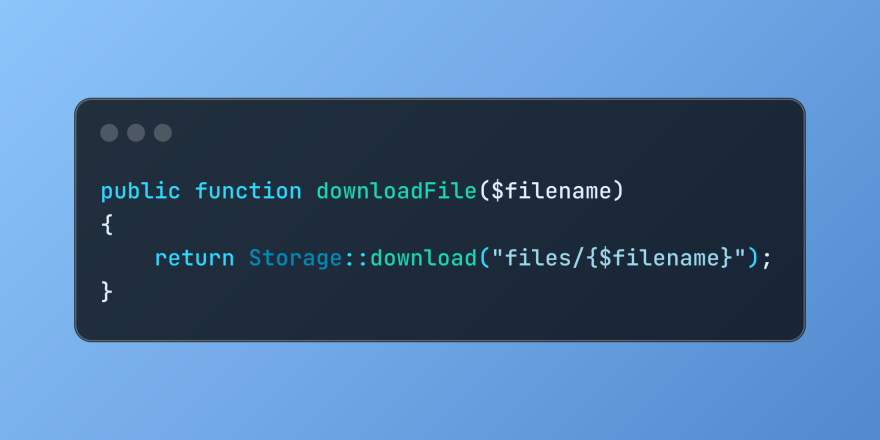
The Storage::download method of the Laravel framework provides a concise API for safely handling file downloads while managing abstractions of file storage. Here is an example of using Storage::download() in the example controller:
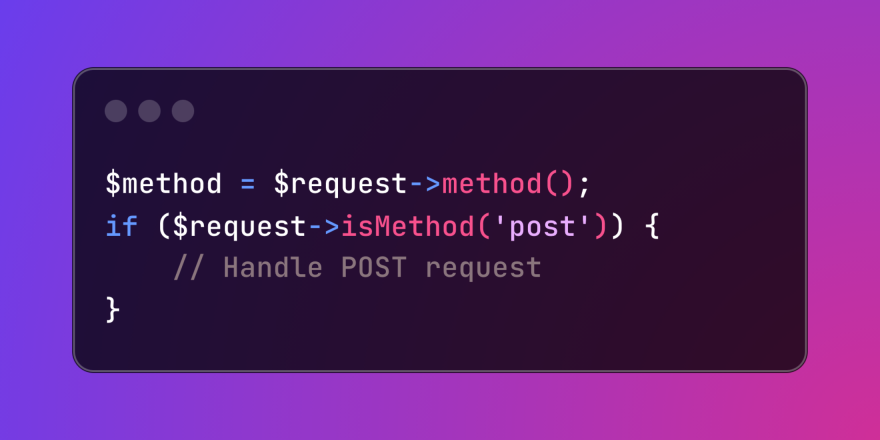
Laravel simplifies HTTP verb handling in incoming requests, streamlining diverse operation management within your applications. The method() and isMethod() methods efficiently identify and validate request types. This feature is crucial for building


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
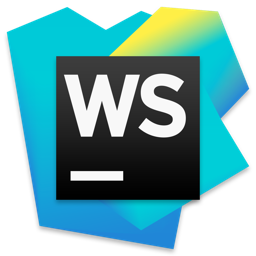
WebStorm Mac version
Useful JavaScript development tools

SublimeText3 Linux new version
SublimeText3 Linux latest version
