Sequential Execution of Promises with Parameter Passing from an Array
Consider the scenario where you have an array of values (e.g., myArray) and need to execute a promise-based function (e.g., myPromise) sequentially, passing each array element as a parameter. How can you implement a "pauseable loop" that ensures the promises are resolved in the correct order?
Solution: Iterative Promise Execution
To achieve sequential execution, you can use a combination of promise chaining and iterative processing. Here's a code snippet demonstrating how this can be done:
myArray.reduce( (p, x) => p.then(() => myPromise(x)), Promise.resolve() )
This approach leverages the reduce method to iterate over the array and create a series of chained promises. Each promise is resolved after the previous promise is completed, effectively enforcing the desired sequence of execution.
Async Function Alternative
If you have support for asynchronous functions, a cleaner solution is available using the forEachSeries function:
const forEachSeries = async (iterable, action) => { for (const x of iterable) { await action(x) } } forEachSeries(myArray, myPromise)
This function iterates over the array and pauses at each element, waiting for the promise to be resolved before proceeding to the next iteration.
Collecting Results
If you need to collect the return values of the promises into an array, you can modify the forEachSeries function as follows:
const mapSeries = async (iterable, fn) => { const results = [] for (const x of iterable) { results.push(await fn(x)) } return results }
This function iterates over the array, accumulates the results of the promises in the results array, and finally returns the collected results.
The above is the detailed content of How to Sequence Promise Execution with Parameter Passing from an Array?. For more information, please follow other related articles on the PHP Chinese website!
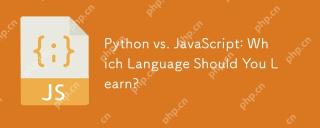
Choosing Python or JavaScript should be based on career development, learning curve and ecosystem: 1) Career development: Python is suitable for data science and back-end development, while JavaScript is suitable for front-end and full-stack development. 2) Learning curve: Python syntax is concise and suitable for beginners; JavaScript syntax is flexible. 3) Ecosystem: Python has rich scientific computing libraries, and JavaScript has a powerful front-end framework.
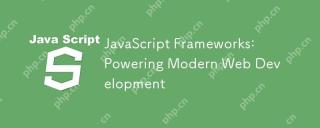
The power of the JavaScript framework lies in simplifying development, improving user experience and application performance. When choosing a framework, consider: 1. Project size and complexity, 2. Team experience, 3. Ecosystem and community support.
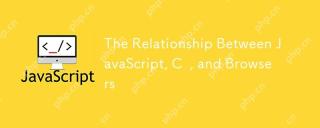
Introduction I know you may find it strange, what exactly does JavaScript, C and browser have to do? They seem to be unrelated, but in fact, they play a very important role in modern web development. Today we will discuss the close connection between these three. Through this article, you will learn how JavaScript runs in the browser, the role of C in the browser engine, and how they work together to drive rendering and interaction of web pages. We all know the relationship between JavaScript and browser. JavaScript is the core language of front-end development. It runs directly in the browser, making web pages vivid and interesting. Have you ever wondered why JavaScr
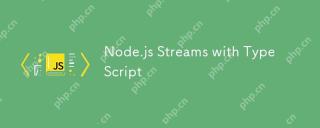
Node.js excels at efficient I/O, largely thanks to streams. Streams process data incrementally, avoiding memory overload—ideal for large files, network tasks, and real-time applications. Combining streams with TypeScript's type safety creates a powe
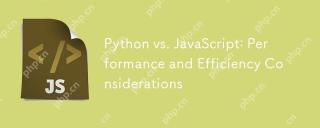
The differences in performance and efficiency between Python and JavaScript are mainly reflected in: 1) As an interpreted language, Python runs slowly but has high development efficiency and is suitable for rapid prototype development; 2) JavaScript is limited to single thread in the browser, but multi-threading and asynchronous I/O can be used to improve performance in Node.js, and both have advantages in actual projects.
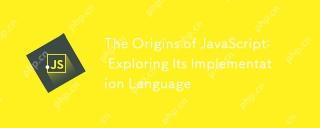
JavaScript originated in 1995 and was created by Brandon Ike, and realized the language into C. 1.C language provides high performance and system-level programming capabilities for JavaScript. 2. JavaScript's memory management and performance optimization rely on C language. 3. The cross-platform feature of C language helps JavaScript run efficiently on different operating systems.
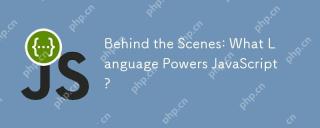
JavaScript runs in browsers and Node.js environments and relies on the JavaScript engine to parse and execute code. 1) Generate abstract syntax tree (AST) in the parsing stage; 2) convert AST into bytecode or machine code in the compilation stage; 3) execute the compiled code in the execution stage.
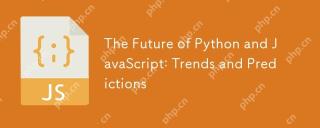
The future trends of Python and JavaScript include: 1. Python will consolidate its position in the fields of scientific computing and AI, 2. JavaScript will promote the development of web technology, 3. Cross-platform development will become a hot topic, and 4. Performance optimization will be the focus. Both will continue to expand application scenarios in their respective fields and make more breakthroughs in performance.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
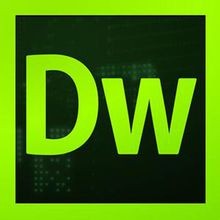
Dreamweaver CS6
Visual web development tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Linux new version
SublimeText3 Linux latest version

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SublimeText3 Chinese version
Chinese version, very easy to use
