This article will explore how React outlets function and the routing fundamentals in React applications. First and foremost we need to understand the basics of routing
Understanding the Basics
Before diving into , let's ensure we have a solid understanding of React Router.
What is a React Router? React Router is a popular JavaScript library that enables navigation between different views in a React application. It allows you to define routes, which map URLs to specific components. More..
Why is routing important? Routing is essential for building single-page applications (SPAs) where the user can navigate without full page reloads, creating a smoother and more dynamic experience.
Now let's dive into the React Outlet
What is React Outlet?
The
This feature helps in managing complex layouts where a portion of the page remains consistent, such as headers or sidebars, while other parts change dynamically based on the route.
How does it work: When the URL matches a parent route, the React Router looks for an
Let's Implement the Outlet with proper react routing for the React Application
import React from "react"; // import components import AllRoutes from "./routes"; // component const App = () => { return ( <allroutes></allroutes> > ); }; export default App;
This AllRoutes Component defines a routing system for a React application using react-router-dom, which handles navigation between different pages in a React web app. Here’s a detailed breakdown of the code
import React from "react"; // import components import AllRoutes from "./routes"; // component const App = () => { return ( <allroutes></allroutes> > ); }; export default App;
Importing Packages
BrowserRouter: This component enables routing in the browser. It uses the HTML5 history API to keep the UI in sync with the URL.
Outlet, Route, Routes: These components define and handle the various routes (or pages) in the application.
Suspense: Suspense is used to handle lazy-loaded components. It displays a fallback component (in this case, a custom loader) while the component is being loaded.
lazy: This function enables code-splitting by lazily loading components only when they are needed, improving the app's performance by reducing the initial bundle size.
Loader: This is a custom component that shows a loading indicator while the components are being lazily loaded.
ProtectedRoute: This custom component ensures that certain routes are only accessible to authorized users, enforcing security.
Root Route (/): This route is for the root path of our application. It renders the Login component.
Importing Route Paths
The application imports predefined paths (e.g., dashboard, homePath, loginPath, rootPath) from a separate file (routePaths). This makes the code easier to maintain and avoids hardcoding route paths within the components.
Defining Routes in the AllRoutesComponent
The main function, AllRoutesComponent, defines how the different routes in the app are handled:
The root (rootPath) route serves as a container for child routes.
The index route renders the LoginComponent by default when the user visits the / path.
The /login path also renders the LoginComponent.
Routes like /home and /dashboard are wrapped inside a ProtectedRoute component, ensuring that only authorized users can access these pages.
What are the Protected Routes?
Some routes (like homePath and dashboard) are nested inside a ProtectedRoute element. This means users must meet certain conditions (like being logged in) to access those pages. If a user is unauthorized, the ProtectedRoute component typically redirects them to the login page or an error page.
Here is the code of the Protected route
// import packages import { BrowserRouter, Outlet, Route, Routes } from "react-router-dom"; import React, { Suspense, lazy } from "react"; import { dashboard, homePath, loginPath, rootPath } from "./routePaths"; import Loader from "../components/loader"; import ProtectedRoute from "./protectedRoute"; // import route paths const LoginComponent = lazy(() => import("../pages/login")); const HomeComponent = lazy(() => import("../pages/home")); const RouteNotFoundComponent = lazy(() => import("../pages/pageNotFound")); const DashboardComponent = lazy(() => import("../pages/dashboard/")); const AllRoutesComponent = () => { return ( <suspense fallback="{<Loader"></suspense>}> <browserrouter> <routes> <route path="{rootPath}" element="{<Outlet"></route>}> <route index element="{<LoginComponent"></route>} /> <route path="{loginPath}" element="{<LoginComponent"></route>} /> <route element="{<ProtectedRoute"></route>}> <route path="{homePath}" element="{<HomeComponent"></route>} /> <route path="{dashboard}" element="{<DashboardComponent"></route>} /> <route path="*" element="{<RouteNotFoundComponent"></route>} /> </routes> </browserrouter> ); }; export default AllRoutesComponent;
Explanation about the code
- Importing Packages
React, { useEffect }: React is the core package for building the UI, and useEffect is a hook that runs side effects in functional components. Here, useEffect is used to handle the redirection if the user is not authenticated.
useNavigate: This hook from react-router-dom enables programmatic navigation. It's used to redirect users when they are not authenticated.
Outlet: This is a placeholder for nested routes inside the protected route. It allows the rendering child routes dynamically.
BaseLayout: This component wraps the protected route with a layout, providing a consistent look and structure for protected pages.
- Component Definition
useNavigate(): This hook allows navigating to different routes. In this case, it is used to navigate to rootPath if the user is not authenticated.
isAuthenticated and user: These values are hardcoded for now but can be dynamically set, typically by checking some authentication state (like a JWT token, session data, etc.) from a global state or a context.
isAuthenticated: A flag that determines whether the user is authenticated. If false, the user is redirected.
user: The current logged-in user. This value can be dynamically retrieved from a context or global state, but for now, it's a hardcoded string ('shruti').
useEffect:The useEffect hook runs whenever authenticated or navigates changes.
Inside useEffect, the code checks whether the user is authenticated. If not (! is authenticated), the user is redirected to the root path using the navigate function.
The { replace: true } option prevents adding the redirection to the browser's history, meaning the user won't be able to navigate back to the protected page.
Now Let's dive in to the BaseLayout Component Code
import React from "react"; // import components import AllRoutes from "./routes"; // component const App = () => { return ( <allroutes></allroutes> > ); }; export default App;
The BaseLayout component serves as a common layout structure for all pages in our application.
It contains common elements like a sidebar and main content area.
Child components from different routes are rendered using the Outlet component within the main content area.
Try this article to implement the lazy loading routing with router outlet
Conclusion
Lazy loading routing with Outlet in React is a powerful way to optimize performance by reducing the initial load time of your app. By using React.lazy() and Suspense, you ensure that components are loaded only when needed, while the Outlet component allows for efficient nested routing. Combined with the use of protected routes, this approach provides a modular, scalable, and performance-friendly structure for managing routes in your application.
That's It!
Give it a try, and let me know your thoughts in the comments!
The above is the detailed content of Understanding about React Outlet with proper routing. For more information, please follow other related articles on the PHP Chinese website!
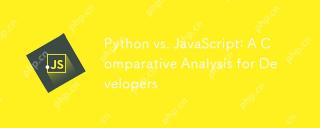
The main difference between Python and JavaScript is the type system and application scenarios. 1. Python uses dynamic types, suitable for scientific computing and data analysis. 2. JavaScript adopts weak types and is widely used in front-end and full-stack development. The two have their own advantages in asynchronous programming and performance optimization, and should be decided according to project requirements when choosing.
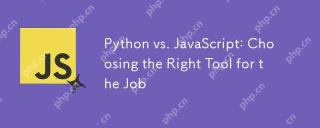
Whether to choose Python or JavaScript depends on the project type: 1) Choose Python for data science and automation tasks; 2) Choose JavaScript for front-end and full-stack development. Python is favored for its powerful library in data processing and automation, while JavaScript is indispensable for its advantages in web interaction and full-stack development.
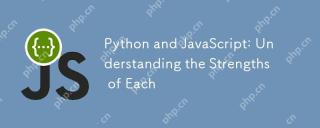
Python and JavaScript each have their own advantages, and the choice depends on project needs and personal preferences. 1. Python is easy to learn, with concise syntax, suitable for data science and back-end development, but has a slow execution speed. 2. JavaScript is everywhere in front-end development and has strong asynchronous programming capabilities. Node.js makes it suitable for full-stack development, but the syntax may be complex and error-prone.
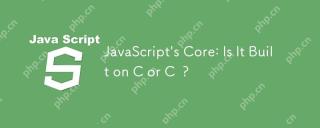
JavaScriptisnotbuiltonCorC ;it'saninterpretedlanguagethatrunsonenginesoftenwritteninC .1)JavaScriptwasdesignedasalightweight,interpretedlanguageforwebbrowsers.2)EnginesevolvedfromsimpleinterpreterstoJITcompilers,typicallyinC ,improvingperformance.
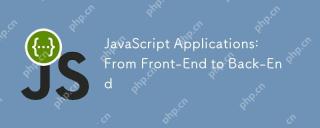
JavaScript can be used for front-end and back-end development. The front-end enhances the user experience through DOM operations, and the back-end handles server tasks through Node.js. 1. Front-end example: Change the content of the web page text. 2. Backend example: Create a Node.js server.
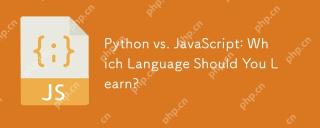
Choosing Python or JavaScript should be based on career development, learning curve and ecosystem: 1) Career development: Python is suitable for data science and back-end development, while JavaScript is suitable for front-end and full-stack development. 2) Learning curve: Python syntax is concise and suitable for beginners; JavaScript syntax is flexible. 3) Ecosystem: Python has rich scientific computing libraries, and JavaScript has a powerful front-end framework.
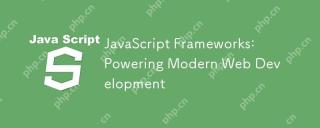
The power of the JavaScript framework lies in simplifying development, improving user experience and application performance. When choosing a framework, consider: 1. Project size and complexity, 2. Team experience, 3. Ecosystem and community support.
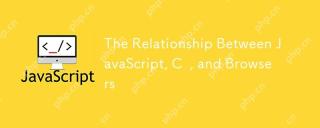
Introduction I know you may find it strange, what exactly does JavaScript, C and browser have to do? They seem to be unrelated, but in fact, they play a very important role in modern web development. Today we will discuss the close connection between these three. Through this article, you will learn how JavaScript runs in the browser, the role of C in the browser engine, and how they work together to drive rendering and interaction of web pages. We all know the relationship between JavaScript and browser. JavaScript is the core language of front-end development. It runs directly in the browser, making web pages vivid and interesting. Have you ever wondered why JavaScr


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
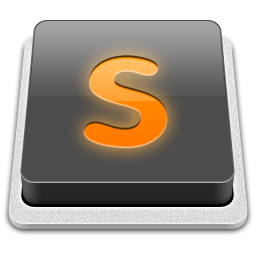
SublimeText3 Mac version
God-level code editing software (SublimeText3)
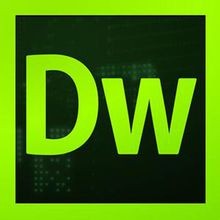
Dreamweaver CS6
Visual web development tools
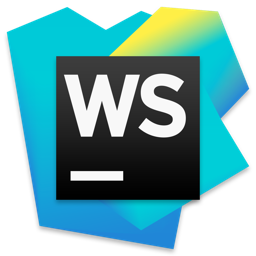
WebStorm Mac version
Useful JavaScript development tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
