In my previous blogs, I explored various creational design patterns that deal with object creation mechanisms. Now, it’s time to dive into structural design patterns, which focus on how objects and classes are composed to form larger structures while keeping them flexible and efficient. Let's start with proxy design pattern
Proxy Design Pattern in JavaScript
The Proxy design pattern is a structural design pattern that provides an object representing another object. It acts as an intermediary that controls access to the real object, adding additional behavior such as lazy initialization, logging, access control, or caching, without changing the original object’s code.
In JavaScript, proxies are built-in features provided by the Proxy object, allowing you to define custom behavior for fundamental operations such as property access, assignment, function invocation, etc.
When Do We Need the Proxy Pattern?
The Proxy pattern is particularly useful when:
- Lazy Initialization: You want to delay the creation of a resource-heavy object until it is needed.
- Access Control: You need to control access to an object, for example, to restrict unauthorized access or to limit operations based on conditions.
- Logging: You want to log actions on an object (e.g., property access or method calls).
- Caching: You want to cache the result of expensive operations to avoid redundant computations.
Components of Proxy Pattern
- Subject: The interface that defines the common operations for both the real object and the proxy.
- RealSubject: The actual object that performs the real work.
- Proxy: The intermediary that controls access to the RealSubject.
Analogy:
Imagine you have a large painting that you want to show your guests, but it takes a lot of time to pull it out from a storage room (because it's heavy and takes time to carry). Instead of waiting for that every time, you decide to use a small postcard image of the painting to show them quickly while they wait for the actual painting to be fetched.
In this analogy:
- The large painting is the real object (like an image that takes time to load).
- The postcard is the proxy (a lightweight substitute that stands in until the real object is ready).
- Once the real painting is ready, you show the actual one to your guests.
Real-World Analogy:
Think of a real estate agent as a proxy. When you want to buy a house, you don’t immediately visit every house (loading the real object). Instead, the real estate agent (proxy) first shows you photos and descriptions. Only when you’re ready to buy (i.e., when you call display()), the agent arranges a house visit (loads the real object).
Real-World Example: Image Loading (Virtual Proxy)
Let’s use the example of image loading in a web application where we want to delay the loading of the image until the user requests it (lazy loading). A proxy can act as a placeholder until the real image is loaded.
Here’s how you can implement the Proxy design pattern in JavaScript.
Example: Proxy for Image Loading
// Step 1: The real object class RealImage { constructor(filename) { this.filename = filename; this.loadImageFromDisk(); } loadImageFromDisk() { console.log(`Loading ${this.filename} from disk...`); } display() { console.log(`Displaying ${this.filename}`); } } // Step 2: The proxy object class ProxyImage { constructor(filename) { this.realImage = null; // no real image yet this.filename = filename; } display() { if (this.realImage === null) { // load the real image only when needed this.realImage = new RealImage(this.filename); } this.realImage.display(); // display the real image } } // Step 3: Using the proxy to display the image const image = new ProxyImage("photo.jpg"); image.display(); // Loads and displays the image image.display(); // Just displays the image (already loaded)
Explanation:
1). The Real Image:
- The RealImageclass represents the actual image.
- It takes a filename as input and simulates the time-consuming process of loading the image from disk (shown by the loadImageFromDiskmethod).
- Once loaded, the displaymethod is used to show the image.
2). The Proxy Image:
- The ProxyImageclass acts as a stand-in for the RealImage. It doesn’t load the real image immediately.
- It holds a reference to the real image (but initially it’s nullbecause the real image hasn’t been loaded yet).
- When you call the displaymethod on the proxy, it checks if the real image has been loaded. If not, it loads it first, and then displays it.
3). Usage:
- When we create an instance of ProxyImage, the actual image is not loaded yet (because it's resource-intensive).
- The first time displayis called, the proxy loads the image (using the RealImageclass) and then displays it.
- The second time displayis called, the real image has already been loaded, so it only displays the image without loading it again.
The built-in Proxy object
The ES6 proxy consists of a proxy constructor that accepts a target & handler as arguments
const proxy = new Proxy(target, handler)
Here, target represents the object on which proxy is applied, while handler is a special object that defines the behaviour of the proxy.
The handler object contains a series of optional methods with predefined names called trap methods ( forexample apply,get,set and has) that are automatically called when the corresponding operations are performed on the proxy instance.
Let's understand this by implementing calculator using built-in proxy
// Step 1: The real object class RealImage { constructor(filename) { this.filename = filename; this.loadImageFromDisk(); } loadImageFromDisk() { console.log(`Loading ${this.filename} from disk...`); } display() { console.log(`Displaying ${this.filename}`); } } // Step 2: The proxy object class ProxyImage { constructor(filename) { this.realImage = null; // no real image yet this.filename = filename; } display() { if (this.realImage === null) { // load the real image only when needed this.realImage = new RealImage(this.filename); } this.realImage.display(); // display the real image } } // Step 3: Using the proxy to display the image const image = new ProxyImage("photo.jpg"); image.display(); // Loads and displays the image image.display(); // Just displays the image (already loaded)
The best part using proxy this way as :
- The proxy object inherits the prototype of the original Calculator class.
- Mutations are avoided through the set trap of the Proxy.
Explanation of the Code
1). Prototype Inheritance:
- The proxy does not interfere with the original prototype of the **Calculator **class.
- this is confirmed by checking if proxiedCalculator.proto === Calculator.prototype. The result will be true.
2). Handling getOperations:
- The get trap intercepts property access on the proxy object.
- We use Reflect.get to safely access properties and methods from the original object.
3). Preventing Mutations:
The set trap throws an error whenever there is an attempt to modify any property on the target object. This ensures immutability.
4). Using Prototype Methods through the Proxy:
The proxy allows access to methods such as add, subtract, multiply, and divide, all of which are defined on the original object's prototype.
Key points to observe here is:
- Preserves Prototype Inheritance: The proxy retains access to all prototype methods, making it behave like the original Calculator.
- Prevents Mutation: The set trap ensures the internal state of the calculator object cannot be altered unexpectedly.
- Safe Access to Properties and Methods: The get trap ensures only valid properties are accessed, improving robustness.
If you've made it this far, don't forget to hit like ❤️ and drop a comment below with any questions or thoughts. Your feedback means the world to me, and I'd love to hear from you!
The above is the detailed content of Proxy Design Pattern. For more information, please follow other related articles on the PHP Chinese website!
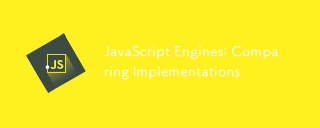
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
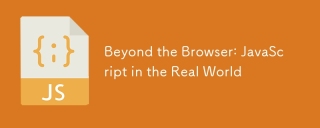
JavaScript's applications in the real world include server-side programming, mobile application development and Internet of Things control: 1. Server-side programming is realized through Node.js, suitable for high concurrent request processing. 2. Mobile application development is carried out through ReactNative and supports cross-platform deployment. 3. Used for IoT device control through Johnny-Five library, suitable for hardware interaction.
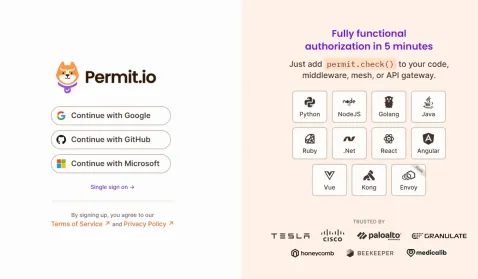
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
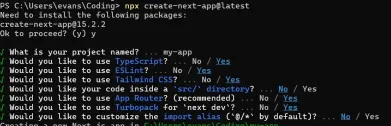
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
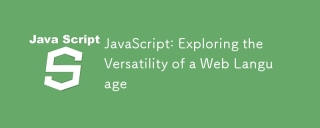
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
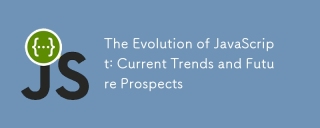
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
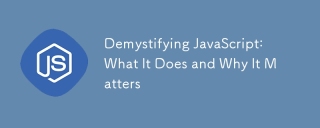
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
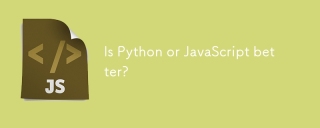
Python is more suitable for data science and machine learning, while JavaScript is more suitable for front-end and full-stack development. 1. Python is known for its concise syntax and rich library ecosystem, and is suitable for data analysis and web development. 2. JavaScript is the core of front-end development. Node.js supports server-side programming and is suitable for full-stack development.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
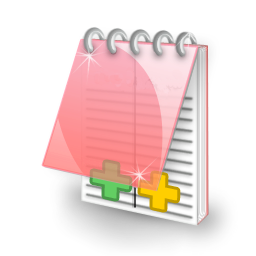
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SublimeText3 Linux new version
SublimeText3 Linux latest version

SublimeText3 Chinese version
Chinese version, very easy to use