Read full post at Getting Started with Maven
Overview
If you're a Java developer, you've likely used Maven to build and manage your projects.
In this post, you'll learn the basics of using Maven in your projects.
Understanding Maven's Project Structure
- my-maven-project/pom.xml
Defines the Maven project. It includes a unique identifier for the project, properties, dependencies, and necessary modules.
- my-maven-project/src/main/java
Contains the source code of the project.
- my-maven-project/src/resources
Contains resource files (like application.properties).
- my-maven-project/src/test
Contains the test code.
- my-maven-project/src/it
Contains integration tests.
- my-maven-project/target
Contains compiled classes, JAR/WAR files, and other artifacts.
POM File
Key Elements
The pom.xml file holds crucial information about the project. Each project must have a unique identifier, specified by:
groupId (usually your domain name)
artifactId (usually the project name)
version (project version)
Additionally, the pom.xml can include the project's name, description, and packaging type (e.g., JAR/WAR).
Example:
<groupid>com.kramti</groupid> <artifactid>example</artifactid> <version>1.0</version> <name>My Maven Example</name> <description>This is a Maven pom.xml example</description> <packaging>war</packaging> <properties> <java.version>21</java.version> </properties>
Dependencies
Dependencies are external libraries your project requires. Instead of manually downloading and importing JAR files, Maven simplifies this by managing dependencies within the pom.xml.
To add a dependency, you need its groupId, artifactId, version (optional), and scope (optional). You can find dependencies on the Maven Repository.
Example of a dependency:
<dependency> <groupid>org.springframework.boot</groupid> <artifactid>spring-boot-starter-data-jpa</artifactid> <version></version> </dependency>
For example, if you need a dependency only for testing purposes, use the scope tag:
<dependency> <groupid>org.springframework.boot</groupid> <artifactid>spring-boot-starter-test</artifactid> <scope>test</scope> </dependency>
Maven Build Lifecycle
- validate: Checks if the project is correct and all necessary information is available.
compile: Compiles the project's source code.
test: Runs unit tests using a suitable testing framework.
package: Packages the compiled code into a distributable format (e.g., JAR or WAR).
install: Installs the package into the local repository for use by other projects.
deploy: Deploys the built package to a remote repository for sharing with other developers.
Maven Plugins
Using Maven Plugins Maven plugins are essential for customizing the build process. You can use plugins for tasks such as compiling code, running tests, and generating reports.
Example of a compiler plugin:
<groupid>com.kramti</groupid> <artifactid>example</artifactid> <version>1.0</version> <name>My Maven Example</name> <description>This is a Maven pom.xml example</description> <packaging>war</packaging> <properties> <java.version>21</java.version> </properties>
Popular plugins include:
maven-compiler-plugin: Compiles Java source code.
maven-surefire-plugin: Runs unit tests.
maven-jar-plugin: Packages the project into a JAR file.
Best Practices for Maven Projects
Keep Dependencies Up-to-Date: Regularly update your dependencies to avoid vulnerabilities.
Avoid Version Conflicts: Use the section in the parent POM to manage dependency versions in multi-module projects.
Minimize Plugin Configuration: Keep plugin configurations minimal and only configure what’s necessary for your project.
Build performance: For large projects, Maven builds can be slow. Use the -T option to enable parallel builds
Conclusion
Maven is an essential tool for Java developers. It simplifies project management by automating builds, handling dependencies and plugins. Understanding its structure, POM file, and core features allows for more efficient and organized workflows.
You may be interested in
Java Performance Optimization Techniques
Docker in nutshell
Transactions in Spring boot
The above is the detailed content of Getting Started with Maven. For more information, please follow other related articles on the PHP Chinese website!

The article discusses using Maven and Gradle for Java project management, build automation, and dependency resolution, comparing their approaches and optimization strategies.
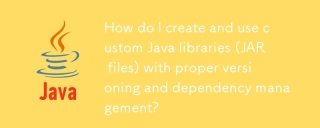
The article discusses creating and using custom Java libraries (JAR files) with proper versioning and dependency management, using tools like Maven and Gradle.
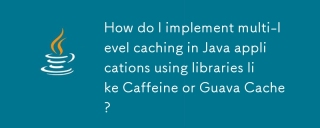
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra

The article discusses using JPA for object-relational mapping with advanced features like caching and lazy loading. It covers setup, entity mapping, and best practices for optimizing performance while highlighting potential pitfalls.[159 characters]
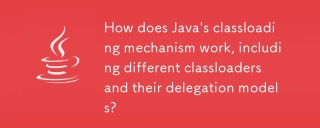
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
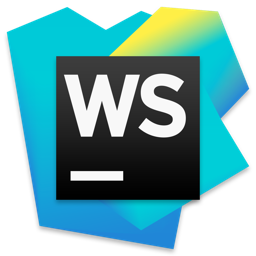
WebStorm Mac version
Useful JavaScript development tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
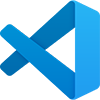
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft