Java またはオブジェクト指向プログラミング (OOP) 言語を学習する場合、カプセル化 と 抽象化 という 2 つの重要な概念が際立ちます。これらの概念は、コードの再利用性、セキュリティ、保守性を促進する OOP の重要な柱です。これらは一緒に使用されることが多いですが、異なる目的を果たします。
この投稿では、Java プログラミングにおけるカプセル化と抽象化の役割を理解するのに役立つ明確な定義、例、コード スニペットを使用して、カプセル化と抽象化の違いを詳しく説明します。分解してみましょう!
カプセル化とは何ですか?
カプセル化は、データ (変数) とそのデータを操作するメソッドを単一のユニット (通常はクラス) にバンドルするプロセスです。オブジェクトの内部状態を外部から隠し、パブリック メソッドを介した制御されたアクセスのみを許可します。
カプセル化の主な特徴:
- データ隠蔽: 内部オブジェクト データは他のクラスから隠蔽されます。
- アクセス制御: 許可された (パブリック) メソッドのみが非表示データを操作できます。
- セキュリティの向上: 外部コードが内部データを直接変更することを防ぎます。
- 簡単なメンテナンス: 内部実装が変更された場合、外部クラスではなくメソッドのみを更新する必要があります。
Java でのカプセル化の例:
// Encapsulation in action public class Employee { // Private variables (data hiding) private String name; private int age; // Getter and setter methods (controlled access) public String getName() { return name; } public void setName(String name) { this.name = name; } public int getAge() { return age; } public void setAge(int age) { this.age = age; } } // Using the encapsulated class public class Main { public static void main(String[] args) { Employee emp = new Employee(); emp.setName("John Doe"); emp.setAge(30); System.out.println("Employee Name: " + emp.getName()); System.out.println("Employee Age: " + emp.getAge()); } }
この例では、Employee クラスはそのフィールド (名前と年齢) をプライベートと宣言することで非表示にします。 Main などの外部クラスは、入出力を制御および検証するゲッター メソッドとセッター メソッドを介してのみこれらのフィールドにアクセスできます。
抽象化とは何ですか?
抽象化とは、オブジェクトの複雑な実装の詳細を隠し、重要な機能のみを公開するという概念を指します。これにより、オブジェクトとの対話が簡素化され、コードがよりユーザーフレンドリーになります。
Key Features of Abstraction:
- Hides complexity: Users only see what they need, and the underlying code is hidden.
- Focus on 'what' rather than 'how': Provides only the necessary details to the user while keeping implementation hidden.
- Helps in managing complexity: Useful for working with complex systems by providing simplified interfaces.
- Enforced via interfaces and abstract classes: These constructs provide a blueprint without exposing implementation.
Example of Abstraction in Java:
// Abstract class showcasing abstraction abstract class Animal { // Abstract method (no implementation) public abstract void sound(); // Concrete method public void sleep() { System.out.println("Sleeping..."); } } // Subclass providing implementation for abstract method class Dog extends Animal { public void sound() { System.out.println("Barks"); } } public class Main { public static void main(String[] args) { Animal dog = new Dog(); dog.sound(); // Calls the implementation of the Dog class dog.sleep(); // Calls the common method in the Animal class } }
Here, the abstract class Animal contains an abstract method sound() which must be implemented by its subclasses. The Dog class provides its own implementation for sound(). This way, the user doesn't need to worry about how the sound() method works internally—they just call it.
Encapsulation vs. Abstraction: Key Differences
Now that we’ve seen the definitions and examples, let’s highlight the key differences between encapsulation and abstraction in Java:
Feature | Encapsulation | Abstraction |
---|---|---|
Purpose | Data hiding and protecting internal state | Simplifying code by hiding complex details |
Focus | Controls access to data using getters/setters | Provides essential features and hides implementation |
Implementation | Achieved using classes with private fields | Achieved using abstract classes and interfaces |
Role in OOP | Increases security and maintains control over data | Simplifies interaction with complex systems |
Example | Private variables and public methods | Abstract methods and interfaces |
Practical Use Cases in Java
When to Use Encapsulation:
- When you need to protect data: For example, in a banking system where account balances should not be modified directly.
- When you want control over how data is accessed: Ensures that only allowed methods modify or retrieve the data, adding a layer of security.
When to Use Abstraction:
- When working on large-scale systems: In large projects where various classes and modules interact, abstraction can help manage complexity by providing simplified interfaces.
- When developing APIs: Expose only necessary details to the user while keeping the actual implementation hidden.
Benefits of Combining Encapsulation and Abstraction
Though encapsulation and abstraction serve different purposes, they work together to build robust, secure, and maintainable code in Java.
- Security and Flexibility: By combining both, you ensure that data is protected (encapsulation) while still allowing users to interact with it in a simple manner (abstraction).
- Code Maintainability: Abstraction hides complexity, making the system easier to manage, while encapsulation provides controlled access to the data.
- Reusability: Both concepts promote code reuse—encapsulation by isolating data, and abstraction by allowing different implementations of abstract methods.
Conclusion: Mastering Encapsulation and Abstraction in Java
Encapsulation and abstraction are two powerful concepts in object-oriented programming that every Java developer should master. While encapsulation helps protect an object's internal state by controlling data access, abstraction hides the complexities of a system and provides only the necessary details.
By understanding and applying both, you can build secure, maintainable, and scalable applications that stand the test of time.
Did this guide help you clarify encapsulation and abstraction in Java? Share your thoughts or questions in the comments below!
Tags:
- #Java
- #OOP
- #Encapsulation
- #Abstraction
- #JavaProgramming
The above is the detailed content of Encapsulation vs. Abstraction in Java: The Ultimate Guide. For more information, please follow other related articles on the PHP Chinese website!
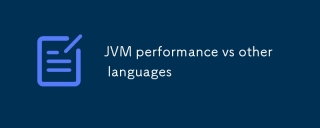
JVM'sperformanceiscompetitivewithotherruntimes,offeringabalanceofspeed,safety,andproductivity.1)JVMusesJITcompilationfordynamicoptimizations.2)C offersnativeperformancebutlacksJVM'ssafetyfeatures.3)Pythonisslowerbuteasiertouse.4)JavaScript'sJITisles

JavaachievesplatformindependencethroughtheJavaVirtualMachine(JVM),allowingcodetorunonanyplatformwithaJVM.1)Codeiscompiledintobytecode,notmachine-specificcode.2)BytecodeisinterpretedbytheJVM,enablingcross-platformexecution.3)Developersshouldtestacross
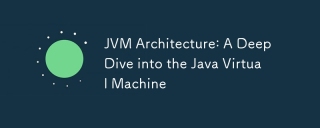
TheJVMisanabstractcomputingmachinecrucialforrunningJavaprogramsduetoitsplatform-independentarchitecture.Itincludes:1)ClassLoaderforloadingclasses,2)RuntimeDataAreafordatastorage,3)ExecutionEnginewithInterpreter,JITCompiler,andGarbageCollectorforbytec
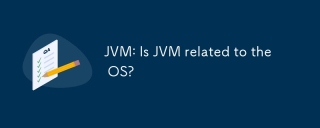
JVMhasacloserelationshipwiththeOSasittranslatesJavabytecodeintomachine-specificinstructions,managesmemory,andhandlesgarbagecollection.ThisrelationshipallowsJavatorunonvariousOSenvironments,butitalsopresentschallengeslikedifferentJVMbehaviorsandOS-spe
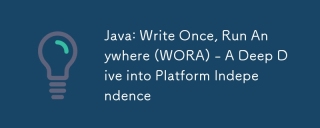
Java implementation "write once, run everywhere" is compiled into bytecode and run on a Java virtual machine (JVM). 1) Write Java code and compile it into bytecode. 2) Bytecode runs on any platform with JVM installed. 3) Use Java native interface (JNI) to handle platform-specific functions. Despite challenges such as JVM consistency and the use of platform-specific libraries, WORA greatly improves development efficiency and deployment flexibility.
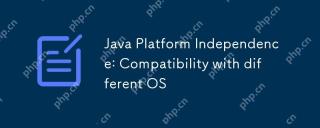
JavaachievesplatformindependencethroughtheJavaVirtualMachine(JVM),allowingcodetorunondifferentoperatingsystemswithoutmodification.TheJVMcompilesJavacodeintoplatform-independentbytecode,whichittheninterpretsandexecutesonthespecificOS,abstractingawayOS
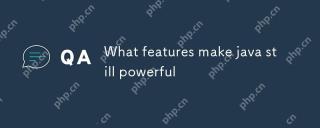
Javaispowerfulduetoitsplatformindependence,object-orientednature,richstandardlibrary,performancecapabilities,andstrongsecurityfeatures.1)PlatformindependenceallowsapplicationstorunonanydevicesupportingJava.2)Object-orientedprogrammingpromotesmodulara
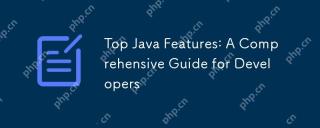
The top Java functions include: 1) object-oriented programming, supporting polymorphism, improving code flexibility and maintainability; 2) exception handling mechanism, improving code robustness through try-catch-finally blocks; 3) garbage collection, simplifying memory management; 4) generics, enhancing type safety; 5) ambda expressions and functional programming to make the code more concise and expressive; 6) rich standard libraries, providing optimized data structures and algorithms.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
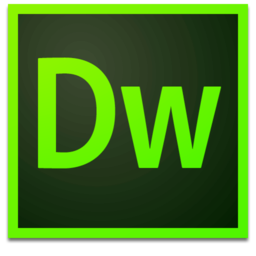
Dreamweaver Mac version
Visual web development tools
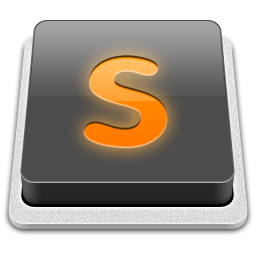
SublimeText3 Mac version
God-level code editing software (SublimeText3)
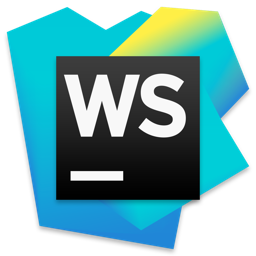
WebStorm Mac version
Useful JavaScript development tools

Atom editor mac version download
The most popular open source editor

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
