In C and C , char** is a pointer to a pointer of type char. It is commonly used to represent arrays of strings, such as command-line arguments (argv), dynamic arrays of strings, or 2D arrays where each row is a string. Though initially confusing, with some examples, you’ll see how it operates similarly to handling a "table of strings."
What is char* *?
A char* is a pointer to a char, representing a single string.
A char** is a pointer to a char*, which means it points to an array of strings (or an array of char* pointers).
Example:
#include <stdio.h> int main() { char* strings[] = {"I love", "Embedded", "Systems"}; // Create a char** pointer to the strings array char** string_ptr = strings; // Access and print the strings using char** for (int i = 0; i <p><strong>Breakdown:</strong></p> <ul> <li>char* strings[]: Declares an array named strings, with each element pointing to a character (a char*), essentially forming an array of strings.</li> <li>{"I love", "Embedded", "Systems"}: Initializes the strings array with string literals stored in memory as character arrays. The compiler converts these literals into char* pointers, assigned to the array elements.</li> </ul> <p><strong>Visual Representation:</strong><br> </p> <pre class="brush:php;toolbar:false">Main Index (char**) → String 1 (char*) → "I love" → String 2 (char*) → "Embedded" → String 3 (char*) → "Systems"
Key Points:
- strings is an array of pointers to strings, not an array of characters.
- Each element of the array points to the first character of a string literal.
- You can manipulate individual characters within the strings using pointer arithmetic or array indexing.
Conclusion:
- char** is a pointer to an array of strings, much like a "table of strings."
- Memory is allocated separately for each string (row), allowing you to work with each string individually.
- Functions can modify the contents of the strings because char** passes a reference to the original array of pointers.
Working with char** is powerful when handling dynamic arrays, command-line arguments, or multi-dimensional arrays of strings in C/C . Once you understand its structure, it simplifies the process of managing arrays of strings in your programs.
The above is the detailed content of Understanding char** in C/C. For more information, please follow other related articles on the PHP Chinese website!
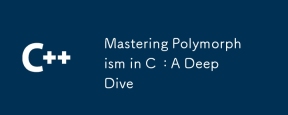
Mastering polymorphisms in C can significantly improve code flexibility and maintainability. 1) Polymorphism allows different types of objects to be treated as objects of the same base type. 2) Implement runtime polymorphism through inheritance and virtual functions. 3) Polymorphism supports code extension without modifying existing classes. 4) Using CRTP to implement compile-time polymorphism can improve performance. 5) Smart pointers help resource management. 6) The base class should have a virtual destructor. 7) Performance optimization requires code analysis first.
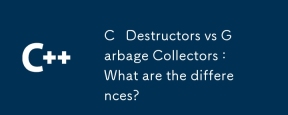
C destructorsprovideprecisecontroloverresourcemanagement,whilegarbagecollectorsautomatememorymanagementbutintroduceunpredictability.C destructors:1)Allowcustomcleanupactionswhenobjectsaredestroyed,2)Releaseresourcesimmediatelywhenobjectsgooutofscop
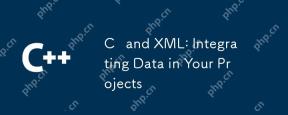
Integrating XML in a C project can be achieved through the following steps: 1) parse and generate XML files using pugixml or TinyXML library, 2) select DOM or SAX methods for parsing, 3) handle nested nodes and multi-level properties, 4) optimize performance using debugging techniques and best practices.
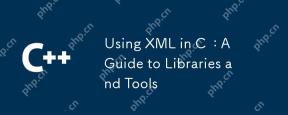
XML is used in C because it provides a convenient way to structure data, especially in configuration files, data storage and network communications. 1) Select the appropriate library, such as TinyXML, pugixml, RapidXML, and decide according to project needs. 2) Understand two ways of XML parsing and generation: DOM is suitable for frequent access and modification, and SAX is suitable for large files or streaming data. 3) When optimizing performance, TinyXML is suitable for small files, pugixml performs well in memory and speed, and RapidXML is excellent in processing large files.
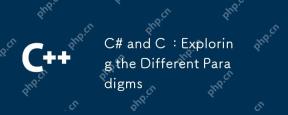
The main differences between C# and C are memory management, polymorphism implementation and performance optimization. 1) C# uses a garbage collector to automatically manage memory, while C needs to be managed manually. 2) C# realizes polymorphism through interfaces and virtual methods, and C uses virtual functions and pure virtual functions. 3) The performance optimization of C# depends on structure and parallel programming, while C is implemented through inline functions and multithreading.
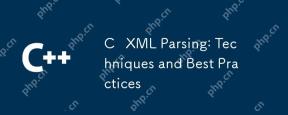
The DOM and SAX methods can be used to parse XML data in C. 1) DOM parsing loads XML into memory, suitable for small files, but may take up a lot of memory. 2) SAX parsing is event-driven and is suitable for large files, but cannot be accessed randomly. Choosing the right method and optimizing the code can improve efficiency.
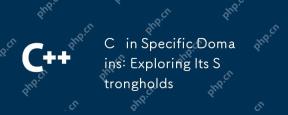
C is widely used in the fields of game development, embedded systems, financial transactions and scientific computing, due to its high performance and flexibility. 1) In game development, C is used for efficient graphics rendering and real-time computing. 2) In embedded systems, C's memory management and hardware control capabilities make it the first choice. 3) In the field of financial transactions, C's high performance meets the needs of real-time computing. 4) In scientific computing, C's efficient algorithm implementation and data processing capabilities are fully reflected.
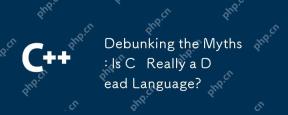
C is not dead, but has flourished in many key areas: 1) game development, 2) system programming, 3) high-performance computing, 4) browsers and network applications, C is still the mainstream choice, showing its strong vitality and application scenarios.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
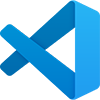
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

Notepad++7.3.1
Easy-to-use and free code editor

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
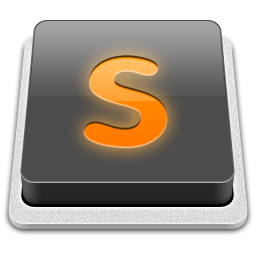
SublimeText3 Mac version
God-level code editing software (SublimeText3)
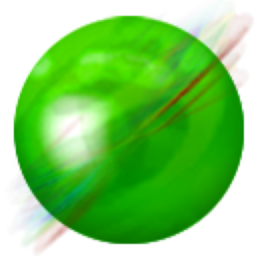
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
