Creation of an interface for character queues.
Three implementations to be developed:
Fixed size linear queue.
Circular queue (reuses array space).
Dynamic queue (grows as needed).
1 Create a file called ICharQ.java
// Character queue interface.
public interface ICharQ {
// Inserts a character into the queue.
void put(char ch);
// Remove a character from the queue.
char get();
}
2 Create a file called IQDemo.java.
3 Start creating IQDemo.java by adding the FixedQueue class shown here:
4 Add the CircularQueue class shown below to IQDemo.java.
Circular Queue Operation: Reuses space freed up in the array when removing elements. It can store an unlimited number of elements, as long as there are removals.
Boundary Conditions: The queue is not full when the end of the array is reached, but when an unremoved item is overwritten by a new one.
The put() method must check several conditions to determine whether the queue is full.Conditions for Full Queue: The queue is full if: putloc is a smaller unit than getloc. putloc is at the end of the array and getloc is at the beginning.
Empty Queue Condition: The queue is empty when getloc and putloc are equal.
Array Size: The underlying array is created one unit larger than the queue size to facilitate checks.
5 Insert the DynQueue class shown below in IQDemo.java. It implements an “extendable” queue that expands its size when space runs out.
- In this queue implementation, when the queue is full, an attempt to store another element makes a new underlying array twice as large as the original will be allocated, the current contents of the queue will be copied into this array and a reference to the new array will be stored in q.
6 To demonstrate the three implementations of ICharQ, insert the following class into IQDemo.java. It uses an ICharQ reference to access all queues.
class IQDemo {
public static void main(String args[]) {
FixedQueue q1 = new FixedQueue(10);
DynQueue q2 = new DynQueue(5);
CircularQueue q3 = new CircularQueue(10);
ICharQ iQ;
char ch;
int i;
iQ = q1;
// Inserts some characters into the fixed queue.
for(i=0; i
iQ.put((char) ('A' i));
// Displays the queue.
System.out.print("Contents of fixed queue: ");
for(i=0; i
ch = iQ.get();
System.out.print(ch);
}
System.out.println();
iQ = q2;
// Inserts some characters into the dynamic queue.
for(i=0; i
iQ.put((char) ('Z' - i));
// Displays the queue.
System.out.print("Contents of dynamic queue: ");
for(i=0; i
ch = iQ.get();
System.out.print(ch);
}
System.out.println();
iQ = q3;
// Inserts some characters into the circular queue.
for(i=0; i
iQ.put((char) ('A' i));
// Displays the queue.
System.out.print("Contents of circular queue: ");
for(i=0; i
ch = iQ.get();
System.out.print(ch);
}
System.out.println();
// Inserts more characters into the circular queue.
for(i=10; i
iQ.put((char) ('A' i));
// Displays the queue.
System.out.print("Contents of circular queue: ");
for(i=0; i
ch = iQ.get();
System.out.print(ch);
}
System.out.println("nStore and consume from"
" circular queue.");
// Stores and consumes items from the circular queue.
for(i=0; i
iQ.put((char) ('A' i));
ch = iQ.get();
System.out.print(ch);
}
}
}
7 Create a circular version of DynQueue. Add a reset( ) method to ICharQ that nets the queue. Create a static method that copies the contents of one queue type to another.
The above is the detailed content of Create a Queue interface. For more information, please follow other related articles on the PHP Chinese website!
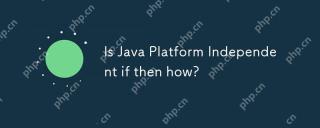
Java is platform-independent because of its "write once, run everywhere" design philosophy, which relies on Java virtual machines (JVMs) and bytecode. 1) Java code is compiled into bytecode, interpreted by the JVM or compiled on the fly locally. 2) Pay attention to library dependencies, performance differences and environment configuration. 3) Using standard libraries, cross-platform testing and version management is the best practice to ensure platform independence.
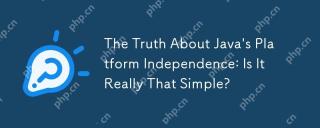
Java'splatformindependenceisnotsimple;itinvolvescomplexities.1)JVMcompatibilitymustbeensuredacrossplatforms.2)Nativelibrariesandsystemcallsneedcarefulhandling.3)Dependenciesandlibrariesrequirecross-platformcompatibility.4)Performanceoptimizationacros
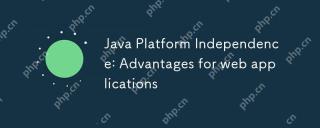
Java'splatformindependencebenefitswebapplicationsbyallowingcodetorunonanysystemwithaJVM,simplifyingdeploymentandscaling.Itenables:1)easydeploymentacrossdifferentservers,2)seamlessscalingacrosscloudplatforms,and3)consistentdevelopmenttodeploymentproce
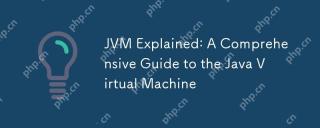
TheJVMistheruntimeenvironmentforexecutingJavabytecode,crucialforJava's"writeonce,runanywhere"capability.Itmanagesmemory,executesthreads,andensuressecurity,makingitessentialforJavadeveloperstounderstandforefficientandrobustapplicationdevelop
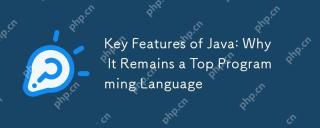
Javaremainsatopchoicefordevelopersduetoitsplatformindependence,object-orienteddesign,strongtyping,automaticmemorymanagement,andcomprehensivestandardlibrary.ThesefeaturesmakeJavaversatileandpowerful,suitableforawiderangeofapplications,despitesomechall
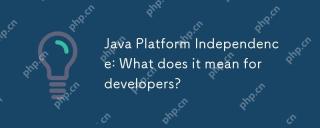
Java'splatformindependencemeansdeveloperscanwritecodeonceandrunitonanydevicewithoutrecompiling.ThisisachievedthroughtheJavaVirtualMachine(JVM),whichtranslatesbytecodeintomachine-specificinstructions,allowinguniversalcompatibilityacrossplatforms.Howev
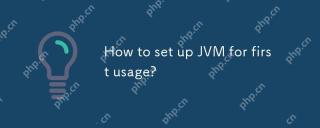
To set up the JVM, you need to follow the following steps: 1) Download and install the JDK, 2) Set environment variables, 3) Verify the installation, 4) Set the IDE, 5) Test the runner program. Setting up a JVM is not just about making it work, it also involves optimizing memory allocation, garbage collection, performance tuning, and error handling to ensure optimal operation.
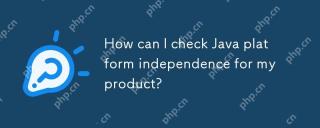
ToensureJavaplatformindependence,followthesesteps:1)CompileandrunyourapplicationonmultipleplatformsusingdifferentOSandJVMversions.2)UtilizeCI/CDpipelineslikeJenkinsorGitHubActionsforautomatedcross-platformtesting.3)Usecross-platformtestingframeworkss


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SublimeText3 Chinese version
Chinese version, very easy to use

SublimeText3 Linux new version
SublimeText3 Linux latest version
