What is Object Oriented Programming?
Object-oriented programming (OOP) is a programming paradigm that organizes code around "objects", which are instances of "classes".
This approach, inspired by the real world, allows systems to be modeled in a more intuitive and modular way.
Instead of thinking of a program as a sequence of instructions, OOP invites us to think in terms of objects that interact with each other. For example, in a game, we can have objects like "character", "enemy" and "item". Each object has its own characteristics (attributes) and behaviors (methods).
Fundamental Concepts
Classes and Objects
- Class: Think of them as a mold to create objects. Defines the attributes (characteristics) and methods (behaviors) that an object will have.
- Object: It is an instance of a class. Each object has its own values for attributes.
Attributes and Methods
- Attributes: These are the characteristics of an object. For example, a "Dog" object can have attributes such as "name", "breed" and "age".
- Methods: These are the actions that an object can perform. In the case of "Dog", methods could be "bark()", "run()" and "eat()".
Example in Java:
public class Cachorro { String nome; String raca; int idade; public void latir() { System.out.println("Au au!"); } public void correr() { System.out.println("Estou correndo!"); } } // Criando um objeto da classe Cachorro Cachorro meuCachorro = new Cachorro(); meuCachorro.nome = "Rex"; meuCachorro.raca = "Labrador"; meuCachorro.idade = 3; meuCachorro.latir(); meuCachorro.correr();
Why use POO?
Code reuse: Create base classes and inherit their characteristics to create new classes.
Maintenance: Makes it easier to find and correct errors.
Modularity: Divides the problem into smaller, more manageable parts.
Code organization: Improves code readability and understanding.
In short, OOP offers a more natural and organized way to model real-world problems, making software development more efficient and scalable.
In the next articles, we will explore:
- Encapsulation
- Inheritance
- Polymorphism
- Abstraction
- And much more!
The above is the detailed content of Introduction to Object-Oriented Programming: Thinking in Objects. For more information, please follow other related articles on the PHP Chinese website!
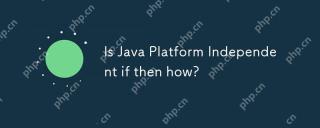
Java is platform-independent because of its "write once, run everywhere" design philosophy, which relies on Java virtual machines (JVMs) and bytecode. 1) Java code is compiled into bytecode, interpreted by the JVM or compiled on the fly locally. 2) Pay attention to library dependencies, performance differences and environment configuration. 3) Using standard libraries, cross-platform testing and version management is the best practice to ensure platform independence.
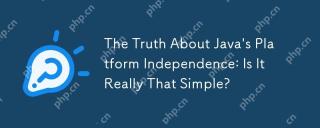
Java'splatformindependenceisnotsimple;itinvolvescomplexities.1)JVMcompatibilitymustbeensuredacrossplatforms.2)Nativelibrariesandsystemcallsneedcarefulhandling.3)Dependenciesandlibrariesrequirecross-platformcompatibility.4)Performanceoptimizationacros
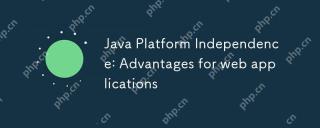
Java'splatformindependencebenefitswebapplicationsbyallowingcodetorunonanysystemwithaJVM,simplifyingdeploymentandscaling.Itenables:1)easydeploymentacrossdifferentservers,2)seamlessscalingacrosscloudplatforms,and3)consistentdevelopmenttodeploymentproce
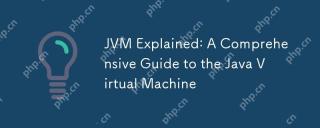
TheJVMistheruntimeenvironmentforexecutingJavabytecode,crucialforJava's"writeonce,runanywhere"capability.Itmanagesmemory,executesthreads,andensuressecurity,makingitessentialforJavadeveloperstounderstandforefficientandrobustapplicationdevelop
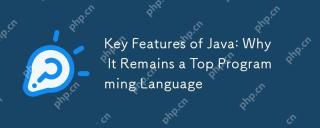
Javaremainsatopchoicefordevelopersduetoitsplatformindependence,object-orienteddesign,strongtyping,automaticmemorymanagement,andcomprehensivestandardlibrary.ThesefeaturesmakeJavaversatileandpowerful,suitableforawiderangeofapplications,despitesomechall
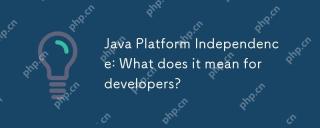
Java'splatformindependencemeansdeveloperscanwritecodeonceandrunitonanydevicewithoutrecompiling.ThisisachievedthroughtheJavaVirtualMachine(JVM),whichtranslatesbytecodeintomachine-specificinstructions,allowinguniversalcompatibilityacrossplatforms.Howev
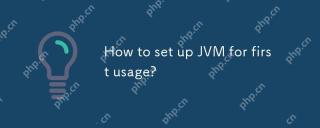
To set up the JVM, you need to follow the following steps: 1) Download and install the JDK, 2) Set environment variables, 3) Verify the installation, 4) Set the IDE, 5) Test the runner program. Setting up a JVM is not just about making it work, it also involves optimizing memory allocation, garbage collection, performance tuning, and error handling to ensure optimal operation.
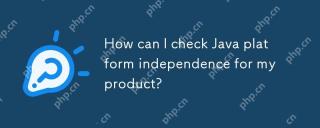
ToensureJavaplatformindependence,followthesesteps:1)CompileandrunyourapplicationonmultipleplatformsusingdifferentOSandJVMversions.2)UtilizeCI/CDpipelineslikeJenkinsorGitHubActionsforautomatedcross-platformtesting.3)Usecross-platformtestingframeworkss


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

Atom editor mac version download
The most popular open source editor
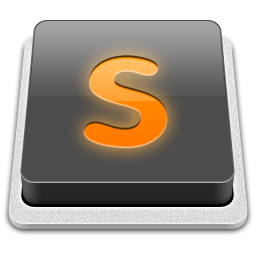
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SublimeText3 Chinese version
Chinese version, very easy to use
