Hello everyone, today I came to introduce you to a new unit testing library called sheepy, but first let's talk about the importance of unit testing. This library is not for beginners, to do unit testing with it you will need to pay a little extra attention. It has asserts only for API testing with endpoint and http error checking modules.
Github link: github
PyPi link: pypi
All mature, self-respecting software in production has unit tests, whether to get a sense of whether what was already in the code remains working, to prevent bugs that have already been reported and fixed before or to test new features, it is a good indication that things they are moving forward and not having accumulated technical debt. Let's use the Firefox browser as an example, every directory has a tests subdirectory, with specific tests for bugs that have already been reported, this way they guarantee that fixed bugs will not appear again out of nowhere, bugs that have already been fixed appear out of nowhere again It's called throwing money away. Over time you are losing time, money, efficiency and market share to a competitor who is doing better than you with fewer resources.
Everyone who feels incapable of doing something tries to defame that something, and unit tests are no different. To create better unit tests that cover each use case takes time, like everything in life, you backend I doubt you read just one tutorial and came out making perfect api's, the same thing for you front end, I doubt you watched a course and came out making interfaces perfect. So don't think that with unit tests it will be any different!
Assertion methods
+-----------------------+-------------------------------------------------------+ | Assertion Method | Description | +-----------------------+-------------------------------------------------------+ | assertEqual(a, b) | Checks if two values are equal. | | assertNotEqual(a, b) | Checks if two values are not equal. | | assertTrue(expr) | Verifies that the expression is True. | | assertFalse(expr) | Verifies that the expression is False. | | assertRaises(exc, fn) | Asserts that a function raises a specific exception. | | assertStatusCode(resp) | Verifies if the response has the expected status code.| | assertJsonResponse(resp)| Confirms the response is in JSON format. | | assertResponseContains(resp, key) | Ensures the response contains a given key. | +-----------------------+-------------------------------------------------------+
Installation
To install it is very simple, just open a terminal of your choice, with pip installed and type pip install sheepy
Usage example
from sheepy.sheeptest import SheepyTestCase class ExampleTest(SheepyTestCase): def test_success(self): self.assertTrue(True) def test_failure(self): self.assertEqual(1, 2) def test_error(self): raise Exception("Forced error") @SheepyTestCase.skip("Reason to ignore") def test_skipped(self): pass @SheepyTestCase.expectedFailure def test_expected_failure(self): self.assertEqual(1, 2)
The SheepyTestCase class provides several functionalities for creating and executing unit tests, including assertiveness methods and mechanisms for configuring special behaviors, such as skipping tests or handling expected failures.
Within the ExampleTest class, five test methods are defined:
test_success: This test checks whether the expression passed to the assertTrue method is true. Since the True value is explicitly passed, this test will succeed.
test_failure: This test checks equality between two values using the assertEqual method. However, the compared values, 1 and 2, are different, which results in a test failure. This demonstrates a case of expected failure, where the test must detect the inconsistency.
test_error: This method raises a purposeful exception with the message "Forced error". The goal is to test the system's behavior when dealing with errors that occur during test execution. As the method throws an exception without handling it, the result will be an error in the test.
test_skipped: This test has been decorated with the skip method of the SheepyTestCase class, which means that it will be skipped while running the tests. The reason for skipping the test was provided as "Reason to ignore", and this justification can be displayed in the final test report.
test_expected_failure: This method uses the expectedFailure decorator, indicating that a failure is expected. Inside the method, there is an equality check between 1 and 2, which would normally result in failure, but as the decorator was applied, the framework considers this failure to be part of the expected behavior and will not be treated as an error, but as a "expected failure".
Output
Test Results:
ExampleTest.test_error: FAIL - Forced error
ExampleTest.test_expected_failure: EXPECTED FAILURE
ExampleTest.test_failure: FAIL - 1 != 2
ExampleTest.test_skipped: SKIPPED -
ExampleTest.test_success: OK
Api test case
API testing in the Sheepy Test Framework is designed to be straightforward yet powerful, allowing testers to interact with APIs using common HTTP methods such as GET, POST, PUT, and DELETE. The framework provides a dedicated class, ApiRequests, to simplify sending requests and handling responses, with built-in error management via the HttpError exception class.
When testing an API, the test class inherits from SheepyTestCase, which is equipped with various assertion methods to verify the behavior of the API. These include assertStatusCode to validate HTTP status codes, assertJsonResponse to ensure the response is in JSON format, and assertResponseContains to check if specific keys exist in the response body.
For instance, the framework allows you to send a POST request to an API, verify that the status code matches the expected value, and assert that the JSON response contains the correct data. The API requests are handled through the ApiRequests class, which takes care of constructing and sending the requests, while error handling is streamlined by raising HTTP-specific errors when the server returns unexpected status codes.
By providing built-in assertions and error handling, the framework automates much of the repetitive tasks in API testing, ensuring both correctness and simplicity in writing tests. This system allows developers to focus on verifying API behavior and logic, making it an efficient tool for ensuring the reliability of API interactions.
from sheepy.sheeptest import SheepyTestCase class TestHttpBinApi(SheepyTestCase): def __init__(self): super().__init__(base_url="https://httpbin.org") def test_get_status(self): response = self.api.get("/status/200") self.assertStatusCode(response, 200) def test_get_json(self): response = self.api.get("/json") self.assertStatusCode(response, 200) self.assertJsonResponse(response) self.assertResponseContains(response, "slideshow") def test_post_data(self): payload = {"name": "SheepyTest", "framework": "unittest"} response = self.api.post("/post", json=payload) self.assertStatusCode(response, 200) self.assertJsonResponse(response) self.assertResponseContains(response, "json") self.assertEqual(response.json()["json"], payload) def test_put_data(self): payload = {"key": "value"} response = self.api.put("/put", json=payload) self.assertStatusCode(response, 200) self.assertJsonResponse(response) self.assertResponseContains(response, "json") self.assertEqual(response.json()["json"], payload) def test_delete_resource(self): response = self.api.delete("/delete") self.assertStatusCode(response, 200) self.assertJsonResponse(response)
Output example
Test Results: TestHttpBinApi.test_delete_resource: OK TestHttpBinApi.test_get_json: OK TestHttpBinApi.test_get_status: OK TestHttpBinApi.test_post_data: OK TestHttpBinApi.test_put_data: OK
Summary:
The new sheepy library is an incredible unit testing library, which has several test accession methods, including a module just for API testing, in my opinion, it is not a library for beginners, it requires basic knowledge of object-oriented programming such as methods, classes and inheritance.
The above is the detailed content of Unit testing in Python with sheepy. For more information, please follow other related articles on the PHP Chinese website!
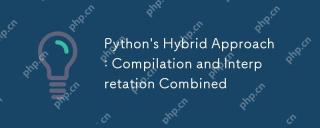
Pythonusesahybridapproach,combiningcompilationtobytecodeandinterpretation.1)Codeiscompiledtoplatform-independentbytecode.2)BytecodeisinterpretedbythePythonVirtualMachine,enhancingefficiencyandportability.
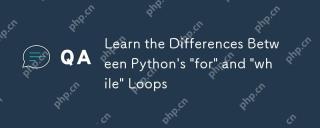
ThekeydifferencesbetweenPython's"for"and"while"loopsare:1)"For"loopsareidealforiteratingoversequencesorknowniterations,while2)"while"loopsarebetterforcontinuinguntilaconditionismetwithoutpredefinediterations.Un
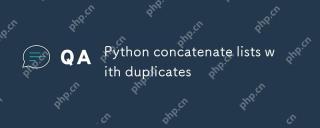
In Python, you can connect lists and manage duplicate elements through a variety of methods: 1) Use operators or extend() to retain all duplicate elements; 2) Convert to sets and then return to lists to remove all duplicate elements, but the original order will be lost; 3) Use loops or list comprehensions to combine sets to remove duplicate elements and maintain the original order.
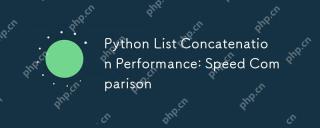
ThefastestmethodforlistconcatenationinPythondependsonlistsize:1)Forsmalllists,the operatorisefficient.2)Forlargerlists,list.extend()orlistcomprehensionisfaster,withextend()beingmorememory-efficientbymodifyinglistsin-place.
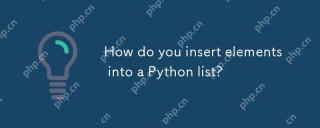
ToinsertelementsintoaPythonlist,useappend()toaddtotheend,insert()foraspecificposition,andextend()formultipleelements.1)Useappend()foraddingsingleitemstotheend.2)Useinsert()toaddataspecificindex,thoughit'sslowerforlargelists.3)Useextend()toaddmultiple
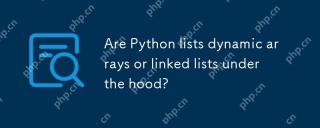
Pythonlistsareimplementedasdynamicarrays,notlinkedlists.1)Theyarestoredincontiguousmemoryblocks,whichmayrequirereallocationwhenappendingitems,impactingperformance.2)Linkedlistswouldofferefficientinsertions/deletionsbutslowerindexedaccess,leadingPytho
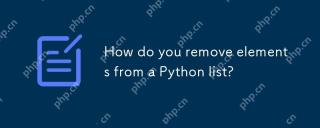
Pythonoffersfourmainmethodstoremoveelementsfromalist:1)remove(value)removesthefirstoccurrenceofavalue,2)pop(index)removesandreturnsanelementataspecifiedindex,3)delstatementremoveselementsbyindexorslice,and4)clear()removesallitemsfromthelist.Eachmetho
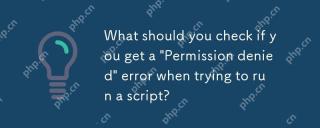
Toresolvea"Permissiondenied"errorwhenrunningascript,followthesesteps:1)Checkandadjustthescript'spermissionsusingchmod xmyscript.shtomakeitexecutable.2)Ensurethescriptislocatedinadirectorywhereyouhavewritepermissions,suchasyourhomedirectory.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
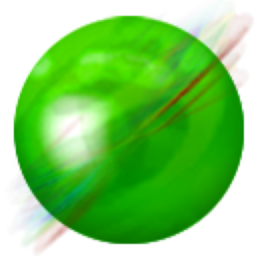
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
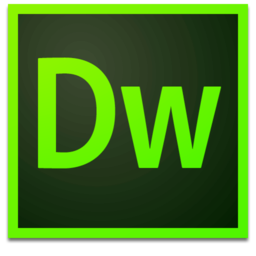
Dreamweaver Mac version
Visual web development tools
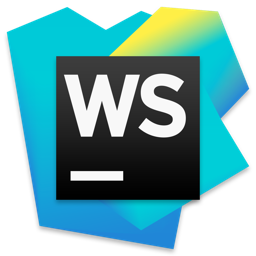
WebStorm Mac version
Useful JavaScript development tools
