Streams are a powerful feature in Node.js that allows the handling of large amounts of data efficiently by processing it piece by piece, rather than loading everything into memory at once. They are especially useful for dealing with large files, real-time data, or even network connections. In this article, we'll dive deep into Node.js streams, covering the types of streams, how to use them with code examples, and a real-world use case to solidify your understanding.
What are Streams?
A stream is a sequence of data that is processed over time. In Node.js, streams are instances of EventEmitter, which means they can emit and respond to events. Streams allow data to be read and written in chunks (small pieces) rather than loaded all of the data at once, which makes them memory-efficient and faster.
Why Use Streams?
- Efficient Memory Usage: Streams process data as it comes in chunks, without having to load the entire data set into memory.
- Faster Processing: They begin processing data as soon as it is available, rather than waiting for everything to load.
- Non-blocking I/O: Since streams operate asynchronously, they don't block other operations, making them ideal for real-time applications.
Types of Streams
Node.js provides four types of streams:
- Readable Streams: Used to read data sequentially.
- Writable Streams: Used to write data sequentially.
- Duplex Streams: Can be both readable and writable.
- Transform Streams: A duplex stream where the output is a transformation of the input.
Let's explore each type of stream with examples.
Readable Streams
A readable stream lets you consume data, chunk by chunk, from a source such as a file or network request.
Example: Reading a file using a readable stream
const fs = require('fs'); // Create a readable stream const readableStream = fs.createReadStream('example.txt', 'utf8'); // Listen for 'data' events to read chunks of data readableStream.on('data', (chunk) => { console.log('New chunk received:'); console.log(chunk); }); // Handle 'end' event when the file has been completely read readableStream.on('end', () => { console.log('File reading completed.'); }); // Handle any errors readableStream.on('error', (err) => { console.error('Error reading file:', err.message); });
Explanation:
- fs.createReadStream() creates a stream to read the contents of example.txt.
- The stream emits 'data' events for each chunk it reads, and 'end' event when it finishes reading.
Writable Streams
Writable streams are used to write data chunk by chunk, such as saving data to a file.
Example: Writing data to a file using a writable stream
const fs = require('fs'); // Create a writable stream const writableStream = fs.createWriteStream('output.txt'); // Write chunks of data to the file writableStream.write('First chunk of data.\n'); writableStream.write('Second chunk of data.\n'); // End the stream writableStream.end('Final chunk of data.'); // Handle 'finish' event when writing is complete writableStream.on('finish', () => { console.log('Data writing completed.'); }); // Handle any errors writableStream.on('error', (err) => { console.error('Error writing to file:', err.message); });
Explanation:
- fs.createWriteStream() creates a writable stream to write to output.txt.
- The write() method is used to send chunks of data to the stream. Once all data is written, the end() method is called, signalling the stream to finish.
Duplex Streams
Duplex streams can both read and write data, and are used for operations like network protocols where you need to send and receive data.
Example: Custom Duplex Stream
const { Duplex } = require('stream'); // Create a custom duplex stream const myDuplexStream = new Duplex({ read(size) { this.push('Reading data...'); this.push(null); // No more data to read }, write(chunk, encoding, callback) { console.log(`Writing: ${chunk.toString()}`); callback(); } }); // Read from the stream myDuplexStream.on('data', (chunk) => { console.log(chunk.toString()); }); // Write to the stream myDuplexStream.write('This is a test.'); myDuplexStream.end();
Explanation:
- Duplex streams can perform both read and write operations. In the example, we define custom read and write methods for the duplex stream.
Transform Streams
Transform streams allow you to modify or transform the data as it passes through. They're a special type of duplex stream.
Example: A simple transform stream to uppercase text
const { Transform } = require('stream'); // Create a custom transform stream const toUpperCaseTransform = new Transform({ transform(chunk, encoding, callback) { this.push(chunk.toString().toUpperCase()); callback(); } }); // Pipe data through the transform stream process.stdin.pipe(toUpperCaseTransform).pipe(process.stdout);
Explanation:
- Transform streams take input, process it (in this case, converting text to uppercase), and output the modified data.
- In this example, data is piped from standard input (process.stdin) through the transform stream, and the result is outputted to the console (process.stdout).
Piping Streams
One of the most common ways to work with streams is to "pipe" them together. This means passing data from one stream to another. This is useful when you need to process data step by step, such as reading from a file and writing to another file.
Example: Piping a readable stream to a writable stream
const fs = require('fs'); // Create a readable stream const readableStream = fs.createReadStream('input.txt'); // Create a writable stream const writableStream = fs.createWriteStream('output.txt'); // Pipe the readable stream into the writable stream readableStream.pipe(writableStream); // Handle 'finish' event when piping is done writableStream.on('finish', () => { console.log('File copied successfully.'); });
Explanation:
- The pipe() method passes data from the readable stream (input.txt) directly to the writable stream (output.txt).
Real-World Use Case: Streaming a Large File Upload
In real-world applications, you might need to upload large files to the server. Instead of loading the entire file into memory, you can use streams to handle file uploads efficiently.
Example: Uploading a file using streams with Node.js and multer
const express = require('express'); const multer = require('multer'); const fs = require('fs'); const app = express(); const upload = multer({ dest: 'uploads/' }); app.post('/upload', upload.single('file'), (req, res) => { const readableStream = fs.createReadStream(req.file.path); const writableStream = fs.createWriteStream(`./uploads/${req.file.originalname}`); // Pipe the uploaded file to the writable stream readableStream.pipe(writableStream); writableStream.on('finish', () => { res.send('File uploaded and saved.'); }); writableStream.on('error', (err) => { res.status(500).send('Error saving file.'); }); }); app.listen(3000, () => { console.log('Server is running on port 3000'); });
Explanation:
- We use multer to handle file uploads. When the file is uploaded, it is piped from a temporary location to the desired directory on the server.
- This method is efficient as it streams the file data instead of holding it all in memory at once.
Best Practices for Working with Streams
- Error Handling: Always handle errors in streams to avoid unhandled exceptions, especially when dealing with file systems or network operations.
Example:
readableStream.on('error', (err) => { console.error('Stream error:', err.message); });
- Flow Control: Be mindful of flow control when reading and writing data, as writable streams can become overwhelmed if data is being written faster than it can be consumed.
Example:
writableStream.write(chunk, (err) => { if (err) console.error('Error writing chunk:', err.message); });
- Use Pipe for Simplicity: When transferring data between streams, always prefer using pipe() instead of manually managing the flow of data.
Conclusion
Streams in Node.js offer a powerful and efficient way to handle data, especially in cases where data comes in large quantities or needs to be processed incrementally. From reading and writing files to handling network requests and processing data in real time, streams allow you to build scalable and performant applications. In this article, we explored the different types of streams, how to use them, and real-world use cases to deepen your understanding of stream-based processing in Node.js.
The above is the detailed content of Understanding Streams in Node.js — Efficient Data Handling. For more information, please follow other related articles on the PHP Chinese website!
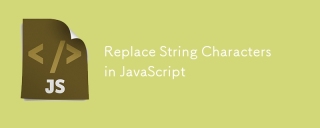
Detailed explanation of JavaScript string replacement method and FAQ This article will explore two ways to replace string characters in JavaScript: internal JavaScript code and internal HTML for web pages. Replace string inside JavaScript code The most direct way is to use the replace() method: str = str.replace("find","replace"); This method replaces only the first match. To replace all matches, use a regular expression and add the global flag g: str = str.replace(/fi
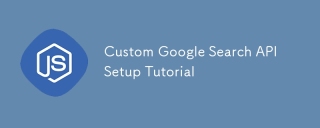
This tutorial shows you how to integrate a custom Google Search API into your blog or website, offering a more refined search experience than standard WordPress theme search functions. It's surprisingly easy! You'll be able to restrict searches to y
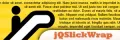
Leverage jQuery for Effortless Web Page Layouts: 8 Essential Plugins jQuery simplifies web page layout significantly. This article highlights eight powerful jQuery plugins that streamline the process, particularly useful for manual website creation
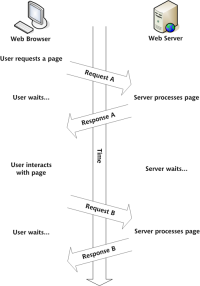
So here you are, ready to learn all about this thing called AJAX. But, what exactly is it? The term AJAX refers to a loose grouping of technologies that are used to create dynamic, interactive web content. The term AJAX, originally coined by Jesse J
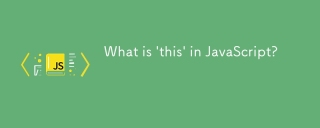
Core points This in JavaScript usually refers to an object that "owns" the method, but it depends on how the function is called. When there is no current object, this refers to the global object. In a web browser, it is represented by window. When calling a function, this maintains the global object; but when calling an object constructor or any of its methods, this refers to an instance of the object. You can change the context of this using methods such as call(), apply(), and bind(). These methods call the function using the given this value and parameters. JavaScript is an excellent programming language. A few years ago, this sentence was
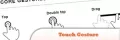
This post compiles helpful cheat sheets, reference guides, quick recipes, and code snippets for Android, Blackberry, and iPhone app development. No developer should be without them! Touch Gesture Reference Guide (PDF) A valuable resource for desig

jQuery is a great JavaScript framework. However, as with any library, sometimes it’s necessary to get under the hood to discover what’s going on. Perhaps it’s because you’re tracing a bug or are just curious about how jQuery achieves a particular UI
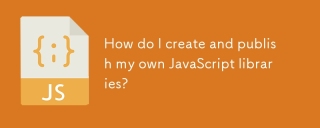
Article discusses creating, publishing, and maintaining JavaScript libraries, focusing on planning, development, testing, documentation, and promotion strategies.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
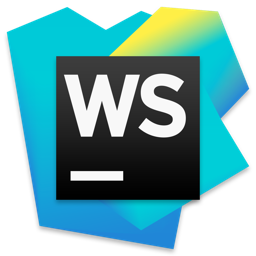
WebStorm Mac version
Useful JavaScript development tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
