Here is the draft for Part 5: Testing Strategies for Redux Toolkit and RTK Query. This part will cover the best practices for testing Redux Toolkit and RTK Query, focusing on unit testing, integration testing, and ensuring your code is robust and maintainable.
Part 5: Testing Strategies for Redux Toolkit and RTK Query
1. Importance of Testing in Redux Applications
Testing is a crucial aspect of any application development process. It ensures that your application behaves as expected, helps catch bugs early, and provides confidence when making changes. With Redux Toolkit (RTK) and RTK Query, testing becomes more manageable due to their simplified APIs and reduced boilerplate. In this part, we will explore different testing strategies to ensure Redux applications are reliable and maintainable.
2. Setting Up a Testing Environment
Before diving into specific testing strategies, make sure you have a proper testing environment set up. For a typical React Redux Toolkit project, you might use tools like:
- Jest: A popular testing framework for JavaScript.
- React Testing Library (RTL): A lightweight solution for testing React components.
- MSW (Mock Service Worker): A library for mocking API requests during testing.
To install these libraries, run:
npm install --save-dev jest @testing-library/react @testing-library/jest-dom msw
3. Unit Testing Redux Slices
Redux slices are the core of state management in Redux Toolkit. Unit testing these slices ensures that reducers and actions work correctly.
Step 1: Testing Reducers
Consider the following postsSlice.js:
// src/features/posts/postsSlice.js import { createSlice } from '@reduxjs/toolkit'; const initialState = { posts: [], status: 'idle', error: null, }; const postsSlice = createSlice({ name: 'posts', initialState, reducers: { addPost: (state, action) => { state.posts.push(action.payload); }, removePost: (state, action) => { state.posts = state.posts.filter(post => post.id !== action.payload); }, }, }); export const { addPost, removePost } = postsSlice.actions; export default postsSlice.reducer;
Unit Tests for postsSlice Reducer:
// src/features/posts/postsSlice.test.js import postsReducer, { addPost, removePost } from './postsSlice'; describe('postsSlice reducer', () => { const initialState = { posts: [], status: 'idle', error: null }; it('should handle initial state', () => { expect(postsReducer(undefined, {})).toEqual(initialState); }); it('should handle addPost', () => { const newPost = { id: 1, title: 'New Post' }; const expectedState = { ...initialState, posts: [newPost] }; expect(postsReducer(initialState, addPost(newPost))).toEqual(expectedState); }); it('should handle removePost', () => { const initialStateWithPosts = { ...initialState, posts: [{ id: 1, title: 'New Post' }] }; const expectedState = { ...initialState, posts: [] }; expect(postsReducer(initialStateWithPosts, removePost(1))).toEqual(expectedState); }); });
Explanation:
- Initial State Test: Validates that the reducer returns the correct initial state.
- Action Tests: Tests addPost and removePost actions to ensure they modify the state correctly.
4. Testing RTK Query API Slices
RTK Query simplifies API integration, but testing these API slices is slightly different from testing regular slices. You need to mock API requests and validate how the slice handles those requests.
Step 1: Setting Up MSW for Mocking API Requests
Create a setupTests.js file to configure MSW:
// src/setupTests.js import { setupServer } from 'msw/node'; import { rest } from 'msw'; const server = setupServer( // Mocking GET /posts endpoint rest.get('https://jsonplaceholder.typicode.com/posts', (req, res, ctx) => { return res(ctx.json([{ id: 1, title: 'Mock Post' }])); }), // Mocking POST /posts endpoint rest.post('https://jsonplaceholder.typicode.com/posts', (req, res, ctx) => { return res(ctx.json({ id: 2, ...req.body })); }) ); // Establish API mocking before all tests beforeAll(() => server.listen()); // Reset any request handlers that are declared as a part of our tests (i.e. for testing one-time errors) afterEach(() => server.resetHandlers()); // Clean up after the tests are finished afterAll(() => server.close());
Step 2: Writing Tests for RTK Query Endpoints
Testing the fetchPosts query from postsApi.js:
// src/features/posts/postsApi.test.js import { renderHook } from '@testing-library/react-hooks'; import { Provider } from 'react-redux'; import { setupApiStore } from '../../testUtils'; import { postsApi, useFetchPostsQuery } from './postsApi'; import store from '../../app/store'; describe('RTK Query: postsApi', () => { it('fetches posts successfully', async () => { const { result, waitForNextUpdate } = renderHook(() => useFetchPostsQuery(), { wrapper: ({ children }) => <provider store="{store}">{children}</provider>, }); await waitForNextUpdate(); expect(result.current.data).toEqual([{ id: 1, title: 'Mock Post' }]); expect(result.current.isLoading).toBeFalsy(); }); });
Explanation:
- MSW Setup: setupServer configures MSW to mock API endpoints.
- Render Hook: The renderHook utility from React Testing Library is used to test custom hooks, such as useFetchPostsQuery.
- Mocked API Response: Validates the behavior of the hook with mocked API responses.
5. Integration Testing Redux with Components
Integration tests focus on testing how different pieces work together. In Redux applications, this often means testing components that interact with the Redux store.
Step 1: Writing Integration Tests with React Testing Library
Integration test for the PostsList component:
// src/features/posts/PostsList.test.js import React from 'react'; import { render, screen, waitFor } from '@testing-library/react'; import { Provider } from 'react-redux'; import store from '../../app/store'; import PostsList from './PostsList'; test('renders posts fetched from the API', async () => { render( <provider store="{store}"> <postslist></postslist> </provider> ); expect(screen.getByText(/Loading.../i)).toBeInTheDocument(); // Wait for posts to be fetched and rendered await waitFor(() => { expect(screen.getByText(/Mock Post/i)).toBeInTheDocument(); }); });
Explanation:
- Loading State Test: Checks that the loading indicator is displayed initially.
- Data Render Test: Waits for the posts to be fetched and ensures they are rendered correctly.
6. Best Practices for Testing Redux Toolkit and RTK Query
- Use MSW for API Mocking: MSW is a powerful tool for mocking network requests and simulating different scenarios, such as network errors or slow responses.
- Test Reducers in Isolation: Reducer tests are pure functions and should be tested separately to ensure they handle actions correctly.
- Test Hooks in Isolation: Use renderHook from React Testing Library to test hooks independently from UI components.
- Use setupApiStore for API Slice Testing: When testing RTK Query endpoints, use setupApiStore to mock the Redux store with API slices.
- Focus on Integration Tests for Components: Combine slice and component tests for integration testing to ensure they work together correctly.
- Ensure Coverage for Edge Cases: Test edge cases, such as API errors, empty states, and invalid data, to ensure robustness.
7. Conclusion
In this part, we covered various testing strategies for Redux Toolkit and RTK Query, including unit testing reducers and slices, testing RTK Query API slices with MSW, and writing integration tests for components interacting with the Redux store. By following these best practices, you can ensure that your Redux applications are robust, reliable, and maintainable.
This concludes our series on Redux Toolkit and RTK Query! I hope these parts have helped you understand Redux Toolkit from the basics to advanced topics, including effective testing strategies.
Happy coding, and keep testing to ensure your apps are always in top shape!
The above is the detailed content of Complete redux toolkit (Part -5). For more information, please follow other related articles on the PHP Chinese website!
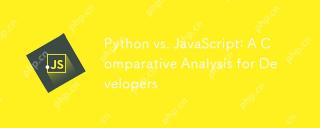
The main difference between Python and JavaScript is the type system and application scenarios. 1. Python uses dynamic types, suitable for scientific computing and data analysis. 2. JavaScript adopts weak types and is widely used in front-end and full-stack development. The two have their own advantages in asynchronous programming and performance optimization, and should be decided according to project requirements when choosing.
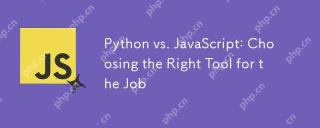
Whether to choose Python or JavaScript depends on the project type: 1) Choose Python for data science and automation tasks; 2) Choose JavaScript for front-end and full-stack development. Python is favored for its powerful library in data processing and automation, while JavaScript is indispensable for its advantages in web interaction and full-stack development.
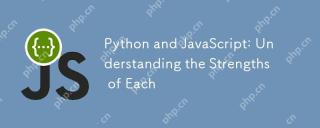
Python and JavaScript each have their own advantages, and the choice depends on project needs and personal preferences. 1. Python is easy to learn, with concise syntax, suitable for data science and back-end development, but has a slow execution speed. 2. JavaScript is everywhere in front-end development and has strong asynchronous programming capabilities. Node.js makes it suitable for full-stack development, but the syntax may be complex and error-prone.
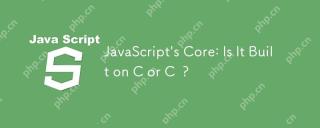
JavaScriptisnotbuiltonCorC ;it'saninterpretedlanguagethatrunsonenginesoftenwritteninC .1)JavaScriptwasdesignedasalightweight,interpretedlanguageforwebbrowsers.2)EnginesevolvedfromsimpleinterpreterstoJITcompilers,typicallyinC ,improvingperformance.
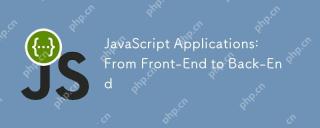
JavaScript can be used for front-end and back-end development. The front-end enhances the user experience through DOM operations, and the back-end handles server tasks through Node.js. 1. Front-end example: Change the content of the web page text. 2. Backend example: Create a Node.js server.
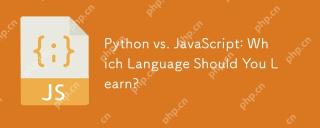
Choosing Python or JavaScript should be based on career development, learning curve and ecosystem: 1) Career development: Python is suitable for data science and back-end development, while JavaScript is suitable for front-end and full-stack development. 2) Learning curve: Python syntax is concise and suitable for beginners; JavaScript syntax is flexible. 3) Ecosystem: Python has rich scientific computing libraries, and JavaScript has a powerful front-end framework.
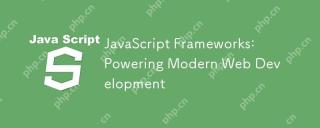
The power of the JavaScript framework lies in simplifying development, improving user experience and application performance. When choosing a framework, consider: 1. Project size and complexity, 2. Team experience, 3. Ecosystem and community support.
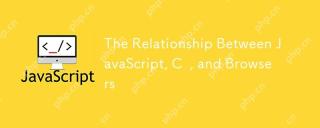
Introduction I know you may find it strange, what exactly does JavaScript, C and browser have to do? They seem to be unrelated, but in fact, they play a very important role in modern web development. Today we will discuss the close connection between these three. Through this article, you will learn how JavaScript runs in the browser, the role of C in the browser engine, and how they work together to drive rendering and interaction of web pages. We all know the relationship between JavaScript and browser. JavaScript is the core language of front-end development. It runs directly in the browser, making web pages vivid and interesting. Have you ever wondered why JavaScr


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
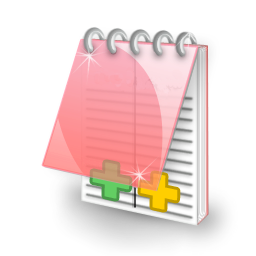
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
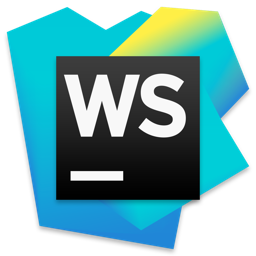
WebStorm Mac version
Useful JavaScript development tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

Zend Studio 13.0.1
Powerful PHP integrated development environment
