ListBox in C# is defined as adding a list of elements to the ListBox to operate on single or multiple elements. The difference between the drop-down box and the list box is drop-down box can select only one element at a time, but in the case of the list box, we can select single or multiple elements at a time. The ListBox provides different types of methods, properties, and events. This ListBox is specified under System. Windows.Forms package (namespace).
The ListBox class again contains 3 different types of collections in C#. They are
- ListBox.ObjectCollection: This collection class holds all the elements of the ListBox control.
- ListBox.SelectedObjectCollection: This collection class holds the collection of selected items in the ListBox control.
- ListBox.SelectedIndexCollection: This collection class holds the collection of selected indexes; these elements are a subset of the indexes of the ListBox.ObjectCollection and this specifically selected indexes in the ListBox control.
Types of List Boxes in C#?
- Single Selected ListBox: ListBox can be able to select only a single element from the list.
- Multi Selected ListBox: ListBox can be able to select multiple elements from the list.
Prerequisites for ListBox in C#:
- .Net libraries must be installed on your PC
- Visual Studio set up
How to Create the ListBox in C#?
ListBox can be created in 2 ways:
- Design-Time
- Run-Time
1. Design-Time
It is very easy to create without any code initially. Steps to create a project
Step 1: Open Visual Studio
Click on File=>New=>Project
Select =>Windows Form Application, then
See the below image for a better understanding of the project structure:
Name the project and click OK then you will get Form1.cs(Design) tab like below
Step 2: Left side of the visual studio or From View, choose Toolbox; next, drag and drop then the required elements onto the Form1.cs(Design) as shown in the above image.
Step 3: After drag and drop select the properties from the right side of the Visual Studio and give some name to the Text property. This is used to write code in the 2nd method Run-Time.
Output:
2. Run-Time
This is not directly doing it as per the above method. We have written some programs to create ListBox. This is very simple; first, drag and drop all the required elements like ListBox, Label, TextField, Button, etc. If you double click on any of the dropped elements, we get some C# code that element action methods, we have to write our logic to what we want to do with those elements. Steps to create Run-Time project code to create ListBox
Step 1: Create ListBox control by using ListBox() constructor.
Syntax:
ListBox listBox = new ListBox();
Step 2: After creating the ListBox property, if we want to set the properties of the ListBox like Font, Font.Size, Color to elements, etc.
Syntax:
listBox.Location = new Point(200, 100); listBox.Size = new Size(100, 90); listBox.ForeColor = Color.Red;
Step 3: Add the elements to the ListBox.
Syntax:
listBox.Items.Add("A"); listBox.Items.Add("B"); listBox.Items.Add("C"); listBox.Items.Add("D");
Step 4: Add this ListBox to the Form.
Syntax:
this.Controls.Add(listBox);
Examples of Listbox in C#
Here are the following examples mentioned below
Example #1 – Creating ListBox and adding elements
Code:
//importing C# required libraries using System; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.Drawing; using System.Linq; using System.Text; using System.Windows.Forms; //namespace is project name namespace WindowsFormsApplication26 { //creating class extends from Form class public partial class Form1 : Form { //constrcutor public Form1() { //initializing components InitializeComponent(); //Creating list box and add some properties and values to the List Box listBox2.ForeColor = Color.Red; listBox2.Items.Add("Java"); listBox2.Items.Add("Python"); listBox2.Items.Add("C++"); listBox2.Items.Add("C"); listBox2.Items.Add("C#"); listBox2.Items.Add("Spring"); listBox2.Items.Add("JavaFX"); listBox2.SelectionMode = SelectionMode.MultiSimple; } //method for selectedIndex change operation private void listBox2_SelectedIndexChanged(object sender, EventArgs e) { } } }
Output:
Example #2 – User enters value added it to the list box by clicking the button
Code:
//importing C# required libraries using System; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.Drawing; using System.Linq; using System.Text; using System.Windows.Forms; //namespace is project name namespace WindowsFormsApp25 { //creating class extends from Form class public partial class Form1 : Form { //constrcutor public Form1() { //initializing components InitializeComponent(); } //saving the enter values into List box private void buttonSave_Click(object sender, EventArgs e) { //If user enter any values then if block executes if (this.textBoxName.Text != "") { NameList.Items.Add(this.textBoxName.Text); this.textBoxName.Focus(); this.textBoxName.Clear(); } //If user did not enter any values then else block executes else { MessageBox.Show("Please enter a name to add..","Error",MessageBoxButtons.OK,MessageBoxIcon.Information); this.textBoxName.Focus(); } } } }
Output:
Before entering a value:
Without entering any value try to click the save button:
After entering a value:
After entering a value and clicking the save button:
Example #3 – Delete, Change the font of List Box values
Code:
//importing C# required libraries using System; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.Drawing; using System.Linq; using System.Text; using System.Windows.Forms; //namespace is project name namespace WindowsFormsApp25 { //creating class extends from Form class public partial class Form1 : Form { //constrcutor public Form1() { //initializing components InitializeComponent(); } //saving the enter values into List box private void buttonSave_Click(object sender, EventArgs e) { //If user enter any values then if block executes if (this.textBoxName.Text != "") { NameList.Items.Add(this.textBoxName.Text); this.textBoxName.Focus(); this.textBoxName.Clear(); } //If user did not enter any values then else block executes else { MessageBox.Show("Please enter a name to add..","Error",MessageBoxButtons.OK,MessageBoxIcon.Information); this.textBoxName.Focus(); } } //Removing the selected elements private void button2_Click(object sender, EventArgs e) { if (this.NameList.SelectedIndex >= 0) { this.NameList.Items.RemoveAt(this.NameList.SelectedIndex); } } //Setting List box selected values font private void button3_Click(object sender, EventArgs e) { if (fontDialog1.ShowDialog() == DialogResult.OK) { NameList.Font = fontDialog1.Font; } } } }
Output:
After adding 3 names:
Deleting selected element:
Change the font of the values:
Conclusion
C# List box is used to add multiple elements to perform any specific operation. List Boxes are used to select a single value or multiple values at a time. In C# List Box can be created using Design-Time and Run-Time methods.
The above is the detailed content of Listbox in C#. For more information, please follow other related articles on the PHP Chinese website!
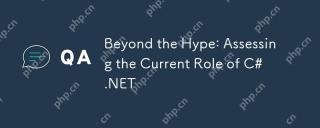
C#.NET is a powerful development platform that combines the advantages of the C# language and .NET framework. 1) It is widely used in enterprise applications, web development, game development and mobile application development. 2) C# code is compiled into an intermediate language and is executed by the .NET runtime environment, supporting garbage collection, type safety and LINQ queries. 3) Examples of usage include basic console output and advanced LINQ queries. 4) Common errors such as empty references and type conversion errors can be solved through debuggers and logging. 5) Performance optimization suggestions include asynchronous programming and optimization of LINQ queries. 6) Despite the competition, C#.NET maintains its important position through continuous innovation.
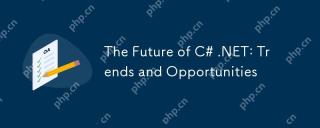
The future trends of C#.NET are mainly focused on three aspects: cloud computing, microservices, AI and machine learning integration, and cross-platform development. 1) Cloud computing and microservices: C#.NET optimizes cloud environment performance through the Azure platform and supports the construction of an efficient microservice architecture. 2) Integration of AI and machine learning: With the help of the ML.NET library, C# developers can embed machine learning models in their applications to promote the development of intelligent applications. 3) Cross-platform development: Through .NETCore and .NET5, C# applications can run on Windows, Linux and macOS, expanding the deployment scope.
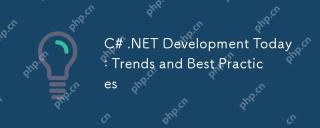
The latest developments and best practices in C#.NET development include: 1. Asynchronous programming improves application responsiveness, and simplifies non-blocking code using async and await keywords; 2. LINQ provides powerful query functions, efficiently manipulating data through delayed execution and expression trees; 3. Performance optimization suggestions include using asynchronous programming, optimizing LINQ queries, rationally managing memory, improving code readability and maintenance, and writing unit tests.
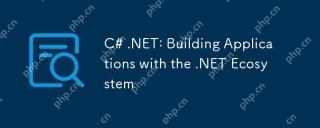
How to build applications using .NET? Building applications using .NET can be achieved through the following steps: 1) Understand the basics of .NET, including C# language and cross-platform development support; 2) Learn core concepts such as components and working principles of the .NET ecosystem; 3) Master basic and advanced usage, from simple console applications to complex WebAPIs and database operations; 4) Be familiar with common errors and debugging techniques, such as configuration and database connection issues; 5) Application performance optimization and best practices, such as asynchronous programming and caching.
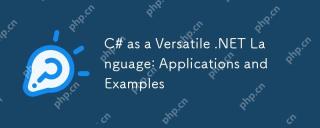
C# is widely used in enterprise-level applications, game development, mobile applications and web development. 1) In enterprise-level applications, C# is often used for ASP.NETCore to develop WebAPI. 2) In game development, C# is combined with the Unity engine to realize role control and other functions. 3) C# supports polymorphism and asynchronous programming to improve code flexibility and application performance.
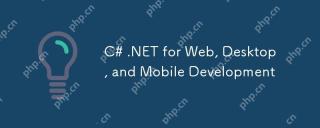
C# and .NET are suitable for web, desktop and mobile development. 1) In web development, ASP.NETCore supports cross-platform development. 2) Desktop development uses WPF and WinForms, which are suitable for different needs. 3) Mobile development realizes cross-platform applications through Xamarin.
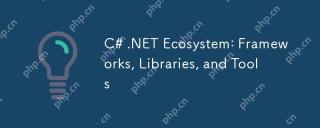
The C#.NET ecosystem provides rich frameworks and libraries to help developers build applications efficiently. 1.ASP.NETCore is used to build high-performance web applications, 2.EntityFrameworkCore is used for database operations. By understanding the use and best practices of these tools, developers can improve the quality and performance of their applications.

How to deploy a C# .NET app to Azure or AWS? The answer is to use AzureAppService and AWSElasticBeanstalk. 1. On Azure, automate deployment using AzureAppService and AzurePipelines. 2. On AWS, use Amazon ElasticBeanstalk and AWSLambda to implement deployment and serverless compute.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
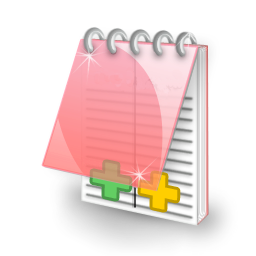
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SublimeText3 Linux new version
SublimeText3 Linux latest version
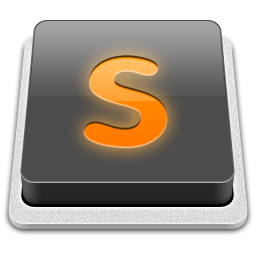
SublimeText3 Mac version
God-level code editing software (SublimeText3)

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
