The terms in an expression can be grouped using precedence of operators affecting the expression evaluation where the precedence of certain operators are high compared to the precedence of other operators and while grouping the operators, the operators with higher precedence is given first priority when compared to the operators with lower precedence for the evaluation of expressions and different types of operators are postfix operator, unary operator, multiplicative operator, additive operator, shift operator, relational operator, equality operator, Bitwise AND operator, Bitwise XOR operator, Bitwise OR operator, Logical AND operator, logical OR operator, conditional operator, assignment operator and comma operator.
Functions of Operator Precedence in C#
In order to understand the working of operator precedence in C#, we need to know the order of precedence of operators. The order of precedence in the higher order is as listed below:
1. Postfix Operator
- This include the operators ()[]->.++–
- The associativity for these operators is from left to right whenever they are used in an expression and considered for evaluation.
2. Unary Operator
- This include the operators + – ! ~ ++ — (type)* &sizeof
- The associativity for these operators is from right to left whenever they are used in an expression and considered for evaluation.
3. Multiplicative Operator
- This include the operators */%
- The associativity for these operators is from left to right whenever they are used in an expression and considered for evaluation.
4. Additive Operator
- This include the operators +-
- The associativity for these operators is from left to right whenever they are used in an expression and considered for evaluation.
5. Shift Operator
- This include the operators <<>>
- The associativity for these operators is from left to right whenever they are used in an expression and considered for evaluation.
6. Relational Operator
- This include the operators <<=>>=
- The associativity for these operators is from left to right whenever they are used in an expression and considered for evaluation.
7. Equality Operator
- This include the operators == !=
- The associativity for these operators is from left to right whenever they are used in an expression and considered for evaluation.
8. Bitwise AND Operator
- This includes the operator &
- The associativity for this operator is from left to right.
9. Bitwise XOR Operator
- This includes the operator ^
- The associativity for this operator is from left to right.
10. Bitwise OR Operator
- This includes the operator |
- The associativity for this operator is from left to right.
11. Logical AND Operator
- This includes the operator &&
- The associativity for these operators is from left to right whenever they are used in an expression and considered for evaluation.
12. Logical OR Operator
- This includes the operator ||
- The associativity for these operators is from left to right whenever they are used in an expression and considered for evaluation.
13. Conditional Operator
- This includes the operator ?:
- The associativity for these operators is from right to left whenever they are used in an expression and considered for evaluation.
14. Assignment Operator
- This include the operators= += -= *= /= %= >>= <<= &= ^= |=
- The associativity for these operators is from right to left whenever they are used in an expression and considered for evaluation.
15. Comma Operator
- This includes the operator ,
- The associativity for this operator is from left to right.
Now that we know the order of precedence of operators taken into consideration whileevaluating an expression, let us understand the working of precedence operators through an example. Consider the below statement:
int y = 2 + 3 * 5;
What is the value of y after the execution of this statement?
- In the above statement, the operators + and * are in association with 3. As we have understood the precedence of operators and * having higher precedence than +, the expression 3 * 5 will be executed first and then the result of the expression is added to 2. Hence the value of y in the above statement will be 17.
- If + had higher precedence than *, then the expression 2 + 3 would have got executed first and the resulting expression would be multiplied with 5. Then the value of y after executing the statement would be 25. Since we are going by the order of precedence for operators in C#, * has higher precedence over + and the result of the expression is 17.
Example of Operator Precedence in C#
C# program to demonstrate the precedence of operators.
Code:
using System;
namespace Op
{
public class OpPrec
{
public static void Main(string[] args)
{
int res;
int x = 4, y = 5, z = 3;
res = --x * y - ++z;
Console.WriteLine(res);
bool res1;
res1 = y >= z + x;
Console.WriteLine(res1);
}
}
}
In the above program, in the expression –x * y – ++z, –x and ++z is evaluated first and then the resulting value of –x is multiplied with y and the resulting value is subtracted from the resulting value of ++z as per the operator precedence in c#. And in the expression y >= z + x, z+x is evaluated first and the resulting value is compared with the value of y as per the operator precedence in c#.
Output:
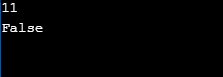
Conclusion
In this article, we have learnt the precedence of operators in C#, their order of precedence, working of operator precedence through definition and examples.
The above is the detailed content of Operator Precedence in C#. For more information, please follow other related articles on the PHP Chinese website!