Ejb is denoted as Enterprise Java Bean Component, also called server-side software components; it will mainly be used as the applications’ business logic. The web containers used for runtime environments include the software components, computer security, servlet lifecycle management, transaction processing, and web services.EJb is the architecture style written in the Java programming languages running on the server-side of the computer network, so it has followed the client-server model in the applications. And also, ejb is the java bean technology to distribute the web components on the client-side it has the feature called reusable components in multiple web applications.
Start Your Free Software Development Course
Web development, programming languages, Software testing & others
Syntax:
The basic syntax of the EJB model in java programming as follows.
import javax.ejb.*; import java packages; class classname { Main method() { -----Some programming logics---- } }
The above codes are basic steps for developing the application using ejb packages.
How to use EJB in Java Works?
- In EJB, the java bean class will use more than one annotation for the ejb specifications, which will be helpful for satisfying the user requirements based on the business purpose.EJB has a different set of versions and has followed the feature like JNDI(java naming directory interface). One of the resources in servers jndi is the directory service is used for locating or allocating the resource, like ejb, data source, and JMS queue services. The servers have a default scheme name for the jndi resources, but it should be overridden if we changed the configuration part’s name.
- EJB will work on java beans; it has two different types 1. Session beans and 2.Message Driven BeansSession beans. Session beans will be used for client-server interactions; it encapsulates the application’s business logic through programmatically by the client invocation will be done by either local machine or remote machine bean will be used by the client with the help of web services. Session beans will be three different categories 1. Stateless,2.Stateful and 3.Singleton.Stateless beans when the client use these type of beans there is no permanent state in web containers, so they are thread-safe performance wise very fast when compared to stateful beans. These beans are shared with multiple clients at the same time.
- Stateful beans can store the states in containers; once the client terminates the session, these states are also destroyed in the server. Singelton beans it has a single instance session for the entire lifecycle of that application; these beans also shared the states with multiple clients. These beans are thread-safe, so developers will use them more easily, and performance also fast compared to stateful beans.
- Message Driven Beans(MDB) is the type of bean that is used as the type of message asynchronous like JMS message listeners, but it will receive the message response as JMS messages instead of the events. We can map the messages using jndi services whenever the message receives the container calls the MDB beans using the onMessage() method for further process. The whole process of the MDB using the onMessage() method will be a single transaction. If suppose message process is a rollback, the receiver message again redelivered.
Examples to Implement EJB in Java
Below are the examples mentioned:
Example #1
Interface:
package JPAEJB; import java.util.List; import javax.ejb.Remote; @Remote public interface CustomerInterface { void addBooks(String name); List getBooks(); }
Implementation:
import java.util.ArrayList; import java.util.List; import javax.ejb.Stateless; import JPAEJB.CustomerInterface; @Stateless public class CustomerClass implements CustomerInterface { List<string>books; public CustomerClass() { books = new ArrayList<string>(); } public void addBooks(String name) { books.add(name); } public List<string> getBooks() { return books; } }</string></string></string>
CustomerInterface:
import JPAEJB.CustomerInterface; import java.io.BufferedReader; import java.io.FileInputStream; import java.io.IOException; import java.io.InputStreamReader; import java.util.List; import java.util.Properties; import javax.naming.InitialContext; import javax.naming.NamingException; public class CustomerInterfaceImplem { BufferedReader brConsoleReader = null; Properties p; InitialContext c; { p = new Properties(); try { p.load(new FileInputStream("jndi.properties")); } catch (IOException ex) { ex.printStackTrace(); } try { c = new InitialContext(p); } catch (NamingException ex) { ex.printStackTrace(); } brConsoleReader = new BufferedReader(new InputStreamReader(System.in)); } public static void main(String[] args) { CustomerInterfaceImplem cust = new CustomerInterfaceImplem(); cust.testStatelessEjb(); } private void show() { System.out.println("Welcome to my domain"); System.out.print("Options \n1. Add the Books\n2. Exit \nEnter your Choice: "); } private void StatelessEjb() { try { intc = 1; CustomerInterface cust = (CustomerInterface)ctx.lookup("CustomerInterface/remote"); while (c != 2) { String books; show(); String str = brConsoleReader.readLine(); c = Integer.parseInt(str); if (c == 1) { books = brConsoleReader.readLine(); cust.addBooks(books); }elseif (c == 2) { break; } } List<string>books1 = cust.getBooks(); System.out.println(books1.size()); for (inti = 0; i<books1.size system.out.println books1.get customerinterface cust1='(CustomerInterface)ctx.lookup("cust/remote");' list>books2 = cust.getBooks(); System.out.println(books2.size()); for (inti = 0; i<books2.size system.out.println books2.get catch e e.printstacktrace finally try if brconsolereader.close ex> <p><strong>Sample Output:</strong></p> <p><img src="/static/imghwm/default1.png" data-src="https://img.php.cn/upload/article/000/000/000/172500613249868.png?x-oss-process=image/resize,p_40" class="lazy" alt="EJB in Java" ></p> <h4 id="Example">Example #2</h4> <p><strong>EJB in Web Services:</strong></p> <pre class="brush:php;toolbar:false">package JPAEJB; import java.util.List; import Customers.CustomerInterfaceImplem; public class Ejbclients{ public static void main(String[] args) { for(CustomerInterface cust:getBooks()) { System.out.println(cust.getBooks()); } } private static List <customerinterface> getBooks() { CustomerInterfaceImplem s = new CustomerInterfaceImplem(); CustomerInterface c = s.getCustomerInterfacePort(); return c.getBooks(); } }</customerinterface>
Example #3
EJB Security:
import javax.ejb.* @Stateless @DeclareRoles({"customer""books"}) public class CustSecurity implements CustomerInterface { @RolesAllowed({"books"}) public void delete(CustomerInterface cust) { System.out.println("customer delete the books"); } @PermitAll public void showBooks(CustomerInterface cust) { System.out.println("customer viewed the books"); } @DenyAll public void deleteAll() { System.out.println("customer delete all the books in libraries"); } }
Security XML:
<?xml version="1.0"encoding="UTF-8"?> <ejb-jar> <security-role-mapping> <role-name>customer</role-name> <group-name>customer-groups</group-name> </security-role-mapping> <security-role-mapping> <role-name>Books</role-name> <group-name>Books-group</group-name> </security-role-mapping> <enterprise-beans></enterprise-beans> </ejb-jar>
Explanation: The above three examples are the same output we used different features of the ejb first example we use the customer will add and delete the books using jndi(java naming directory interface) second example we use the web service for customer done the operations through web final example we used the additional security.
Conclusion
Generally, EJB act as an interface outside of business logic applications; it has the browser compatibility feature security-wise more when compared to other business logic frameworks. It also maintains the system-level transactions.
The above is the detailed content of EJB in Java. For more information, please follow other related articles on the PHP Chinese website!

The article discusses using Maven and Gradle for Java project management, build automation, and dependency resolution, comparing their approaches and optimization strategies.
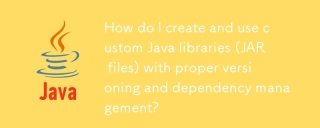
The article discusses creating and using custom Java libraries (JAR files) with proper versioning and dependency management, using tools like Maven and Gradle.
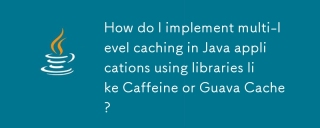
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra

The article discusses using JPA for object-relational mapping with advanced features like caching and lazy loading. It covers setup, entity mapping, and best practices for optimizing performance while highlighting potential pitfalls.[159 characters]
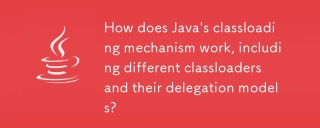
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
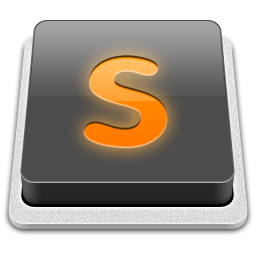
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use