Java Arithmetic Exception is a kind of unchecked error or unusual outcome of code that is thrown when wrong arithmetic or mathematical operation occurs in code at run time. A runtime problem, also known as an exception, occurs when the denominator is integer 0, the JVM is unable to evaluate the result, and therefore the execution of the program is terminated, and an exception is raised. The point at which exception has raised the program terminates but code earlier to that is executed, and the result is shown.
The base class of java arithmetic exception is lang.ArithmeticException, which comes under java.lang.RuntimeException.
ADVERTISEMENT Popular Course in this category JAVA MASTERY - Specialization | 78 Course Series | 15 Mock TestsStart Your Free Software Development Course
Web development, programming languages, Software testing & others
Structure of ArithmeticException in Java
Structure of base Class ArithmeticException:
Constructor of ArithmeticException
1. ArithmeticException(): Define an Arithmetic Exception with no parameter passed or with not any detailed message.
2. ArithmeticException(String s): Define an ArithmeticException with one parameter passed.
s: s is the detailed message
How ArithmeticException Work in Java?
Below are the two situations that can lead to Java ArithmeticException:
- Division of a number by Zero which is not defined and an integer.
- Non-terminating long decimal numbers byBig Decimal.
1. Division of a Number by an Integer Zero
An arithmetic exception in java is thrown when we try to divide a number by zero. Below is the java code to illustrate the operation:
Example #1
Code:
package com.java.exception; public class ArithmeticException { void division(int a,int b) { int c=a/b; System.out.println("Division has been successfully done"); System.out.println("Value after division: "+c); } public static void main(String[] args) { ArithmeticException ex=new ArithmeticException(); ex.division(10,0); } }
Output:
- lang.ArithmeticException: Exception thrown by java language during division
- / by zero: It is the detailed message given to the class ArithmeticException while generating the ArithmeticException instance.
As we have divided 10 by 0, where 0 is an integer and is undefined, it throws above arithmetic exception.
Example #2
Code:
//package com.java.exception; public class ArithmeticException { void division(int a,int b) { int c=a/b; System.out.println("Division of a number is successful"); System.out.println("Output of division: "+c); } public static void main(String[] args) { ArithmeticException ex=new ArithmeticException(); ex.division(10,5); } }
Output:
2. Non-Terminating Long Decimal Numbers by Big Decimal
Java has a BigDecimal class that represents decimal numbers up to a large number of precision digits. This class of java also has some set of functionalities that are not available in the primitive data types, for example, integer, doubles, and floats. These functionalities provide rounding off the decimal numbers
Below is the code for illustration:
Example #1
Code:
//package com.java.exception; import java.math.BigDecimal; public class ArithmeticException { public static void main(String[] args) { BigDecimal a=new BigDecimal(1); BigDecimal b=new BigDecimal(6); a=a.divide(b); System.out.println(a.toString()); } }
Output:
In the java code written above, as the big decimal class doesn’t know what to be done with the division output, hence it throws or shows an arithmetic exception in the output console.
It throws an exception with a detailed message “Non-terminating decimal expansion, no exact representation.”
One possible way out for the above big decimal class is to state the number of decimal places we need from a big decimal number and then limit the value to a definite number of decimals. For example, c should be limited to 7 decimal places by rounding the number upto the 7th decimal precision value.
Example #2
Code:
//package co.java.exception; import java.math.BigDecimal; public class ArithmeticException { public static void main(String[] args) { BigDecimal a=new BigDecimal(1); BigDecimal b=new BigDecimal(6); a=a.divide(b,7,BigDecimal.ROUND_DOWN);// limit of decimal place System.out.println(a.toString()); } }
Output:
The output console shows the result as a number with a 7th decimal point value, which means rounding works fine.
How to Avoid or Handle Java ArithmeticException?
Handling the exception thrown by java virtual machine is known as exception handling. The advantage of exception handling is the execution of the code is not stopped.
An exception is handled by using a combination of try and catch. A try/catch block is placed in the code that might generate an exception. Code written inside a try/catch block is referred to as a protected code.
Syntax:
try { set of statements//protected code } catch (exceptionname except) { // Catch set of statements---can contain single catch or multiple. }
ArithmeticException Handling using try & Catch Blocks
- Write the statements that can throw ArithmeticException with try and catch blocks.
- When an exception occurs, the execution falls to the catch block from an exception’s point of occurrence. It executes the catch block statement and continues with the statement present after the try and catch blocks. Below is the example:
Code:
public class ExceptionHandled { public static void main(String args[]) { int x =100, y = 0; int z; System.out.println("Hello world"); try { z = x/y; System.out.println(z); } catch(ArithmeticException except) { System.out.println("Avoid dividing by integer 0" + except ); } System.out.println("Hello class"); System.out.println("Hello there"); } }
Output:
Hello class and Hello, there are also printed on the output console apart from Hello world. This is the outcome of the exception handling mechanism.
Explanation:
- Try, and catchblocks are exception handling keywords in Java used completely used to handle the exception or unchecked error raised in the code without halting the execution of the code.
- Detect the trouble creating statements and place them in the try block. When the try block throws the exception, the catch block handles that exception, and the execution of the program goes further to the last statement.
- If the try block does not throw the exception, the catch block is simply overlooked.
- Run a suitable exception handler or statements in the catch block to handle or detect the exception thrown by the try block successfully.
Conclusion
This article has learned about java arithmetic exception and how to handle the exception under try and catch block. An arithmetic exception occurs most of the time because of the division of a number by integer 0. In this article, I have used a single catch for a single try, but we can also use multiple catch for a single try.
The above is the detailed content of Java ArithmeticException. For more information, please follow other related articles on the PHP Chinese website!

The article discusses using Maven and Gradle for Java project management, build automation, and dependency resolution, comparing their approaches and optimization strategies.
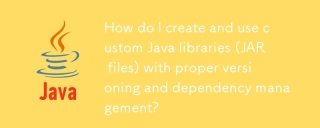
The article discusses creating and using custom Java libraries (JAR files) with proper versioning and dependency management, using tools like Maven and Gradle.
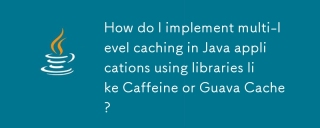
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra

The article discusses using JPA for object-relational mapping with advanced features like caching and lazy loading. It covers setup, entity mapping, and best practices for optimizing performance while highlighting potential pitfalls.[159 characters]
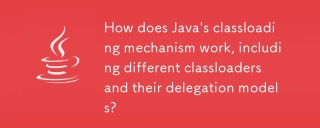
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

Notepad++7.3.1
Easy-to-use and free code editor

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool