File class in Java plays a very important role in saving the application’s paths and managing various files in a directory format. Also, this method is used to manage and version the paths in an order or hierarchy that is too abstract. Naming any file with any string is not enough for mentioning the path for applications. Running applications in different platforms and trying to bind and compile themselves with one or more attributes. File class consists of various methods, which gives java flexibility to create the file, renaming a file and deleting a file.
Start Your Free Software Development Course
Web development, programming languages, Software testing & others
Syntax and Parameters:
File abslt = new File("https://cdn.educba.com/usr/local/bin/at.txt");
The syntax flow works in a way that it starts with:
-
File: It represents that the file is taken into consideration for performing the manipulations.
-
abslt: It represents the file name which can be anything relevant to the file, i.e. containing some content or value.
-
new File(): Creation of the object related to file or can be said as file object that can contain relevant information about the file values and retrieve the values later.
-
(“/usr/local/bin/at.txt”): parameter passed is an absolute path that is recommended. It is not very much a recommended method to pass a relative path as a parameter.
How does File Class work in Java?
A File Class in Java works in a way where the File in a class will have some content that can be needed later. They can be retrieved and modified later with the help of a file object. Since these files are maintained in a hierarchy with different names and path names, it is not enough and recommended to use only string as a name. it Is an abstract representation of the maintained files and directory path names.
A pathname can be absolute or relative depending in the user specification. If it is absolute, then it is quite a recommended method as it will help in the mere future at the time of retrieval to get the designated file mentioned in the absolute path. Therefore, the actual working starts first by creating a file class and then the respective object is created using which the filename and directory name is passed. A file system sometimes prohibits accessibility or provides a restriction at the time of object accessibility which is caused due to the constraints already present over the actual file system object. This creates hinderance for the operations like reading, writing and executing. As the instances created by the file class is immutable hence once created, they cannot change the pathname, and the representation of the file object will also not get changed.
Constructors
There are constructors which are passed and are frequently used by the File class of Java :
-
File(File parent, String child): The file parent parameter is responsible for invoking abstract pathname from the file object created, and the String child parameter is responsible for invoking the child pathname string from the file object created as a new instance of a file.
-
File(URI uri): A new instance of the file gets created when the URI for the given file gets converted into an abstract pathname.
-
File(String parent, String child): A new instance of the file gets created from the parent pathname, and the child pathname string passed as a parameter to the file.
-
File(String pathname): A new instance of the file gets created as soon as the string of pathname gets converted into an abstract pathname.
Methods
Below are the methods:
-
boolean createNewFile(): The abstract pathname passed as an argument to the method is responsible for creating a new empty file.
-
int compareTo(File pathname): When any two pathnames are arranged in lexical order, this method is used to compare both the files and then create a new instance of the file.
-
boolean canExecute(): The abstract pathname parameter passed as an argument is responsible for testing whether the application can execute and function properly.
-
boolean canRead(): The abstract pathname parameter passed as an argument is responsible for testing whether the application can read the passed file.
-
boolean canWrite(): The abstract pathname parameter passed as an argument is responsible for testing whether the application can write some content on the passed file.
-
String getAbsolutePath(): The abstract pathname passed as a parameter for creating an instance returns the abstract pathname string.
-
boolean exists(): This method is responsible for verifying whether the abstract pathname exists.
-
boolean delete(): This method deletes the file or the directory having the contents which is pointed with this abstract pathname.
-
static File createTempFile(String prefix, String suffix): This method is used for creating an empty temporary file or directory by default.
-
boolean equals(Object obj): The file object created as part of the abstract pathname is responsible for verifying the equality with the given object.
-
String[] list(): Files present in the directory or file system gets returned in the form of an array as strings.
-
long length(): The abstract pathname denoted by the file object is responsible for getting the length of the file.
-
boolean isHidden(): This method is responsible for testing whether the abstract pathname names the file is a hidden file.
-
boolean isFile(): This method is responsible for testing whether the file returned is a normal file or is denoted by the abstract pathname object.
-
boolean isDirectory(): This method is responsible for testing whether the file denoted by this abstract pathname is a directory or not.
-
boolean mkdir(): This method is responsible for creating the directory named by passing the abstract pathname.
-
File[] listFiles(): All the files present in the directory is responsible for returning an array of abstract pathnames as denoted by the object.
-
boolean setReadable(boolean readable): It is used for setting the owner’s or user’s permission for reading the content present in the file.
-
boolean renameTo(File dest): The file represented by the abstract pathname is responsible for the renaming of the file.
-
boolean setExecutable(boolean executable): This method is used for setting the owner’s execution permission.
-
boolean setReadable(boolean readable, boolean ownerOnly): This method is responsible for setting up the constraint owner’s readability permission.
-
URI toURI(): Representation of this abstract pathname is done by constructing the URI file.
-
String toString(): This method is responsible for returning the string pathname of the abstract pathname.
-
boolean setReadOnly(): This method is used only to perform the read operations exclusively.
-
boolean setWritable(boolean writable): This method is used for setting up the write permission for the owner even at the time of execution.
Examples
Now we will learn about the Java File and its various operations with the help of examples.
Example #1
This program is used for demonstrating the creation of a new file and return the file is already present if it exists already.
Code:
import java.io.File;
import java.io.IOException;
public class FilePropTest {
public static void main(String[] args) {
try {
File file = new File("java_File.txt");
if (file.createNewFile()) {
System.out.println("Created a new file");
} else {
System.out.println("File is already present ");
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
Output:
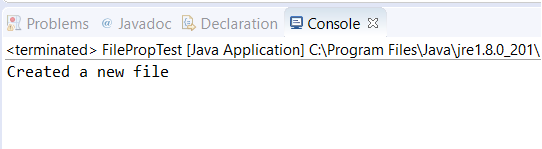
Example #2
This program is used to demonstrate the filenames being passed as an absolute pathname and retrieving all the filenames within the directory of the drive.
Code:
import java.io.File;
public class FilePropTest2 {
public static void main(String[] args) {
File fil_nm=new File("C:/Users/adutta/documents");
String all_file_names[]=fil_nm.list();
for(String enlist_filename:all_file_names){
System.out.println(enlist_filename);
}
}
}
Output:
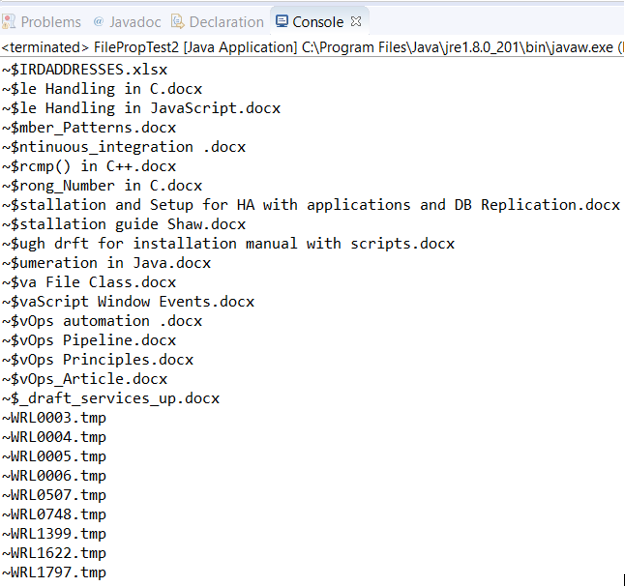
Note: All the methods explained and defined can be demonstrated in this way and can be performed by just calling the method name already present in Java’s file class.
Conclusion – Java File Class
File class in Java is a very useful class as it do not include any of the external specifications but includes methods that are present as part of the file package and can be used seamlessly for performing any of the file related activities, including getting the name of the files and retrieving the files respectively. Thus, the File class has provided a lot of flexibility to the methods and all other files.
The above is the detailed content of Java File Class. For more information, please follow other related articles on the PHP Chinese website!