Java is a popular programming language used to produce software programs and applications and was developed in the mid-’90s. Java is compatible with all modern-day operating systems. Its popularity is due to certain provisions it provides to ensure application-wide security and reusability, like the static member. In Java, the static keyword is primarily used to ensure efficient system memory management and reusability of common properties. We can use the static keyword with variables, methods, nested classes, as well as code blocks. Static is used as a non-access modifier in Java. The Static keyword indicates that a particular member belongs to a type itself instead of individual instances of that particular type. This implies that only a single instance of that static member can be created and shared among all instances of the class.
Start Your Free Software Development Course
Web development, programming languages, Software testing & others
Create a Static Method
To declare a static member, whether it is a block, variable, method, or nested class, we need to precede the member with the keyword static. When we declare a member as static, we can access it before any of its class objects are created since it is independent of its class objects.
In the example below, we will create a static method and see how it can be called without any reference to its class:
Code:
class Main // declaring a class name called staticTest { // Declaring a static method below public static void myStaticmethod() { System.out.println("Hello, I was called from static method."); } public static void main(String[] args ) { // Calling the static method without any object reference myStaticmethod(); } }
Output:
Create a Single Class with Multiple Static Blocks
Sometimes we need to perform certain computations to initialize our static variables. In such cases, we can declare a static block that is loaded only once when the class is first executed.
Example:
Code:
class Main { // Declaring a static variable below static int a = 10; static int b; // Declaring a static block below static { System.out.println( " In static block now. " ); b = a*2; } public static void main( String[] args ) { System.out.println(" Value of b : " + b); } }
Output:
Now moving to static variables, they are primarily global variables since when a variable is declared as static, only a single copy of the variable is created and shared among all objects in a class. We would need to consider that we can create a static variable only at a class level. If a program has both static variables as well as a static block, then the static variable and static block will be executed in the order in which they are written in the program.
A method is Java; when declared with a static keyword preceding, it is called a static method. The most commonly used static method in Java is the main method. Methods in Java declared with static keywords are also accompanied by several restrictions.
Few of which are listed below:
- Static methods are permitted only to call other static methods.
- Static methods can only access static data directly.
- Static methods cannot make reference to this or super under any circumstances.
Why is the Main Method Static in Java?
- The main method in Java is static since we do not require an object to be able to call the main method.
- If it were a non-static method, then we would first need JVM (Java Virtual Machine) to call the class object, and this would not be a memory-efficient process since it would require additional memory to do so.
Use Cases of Static Member
You might be thinking about what circumstances and scenarios should we use static members and variables?
We can use a static variable for any property that would be common to all objects. For example, if we had a class by the name Student, then all student objects would share the same property of college name, which can be declared as a static variable in that case. We can also use static variables when their value is independent of their class objects.
Example:
Code:
class Student { int roll_no; // declaring an instance variable String name; static String college_name ="KC College"; // declaring a static variable for common property college name //declaring the class constructor below Student(int r, String n) { roll_no = r; name = n; } // declaring a method to display the values void display () { System.out.println(roll_no+" "+name+" "+college_name); } } //Declaring a test class to display the values of objects public class Main{ public static void main(String args[]){ Student st1 = new Student(111,"Karan"); Student st2 = new Student(222,"Arjun"); //below we have changed the college name which was a common property of all objects with a single line of code Student.college_name ="Jain Hind College"; st1.display(); st2.display(); } }
Output:
Static Nested Classes in Java
- In Java, we cannot declare the top-level class as static, but we can declare its nested classes as static if need be. Such classes are called nested static classes.
- Static methods in Java are identified at runtime, and since over-riding is a part of runtime polymorphism, static methods cannot be overridden. We cannot declare abstract methods as static.
Conclusion – Static Keyword in Java
With the above examples and explanation, we can conclude that Java provides several features and properties like static and abstract, which make it secure, safe, and robust for usage. These leading to Java are still a very popular choice for developing systems where security is the primary concern.
The above is the detailed content of Static Keyword in Java. For more information, please follow other related articles on the PHP Chinese website!
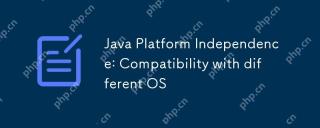
JavaachievesplatformindependencethroughtheJavaVirtualMachine(JVM),allowingcodetorunondifferentoperatingsystemswithoutmodification.TheJVMcompilesJavacodeintoplatform-independentbytecode,whichittheninterpretsandexecutesonthespecificOS,abstractingawayOS
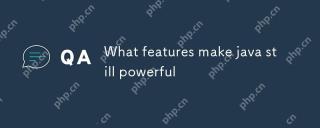
Javaispowerfulduetoitsplatformindependence,object-orientednature,richstandardlibrary,performancecapabilities,andstrongsecurityfeatures.1)PlatformindependenceallowsapplicationstorunonanydevicesupportingJava.2)Object-orientedprogrammingpromotesmodulara
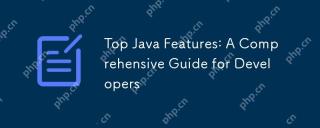
The top Java functions include: 1) object-oriented programming, supporting polymorphism, improving code flexibility and maintainability; 2) exception handling mechanism, improving code robustness through try-catch-finally blocks; 3) garbage collection, simplifying memory management; 4) generics, enhancing type safety; 5) ambda expressions and functional programming to make the code more concise and expressive; 6) rich standard libraries, providing optimized data structures and algorithms.
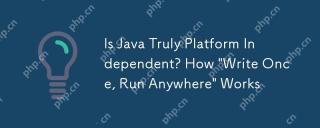
JavaisnotentirelyplatformindependentduetoJVMvariationsandnativecodeintegration,butitlargelyupholdsitsWORApromise.1)JavacompilestobytecoderunbytheJVM,allowingcross-platformexecution.2)However,eachplatformrequiresaspecificJVM,anddifferencesinJVMimpleme
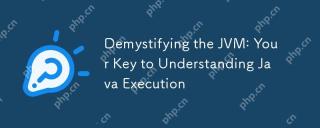
TheJavaVirtualMachine(JVM)isanabstractcomputingmachinecrucialforJavaexecutionasitrunsJavabytecode,enablingthe"writeonce,runanywhere"capability.TheJVM'skeycomponentsinclude:1)ClassLoader,whichloads,links,andinitializesclasses;2)RuntimeDataAr
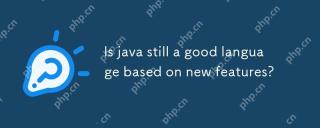
Javaremainsagoodlanguageduetoitscontinuousevolutionandrobustecosystem.1)Lambdaexpressionsenhancecodereadabilityandenablefunctionalprogramming.2)Streamsallowforefficientdataprocessing,particularlywithlargedatasets.3)ThemodularsystemintroducedinJava9im
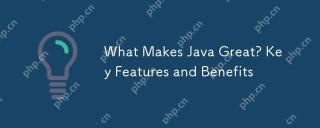
Javaisgreatduetoitsplatformindependence,robustOOPsupport,extensivelibraries,andstrongcommunity.1)PlatformindependenceviaJVMallowscodetorunonvariousplatforms.2)OOPfeatureslikeencapsulation,inheritance,andpolymorphismenablemodularandscalablecode.3)Rich
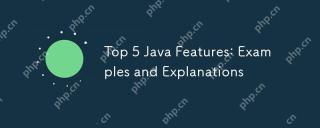
The five major features of Java are polymorphism, Lambda expressions, StreamsAPI, generics and exception handling. 1. Polymorphism allows objects of different classes to be used as objects of common base classes. 2. Lambda expressions make the code more concise, especially suitable for handling collections and streams. 3.StreamsAPI efficiently processes large data sets and supports declarative operations. 4. Generics provide type safety and reusability, and type errors are caught during compilation. 5. Exception handling helps handle errors elegantly and write reliable software.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor
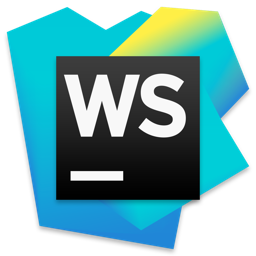
WebStorm Mac version
Useful JavaScript development tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
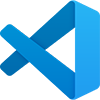
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
